In Roblox Studio, functions are essential programming constructs that allow you to encapsulate a block of code and give it a name. They provide a way to organize and reuse code, making it easier to maintain and enhance your Roblox games and experiences. Functions can be defined to perform specific tasks or operations and can accept input parameters and return values.
Here are the key aspects of understanding functions in Roblox Studio:
Function Definition:
- Functions are defined using the
function
keyword followed by the function name and a set of parentheses()
. - The code block for the function is enclosed in a pair of curly braces
{}
. - Here’s an example of a basic function definition:
function sayHello()
print("Hello, world!")
end
Function Invocation:
- To execute a function and run its code, you need to invoke or call the function by using its name followed by a pair of parentheses
()
. - Here’s an example of invoking the
sayHello()
function defined above:
sayHello() -- Output: Hello, world!
Parameters:
- Functions can have input parameters that allow you to pass values or data to the function for processing.
- Parameters are defined within the parentheses of the function definition.
- You can use these parameters as variables within the function’s code block.
- Here’s an example of a function with parameters:
function greetPlayer(playerName)
print("Welcome, " .. playerName .. "!")
end
Return Values:
- Functions can also return values back to the caller.
- The
return
keyword is used within a function to specify the value to be returned. - Here’s an example of a function that returns a value:
function addNumbers(a, b)
return a + b
end
local result = addNumbers(5, 3)
print(result) -- Output: 8
- Reusability and Modularity:
- Functions promote code reusability and modularity by allowing you to define a block of code that can be called multiple times from different parts of your program.
- You can organize your code into smaller, more manageable functions, making it easier to understand, debug, and maintain.
By understanding functions in Roblox Studio, you gain the ability to create modular and reusable code, making your game development process more efficient and flexible. Functions help you structure and organize your code logic, enable parameter passing for dynamic behavior, and support returning values for data processing and flow control.
Exploring the purpose and benefits of using parameters in functions
In Roblox Studio, parameters in functions serve an important role in defining flexible and reusable code. Parameters allow you to pass values or data to functions when they are called, enabling dynamic behavior and customization. Here are the purposes and benefits of using parameters in functions:
- Customization and Flexibility:
- Parameters provide a way to customize the behavior of a function by allowing you to pass specific values or data to it.
- By accepting parameters, functions can be used in different contexts with varying inputs, making them versatile and adaptable.
- Parameters allow you to create generic functions that can be reused with different data, enhancing code flexibility.
- Encapsulation and Modularity:
- Using parameters allows you to encapsulate related code within a function, promoting modularity.
- Functions with well-defined parameters encapsulate specific functionality, making the code easier to understand, maintain, and debug.
- By breaking down complex operations into smaller, parameterized functions, you can improve code organization and readability.
- Reusability:
- Parameters enable the reusability of functions. By defining functions with parameters, you can create blocks of code that can be called and reused in multiple parts of your program.
- Instead of duplicating code, you can simply invoke a function with different parameter values to achieve specific outcomes.
- This promotes code efficiency, reduces redundancy, and simplifies maintenance.
- Dynamic Behavior:
- Parameters allow functions to handle different inputs, resulting in dynamic behavior.
- By accepting different parameter values, functions can perform calculations, transformations, or actions based on the provided data.
- This flexibility allows functions to adapt to changing circumstances and produce varying outputs based on the inputs they receive.
- Interoperability:
- Parameters facilitate the communication between different parts of your program.
- Functions can accept parameters and return values, allowing for seamless data exchange between functions and the rest of your code.
- This promotes code integration and collaboration, as functions can rely on and interact with each other through parameter passing.
In summary, using parameters in functions in Roblox Studio enhances customization, flexibility, modularity, reusability, and dynamic behavior. By defining functions with parameters, you can create modular, versatile code that can be customized and reused in various contexts. Parameters enable efficient code organization, promote code sharing, and facilitate data exchange between different parts of your program.
Syntax of declaring a function with parameters
In Roblox Studio, declaring a function with parameters involves specifying the parameter names within the parentheses of the function definition. The syntax for declaring a function with parameters in Roblox Studio is as follows:
function functionName(parameter1, parameter2, ...)
-- Function code block
-- Access parameters using their names
-- Perform operations using the passed values
-- Return a value if necessary
end
Let’s break down the syntax:
- The
function
keyword is used to declare a function. functionName
is the name you choose for your function. Replace it with a meaningful name that describes the purpose of the function.- Inside the parentheses
()
, you specify the parameters separated by commas. You can have one or more parameters. - Each parameter is declared with a name, such as
parameter1
,parameter2
, and so on. You can name the parameters as per your preference, but choose descriptive names that convey their purpose. - Within the function’s code block, you can access the parameter values using their names, just like variables.
- Perform operations or calculations using the passed parameter values.
- If required, you can return a value from the function using the
return
keyword.
Here’s an example of a function with parameters:
function calculateSum(a, b)
local sum = a + b
return sum
end
In the above example, the function calculateSum
takes two parameters a
and b
. It calculates their sum and returns the result.
To invoke or call a function with parameters, you provide the corresponding values when invoking it:
local result = calculateSum(5, 3)
print(result) -- Output: 8
In this example, the calculateSum
function is called with the values 5
and 3
, which are assigned to the a
and b
parameters, respectively. The returned result is stored in the result
variable and printed.
Using the syntax for declaring functions with parameters in Roblox Studio, you can create flexible and customizable code that can accept input values and produce desired outputs based on those inputs.
Naming conventions for parameters
When it comes to naming conventions for parameters in Roblox Studio, it’s important to follow best practices to ensure code readability and maintainability. While there are no strict rules, adhering to commonly accepted conventions helps make your code more understandable to yourself and other developers. Here are some recommended naming conventions for parameters in Roblox Studio:
- Descriptive and Meaningful Names:
- Choose parameter names that clearly describe the purpose or meaning of the value being passed.
- Use names that accurately represent the data or functionality the parameter represents.
- For example, if the parameter represents a player’s name, you could use
playerName
as the parameter name.
- Camel Case or Underscore Separation:
- Use camel case or underscore separation to improve readability.
- Camel case: Start with a lowercase letter and capitalize the first letter of each subsequent word within the name (e.g.,
playerName
,maxHealth
). - Underscore separation: Separate words using underscores (e.g.,
player_name
,max_health
). - Choose a consistent naming style and stick to it throughout your codebase.
- Avoid Ambiguous or Generic Names:
- Avoid using generic names like
arg1
,param1
, or single-letter names (x
,y
) unless they are commonly used in specific contexts. - Use names that provide context and meaning to the parameter, making it clear what kind of data is expected.
- Avoid using generic names like
- Plural Names for Collections:
- If the parameter represents a collection or array of values, consider using a plural name to indicate that it is a group of elements.
- For example, if the parameter represents an array of players, you could name it
players
orplayerList
.
- Consistency with Existing Naming Conventions:
- If you are working with an existing codebase, try to follow the existing naming conventions to maintain consistency.
- Consistency in naming conventions makes it easier for developers to understand and navigate the code.
Here’s an example that incorporates these naming conventions:
function greetPlayer(playerName)
print("Welcome, " .. playerName .. "!")
end
local name = "John"
greetPlayer(name) -- Output: Welcome, John!
In the example above, the parameter name playerName
clearly indicates that the function expects a player’s name as input.
By following these naming conventions, you can make your code more readable, self-explanatory, and easier to understand and maintain. Consistency in naming parameters helps create clean and professional code that can be effectively shared and collaborated on within a team or community.
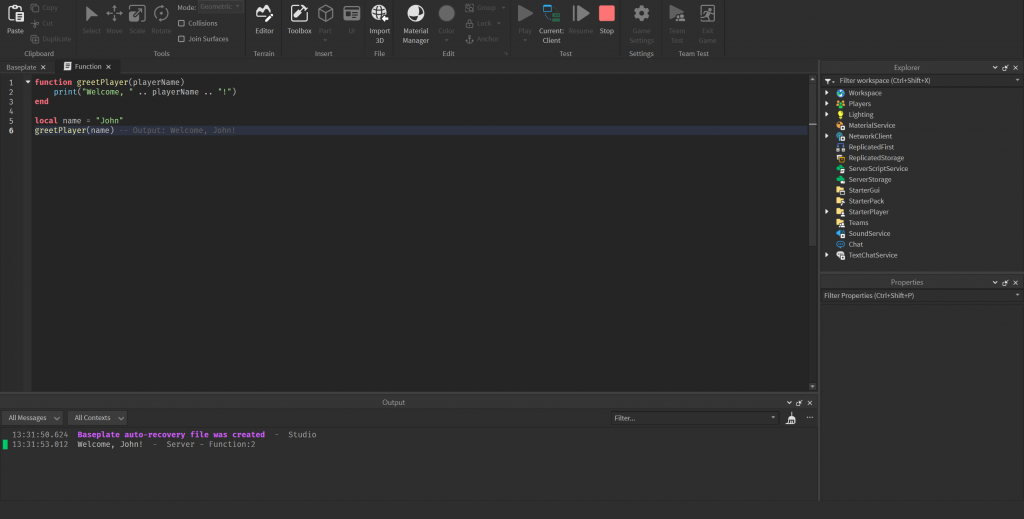
Defining the function body in roblox studio
In Roblox Studio, defining the function body refers to specifying the code that will be executed when the function is called. The function body contains the instructions and logic that define the behavior of the function. Here’s how you define the function body in Roblox Studio:
- Function Definition:
- Begin by declaring the function using the
function
keyword, followed by the function name and a pair of parentheses()
. - Example:
function functionName()
- Begin by declaring the function using the
- Code Block:
- After the parentheses, open a pair of curly braces
{}
to enclose the function body. - The code within these curly braces defines the behavior and operations of the function.
- Example:
- After the parentheses, open a pair of curly braces
function functionName()
-- Function body code goes here
-- You can include multiple statements and expressions
end
Function Logic:
- Inside the function body, you can write any valid Lua code to perform specific operations or tasks.
- This can include calculations, conditional statements, loops, function calls, variable declarations, and more.
- Example:
function greetPlayer(playerName)
print("Welcome, " .. playerName .. "!")
end
Parameters and Local Variables:
- If your function accepts parameters, you can use them within the function body like regular variables.
- Parameters allow you to pass values into the function for processing.
- Additionally, you can declare local variables within the function body using the
local
keyword. - Example:
function calculateSum(a, b)
local sum = a + b
return sum
end
Return Statements:
- If your function needs to return a value back to the caller, use the
return
statement. - The
return
statement specifies the value or values to be returned from the function. - Example:
function multiplyNumbers(a, b)
return a * b
end
By defining the function body in Roblox Studio, you specify the actions and operations that will be executed when the function is called. The code within the function body can access parameters, utilize local variables, perform calculations, make decisions, and return values. Defining clear and concise function bodies helps ensure that your code is well-structured, readable, and serves its intended purpose.
Understanding arguments and their relationship to parameters
In Roblox Studio, understanding the relationship between arguments and parameters is essential for working with functions effectively. Arguments and parameters play complementary roles in passing data to functions. Let’s explore their meaning and relationship:
- Parameters:
- Parameters are variables defined within the function’s parentheses during its declaration.
- They act as placeholders that receive values from the function’s caller.
- Parameters define the expected inputs for the function and allow it to operate on different data.
- Parameter names are used within the function body to reference the passed values.
- Arguments:
- Arguments are the actual values passed to a function when it is called or invoked.
- They correspond to the parameters of the function and provide the necessary data for the function to work with.
- Arguments can be literal values, variables, expressions, or the result of other function calls.
- The order and data types of the arguments should match the corresponding parameters of the function.
- Relationship between Arguments and Parameters:
- When a function is called, the arguments are provided in the same order as the parameters are defined in the function declaration.
- The values of the arguments are assigned to the corresponding parameters inside the function.
- This binding of arguments to parameters allows the function to access and utilize the passed data.
- The function can then perform operations using the parameter values, modify them, or return a result based on the provided arguments.
Here’s an example to illustrate the relationship between arguments and parameters:
function calculateSum(a, b)
local sum = a + b
return sum
end
local num1 = 5
local num2 = 3
local result = calculateSum(num1, num2)
print(result) -- Output: 8
In the above example, num1
and num2
are variables holding the values 5
and 3
, respectively. These values are passed as arguments when calling the calculateSum
function. The function’s parameters a
and b
receive these values, allowing the function to calculate their sum and return the result.
By understanding the relationship between arguments and parameters in Roblox Studio, you can effectively pass data to functions, manipulate it within the function body, and utilize the results as needed. This enables code reusability, flexibility, and dynamic behavior in your Roblox projects.
Passing values as arguments when calling a function
In Roblox Studio, passing values as arguments when calling a function involves providing the necessary data or values that correspond to the function’s parameters. This allows the function to operate on specific input data and produce the desired result. Here’s how you can pass values as arguments when calling a function:
- Identify the Function:
- Identify the function you want to call and understand its parameter requirements.
- Parameters define the type and order of the values you need to pass as arguments.
- Prepare the Values:
- Determine the values you want to pass as arguments.
- Values can be literal values, variables, expressions, or the result of other function calls.
- Make sure the data types and order of the values match the corresponding parameters of the function.
- Call the Function:
- To call a function and pass values as arguments, use the function’s name followed by parentheses
()
. - Inside the parentheses, provide the values you want to pass as arguments, separated by commas.
- The order of the arguments should match the order of the parameters defined in the function.
- To call a function and pass values as arguments, use the function’s name followed by parentheses
- Capture the Return Value:
- If the function returns a value, you can capture it in a variable for further use or display.
- Use the assignment operator (
=
) to assign the return value to a variable.
Here’s an example to demonstrate passing values as arguments when calling a function in Roblox Studio:
function calculateSum(a, b)
local sum = a + b
return sum
end
-- Call the function and pass values as arguments
local num1 = 5
local num2 = 3
local result = calculateSum(num1, num2)
print(result) -- Output: 8
In the example above, the function calculateSum
takes two parameters a
and b
, representing the numbers to be added. When calling the function, the variables num1
and num2
are passed as arguments. The function performs the addition operation using the provided values and returns the sum, which is then captured in the result
variable.
By passing values as arguments when calling a function in Roblox Studio, you can provide specific data for the function to work with. This enables you to perform calculations, transformations, or actions based on the provided values, allowing for dynamic behavior and flexible code execution.
Performing operations and calculations with parameters
In Roblox Studio, parameters in functions allow you to pass values into the function and perform various operations and calculations based on those values. Here’s how you can perform operations and calculations with parameters in Roblox Studio:
- Define Parameters:
- Declare the parameters within the function’s parentheses during its declaration.
- Parameters act as placeholders for the values that will be passed into the function.
- Assign meaningful names to the parameters to indicate their purpose.
- Accessing Parameter Values:
- Inside the function body, you can access the parameter values just like regular variables.
- Use the parameter names to reference the passed values.
- Perform operations, calculations, or any desired manipulations using these values.
- Example of Operations and Calculations:
- You can use the parameter values to perform arithmetic operations such as addition, subtraction, multiplication, or division.
- Example:
function calculateSum(a, b)
local sum = a + b
return sum
end
Conditionals and Control Flow:
- Parameters can be used in conditional statements like
if
,else
, orelseif
. - Based on the parameter values, you can execute different blocks of code or perform specific actions.
- Example:
function isPositive(number)
if number > 0 then
return true
else
return false
end
end
String Manipulation:
- You can manipulate strings using parameters to concatenate, split, or transform them.
- Example:
function greetPlayer(playerName)
local greeting = "Hello, " .. playerName .. "!"
return greeting
end
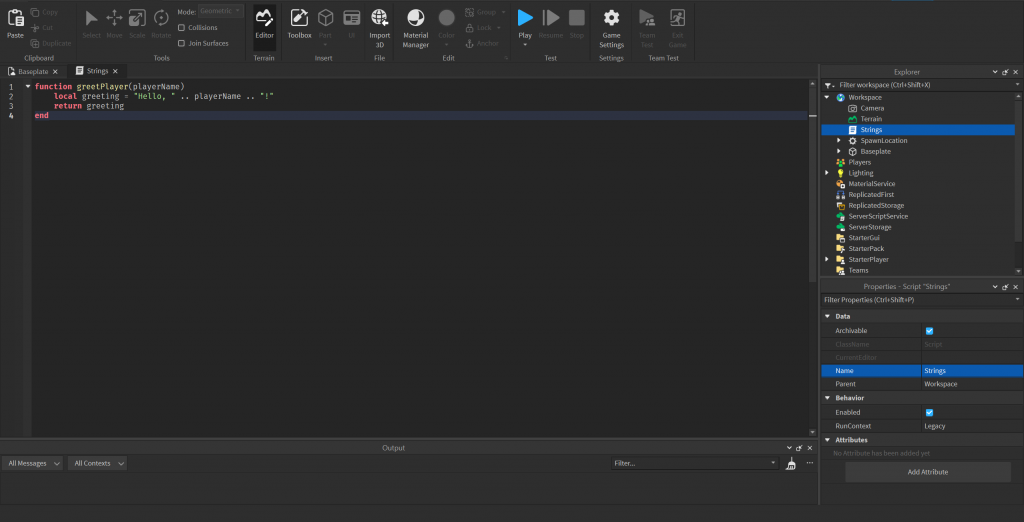
- Mathematical Calculations:
- Parameters can be utilized in complex mathematical calculations, formulas, or algorithms.
- You can perform mathematical operations using the parameter values to obtain the desired results.
By performing operations and calculations with parameters in Roblox Studio, you can create functions that accept input data and generate meaningful output based on that data. The flexibility provided by parameters allows your code to be reusable, adaptable, and dynamic, enabling you to build interactive and engaging experiences in your Roblox games and applications.
Assigning default values to function parameters
In Roblox Studio, you can assign default values to function parameters, which allows you to provide a fallback value in case the caller does not pass a specific argument when calling the function. Default values help ensure that functions can handle different scenarios and provide a sensible result even when certain arguments are not explicitly provided. Here’s how you can assign default values to function parameters in Roblox Studio:
- Declare Parameters with Default Values:
- When declaring the function’s parameters, assign default values to them using the assignment operator (
=
). - The default values should be of the same type as the expected argument or compatible with the parameter’s purpose.
- Example:
- When declaring the function’s parameters, assign default values to them using the assignment operator (
function greetPlayer(playerName, greetingMessage)
playerName = playerName or "Guest" -- Assigns "Guest" if playerName is nil or false
greetingMessage = greetingMessage or "Hello" -- Assigns "Hello" if greetingMessage is nil or false
print(greetingMessage .. ", " .. playerName .. "!")
end
Handling Default Values:
- Inside the function body, you can check if the parameter values are
nil
orfalse
using conditional statements. - If a parameter is
nil
orfalse
, you can assign the default value to it using the logical OR (or
) operator. - The logical OR operator returns the first non-nil or non-false value it encounters.
- Example:
function calculateArea(length, width)
length = length or 0 -- Assigns 0 if length is nil or false
width = width or 0 -- Assigns 0 if width is nil or false
return length * width
end
Calling the Function:
- When calling a function with default parameters, you have the flexibility to provide specific values or omit the arguments.
- If an argument is omitted, the default value assigned to the parameter will be used instead.
- Example:
greetPlayer("John") -- Output: Hello, John!
greetPlayer("Emma", "Hi") -- Output: Hi, Emma!
greetPlayer() -- Output: Hello, Guest!
By assigning default values to function parameters in Roblox Studio, you ensure that your functions can handle different scenarios gracefully and provide meaningful results even when certain arguments are not explicitly provided. This flexibility allows for more robust and versatile function implementations, as well as improved code reusability and ease of use.
Benefits of default parameter values
Assigning default parameter values in Roblox Studio brings several benefits to your code and function implementations. Here are some key advantages of using default parameter values:
- Increased Flexibility:
- Default parameter values provide flexibility when calling functions by allowing you to omit certain arguments.
- This flexibility is particularly useful when a function has optional parameters or when you want to provide a default behavior.
- It simplifies the function call syntax and reduces the need for excessive argument passing.
- Robustness and Error Handling:
- Default values help prevent errors caused by missing or undefined arguments.
- If an argument is not provided, the function can rely on the default value instead of encountering unexpected
nil
values. - This reduces the risk of runtime errors and makes your code more robust and reliable.
- Improved Code Reusability:
- Default parameter values enhance code reusability by making functions more versatile.
- Functions can provide sensible behavior even without all arguments explicitly specified, increasing their usability in different contexts.
- By defining default values within the function, you reduce the need to create multiple versions of similar functions with different argument configurations.
- Easier Maintenance and Evolution:
- Default parameter values simplify the maintenance and evolution of your codebase.
- If you need to introduce new parameters to a function, existing code that calls the function with fewer arguments can still work without modifications.
- Default values allow for backwards compatibility, minimizing the need to update every function call when extending functionality.
- Enhanced Readability and Documentation:
- Default parameter values improve the readability of your code by providing self-documenting behavior.
- When default values are assigned directly within the function declaration, it becomes clearer which values are optional and what they default to.
- This helps other developers understand the intended behavior of the function without explicitly checking its implementation.
Overall, default parameter values in Roblox Studio contribute to more flexible, robust, and maintainable code. They improve the usability and versatility of functions, reduce the likelihood of errors, and enhance the readability and understandability of your codebase. By leveraging default parameter values effectively, you can create more efficient and flexible solutions in your Roblox games and applications.
Understanding function overloading and its limitations in Lua
Function overloading refers to the ability to define multiple functions with the same name but different parameter lists. Each function variant is differentiated by the number, types, or order of its parameters. However, Lua, the scripting language used in Roblox Studio, does not natively support function overloading like some other programming languages. Here’s an explanation of function overloading in Lua and its limitations:
- Function Overloading in Other Languages:
- In languages that support function overloading, such as C++ or Java, you can define multiple functions with the same name but different parameter signatures.
- The compiler or runtime environment determines which function to call based on the arguments provided during the function call.
- This allows for more intuitive and expressive code, as different versions of the same function can be called with different argument combinations.
- Limitations in Lua:
- Lua does not have built-in support for function overloading.
- In Lua, defining multiple functions with the same name will result in the previous definition being overwritten by the latest one.
- Lua’s approach to function definitions follows a “last declaration wins” rule.
- Alternatives in Lua:
- Although function overloading is not directly supported in Lua, there are alternative approaches to achieve similar functionality:
- Use different function names: Instead of overloading a single function, you can define multiple functions with different names that convey their variations.
- Use optional or table-based parameters: You can define a single function with optional parameters or utilize tables to handle different argument configurations.
- Although function overloading is not directly supported in Lua, there are alternative approaches to achieve similar functionality:
- Optional Parameters:
- Lua allows defining functions with default parameter values, as discussed earlier.
- By making certain parameters optional, you can achieve similar effects to function overloading, as the function can handle different argument combinations.
- Table-based Parameters:
- Lua functions can accept tables as arguments.
- By passing different table structures or keys to a function, you can achieve behavior similar to function overloading.
- The function can inspect the table structure or keys to determine how to handle the input.
Despite the lack of native function overloading in Lua, you can still achieve similar functionality through alternative means like using different function names, optional parameters, or table-based parameters. These approaches allow you to handle different argument combinations and provide flexibility in your code. It’s important to consider Lua’s language limitations and choose the most suitable approach based on your specific requirements when working with functions in Roblox Studio.
Creating functions with parameters to perform specific tasks
Creating functions with parameters in Roblox Studio allows you to define reusable blocks of code that can perform specific tasks based on the input provided. Parameters act as placeholders for values that will be passed into the function when calling it. Here’s how you can create functions with parameters to perform specific tasks in Roblox Studio:
- Function Declaration:
- Start by declaring the function using the
function
keyword, followed by the function name. - Inside the parentheses, list the parameters separated by commas.
- Example:
- Start by declaring the function using the
function calculateArea(length, width)
-- Function code goes here
end
Accessing Parameters:
- Inside the function body, you can access the parameter values using their names, treating them as local variables.
- Parameters can be used just like any other variable to perform operations or calculations.
- Example:
function calculateArea(length, width)
local area = length * width
print("The area is: " .. area)
end
Calling the Function:
- To execute the function and perform the specific task, you need to call it and pass the required arguments.
- Provide the values for the parameters in the order they were declared.
- Example:
calculateArea(5, 3) -- Output: The area is: 15
Reusability:
- By defining functions with parameters, you can reuse the same block of code for different inputs.
- Simply call the function with different argument values to perform the specific task with different data.
- Example:
calculateArea(10, 7) -- Output: The area is: 70
calculateArea(8, 8) -- Output: The area is: 64
Parameter Validation:
- You can add validation logic inside the function to ensure that the input values meet certain criteria.
- This can involve checking for specific data types, ranges, or conditions before performing the desired task.
- Example:
function calculateArea(length, width)
if type(length) == "number" and type(width) == "number" then
local area = length * width
print("The area is: " .. area)
else
print("Invalid input. Length and width must be numbers.")
end
end
By creating functions with parameters in Roblox Studio, you can encapsulate specific tasks or operations in reusable blocks of code. Parameters allow you to customize the behavior of the function based on the input data provided when calling it. This modular approach enhances code organization, reusability, and readability, making it easier to build complex and dynamic functionalities in your Roblox games and applications.
Passing different data types as arguments to functions
In Roblox Studio, you can pass different data types as arguments to functions, allowing for flexible and versatile code. Here’s a guide on how to pass different data types as arguments to functions in Roblox Studio:
- Primitive Data Types:
- Numbers: You can pass numeric values, such as integers or floating-point numbers, as arguments to functions. Example:
function myFunction(numberArg) ... end
- Strings: You can pass text values enclosed in quotes as string arguments. Example:
function myFunction(stringArg) ... end
- Booleans: You can pass
true
orfalse
as boolean arguments. Example:function myFunction(booleanArg) ... end
- Numbers: You can pass numeric values, such as integers or floating-point numbers, as arguments to functions. Example:
- Tables:
- Tables are a versatile data type in Lua and can be used to pass complex or structured data to functions.
- You can create a table and pass it as an argument. Example:
function myFunction(tableArg) ... end
- Tables can contain a mix of data types, including numbers, strings, booleans, and even other tables.
- Instances:
- Roblox Studio is built around game instances like parts, models, and other objects.
- You can pass instances as arguments to functions to manipulate or interact with them.
- Example:
function myFunction(instanceArg) ... end
- Functions:
- Functions are first-class citizens in Lua, meaning you can pass functions as arguments to other functions.
- This enables powerful techniques like callbacks and event handling.
- Example:
function myFunction(callbackArg) ... end
- User-defined Types:
- In addition to the built-in data types, you can create and pass your own custom data types as arguments.
- By defining classes or data structures, you can encapsulate related data and behavior and pass instances of these types as arguments.
- Example:
function myFunction(customTypeArg) ... end
- Type Checking:
- It’s a good practice to perform type checking within the function if you have specific requirements for the argument data types.
- Use conditional statements or type-checking functions like
type()
to ensure the received argument matches the expected data type. - Example:
function myFunction(numberArg)
if type(numberArg) == "number" then
-- Perform operations with the number argument
else
error("Invalid argument type. Expected number.")
end
end
By allowing different data types as arguments, you can create more flexible and reusable functions in Roblox Studio. Whether you’re working with primitive data types, tables, instances, functions, or custom types, understanding how to pass and handle different data types enables you to build dynamic and interactive experiences in your Roblox games and applications.
Conclusion
Functions with parameters in Roblox Studio provide a powerful way to create reusable and customizable blocks of code. By defining functions that accept arguments, you can perform specific tasks based on the provided input. Here’s a summary of the benefits and key takeaways:
- Reusability: Functions with parameters allow you to encapsulate a specific task or operation that can be reused throughout your code. By calling the function with different argument values, you can perform the task with varying data.
- Flexibility: Parameters make functions flexible, as they can accept different data types and values. This flexibility enables your functions to handle a wide range of inputs and adapt to different scenarios.
- Customization: Parameters provide a way to customize the behavior of a function. By passing different values as arguments, you can control how the function operates and produces results.
- Validation and Error Handling: You can include validation logic within your functions to ensure that the provided arguments meet specific criteria. This helps to prevent errors and ensures the function operates as intended.
- Improved Code Organization and Readability: Functions with parameters enhance code organization by breaking down complex tasks into modular components. They also improve code readability by clearly indicating the required input and expected behavior.
- Enhanced Collaboration: Functions with parameters make it easier for multiple developers to work on a project. By clearly defining the input and output requirements, functions with parameters promote better collaboration and understanding among team members.
By leveraging functions with parameters, you can create more modular, reusable, and maintainable code in Roblox Studio. They provide flexibility, customization, and improved code organization, making it easier to build dynamic and interactive experiences in your Roblox games and applications.