Server Script Analysis:
This Lua script demonstrates a simple implementation of an inventory system using tables and functions. Let’s break down the script and analyze its components:
First of all, put script in server script service.

Inventory Table Creation:
local Inventory = {}
addItem Function:
function Inventory:addItem(itemID) if self[itemID] then self[itemID] = self[itemID] + 1 else self[itemID] = 1 end print(itemID.." added to inventory") end
- This function adds an item to the inventory.
- It takes
itemID
as a parameter. - If the item already exists in the inventory, its quantity is incremented by 1.
- If the item doesn’t exist, it’s added to the inventory with a quantity of 1.
- It prints a message indicating the addition of the item to the inventory.
removeItem Function:
function Inventory:removeItem(itemID) if self[itemID] then self[itemID] = self[itemID] - 1 if self[itemID] <= 0 then self[itemID] = nil end else print("Item not found in inventory") end end
- This function removes an item from the inventory.
- It takes
itemID
as a parameter. - If the item exists in the inventory, its quantity is decremented by 1.
- If the quantity becomes zero or less, the item is removed from the inventory.
- If the item doesn’t exist, it prints a message indicating that the item was not found in the inventory.
useItem Function:
function Inventory:useItem(itemID) if self[itemID] then self:removeItem(itemID) print("Used item: "..itemID) else print("Item not found in inventory") end end
- This function simulates the usage of an item from the inventory.
- It takes
itemID
as a parameter. - If the item exists in the inventory, it removes the item using the
removeItem
function and prints a message indicating that the item has been used. - If the item doesn’t exist, it prints a message indicating that the item was not found in the inventory.
BindableEvent Connection:
local BindableEventInstance = game.ReplicatedStorage:FindFirstChild("addItem") BindableEventInstance.Event:Connect(function(itemType) Inventory:addItem(itemType) end)
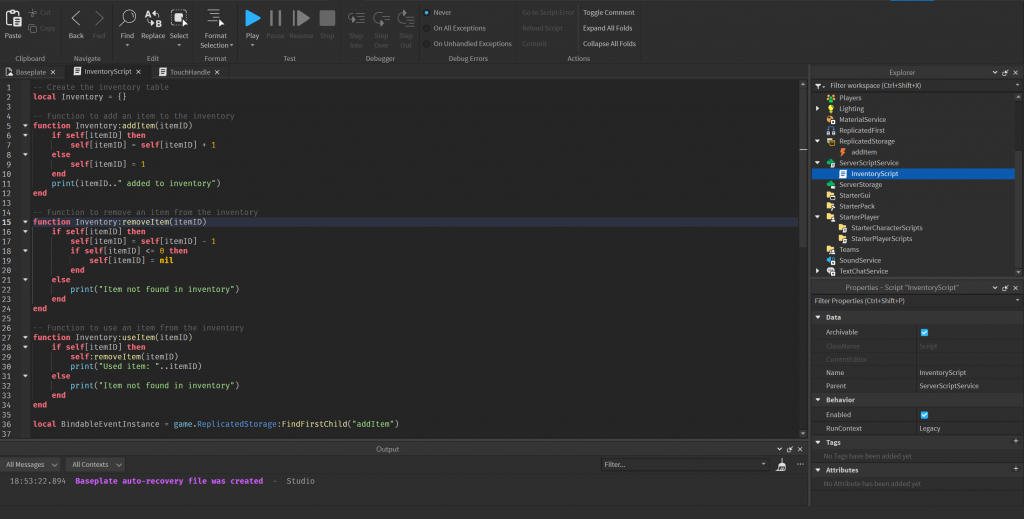
How to add Bindable Event?
In Roblox Studio, you can add a bindable event to ReplicatedStorage using Lua scripting. Here’s a step-by-step guide:
- Locate ReplicatedStorage: In the Explorer window, find and select “ReplicatedStorage.” If it’s not visible, you can find it under the “DataModel” object.
- Insert BindableEvent: Right-click on ReplicatedStorage in the Explorer window, hover over “Insert Object,” and select “BindableEvent” from the menu. This will create a new BindableEvent object under ReplicatedStorage.
- Rename the BindableEvent (Optional): Rename the BindableEvent to “addItem”

- This part connects a BindableEvent named “addItem” to a function.
- Whenever the “addItem” event is fired, it triggers the anonymous function defined inside
Connect
. - The anonymous function calls the
addItem
function of theInventory
table, passing theitemType
received as a parameter.
Lesson: This script illustrates a fundamental implementation of an inventory management system in Lua. Here are some key takeaways:
- Tables and Functions: Lua’s tables are versatile data structures used to implement various data types. Functions can be defined within tables to create object-oriented structures.
- Inventory Management: The script demonstrates basic inventory operations such as adding, removing, and using items.
- Event Handling: The use of BindableEvents showcases event-driven programming, where actions in one part of the program (e.g., adding an item) trigger responses in another part (e.g., updating the inventory).
- Error Handling: The script includes basic error handling to deal with situations like attempting to remove or use an item that doesn’t exist in the inventory.
Understanding this script can serve as a foundation for developing more complex inventory systems in Lua-based game development or other applications.
Full Server script
-- Create the inventory table
local Inventory = {}
-- Function to add an item to the inventory
function Inventory:addItem(itemID)
if self[itemID] then
self[itemID] = self[itemID] + 1
else
self[itemID] = 1
end
print(itemID.." added to inventory")
end
-- Function to remove an item from the inventory
function Inventory:removeItem(itemID)
if self[itemID] then
self[itemID] = self[itemID] - 1
if self[itemID] <= 0 then
self[itemID] = nil
end
else
print("Item not found in inventory")
end
end
-- Function to use an item from the inventory
function Inventory:useItem(itemID)
if self[itemID] then
self:removeItem(itemID)
print("Used item: "..itemID)
else
print("Item not found in inventory")
end
end
local BindableEventInstance = game.ReplicatedStorage:FindFirstChild("addItem")
BindableEventInstance.Event:Connect(function(itemType)
Inventory:addItem(itemType)
end)
Part Script Overview
First what you need to do, is to insert part into workspace and add script to it.

local debounce = false
- This line declares a variable
debounce
and initializes it tofalse
. The purpose of this variable is to prevent rapid firing of events.
script.Parent.Touched:Connect(function(hit)
if debounce then
return -- If debounce is true, exit the function immediately
end
- This line connects a function to the
Touched
event of the part to which the script is attached. When any object touches this part, the function specified insideConnect
will be executed. - Inside the function, it checks if
debounce
istrue
. If it is, the function exits immediately, preventing further execution.
local character = hit.Parent
local player = game.Players:GetPlayerFromCharacter(character)
if player then
- These lines retrieve the character that touched the part and attempt to get the player associated with that character.
local BindableEventInstance = game.ReplicatedStorage:FindFirstChild("addItem")
- This line finds a BindableEvent object named “addItem” in the ReplicatedStorage. ReplicatedStorage is a location where objects are stored and can be accessed by both the server and the client.
BindableEventInstance:Fire("orange")
- This line fires the “addItem” event with the argument “orange”. This event is likely meant to signal to the server that the player should receive an item named “orange”.
debounce = true -- Set debounce to true to start the cooldown
- This line sets
debounce
totrue
, indicating that the script is currently on cooldown to prevent rapid execution.
wait(2)
debounce = false -- Set debounce back to false to allow the script to run again
- These lines introduce a cooldown mechanism. After firing the event, the script waits for 2 seconds before resetting
debounce
back tofalse
, allowing the script to run again.
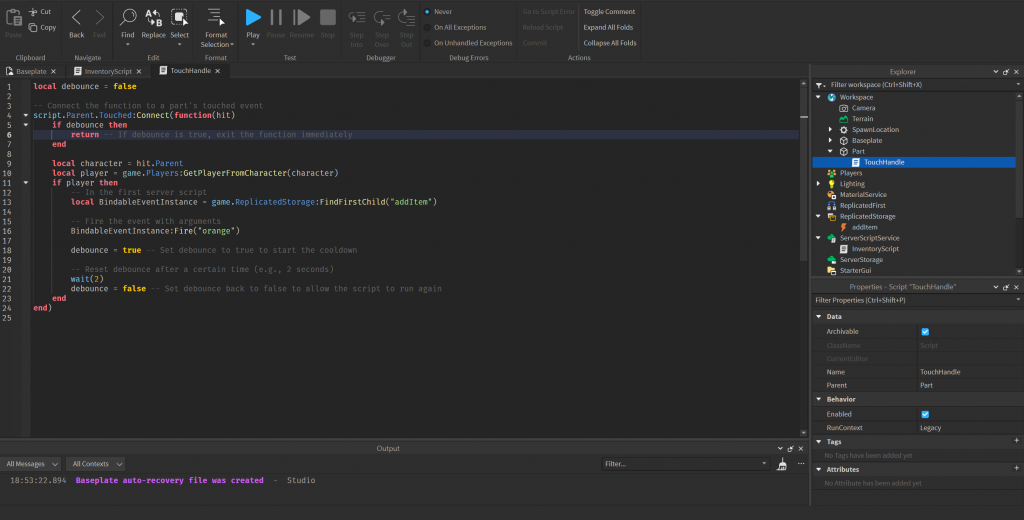
Full Part script
local debounce = false
-- Connect the function to a part's touched event
script.Parent.Touched:Connect(function(hit)
if debounce then
return -- If debounce is true, exit the function immediately
end
local character = hit.Parent
local player = game.Players:GetPlayerFromCharacter(character)
if player then
-- In the first server script
local BindableEventInstance = game.ReplicatedStorage:FindFirstChild("addItem")
-- Fire the event with arguments
BindableEventInstance:Fire("orange")
debounce = true -- Set debounce to true to start the cooldown
-- Reset debounce after a certain time (e.g., 2 seconds)
wait(2)
debounce = false -- Set debounce back to false to allow the script to run again
end
end)
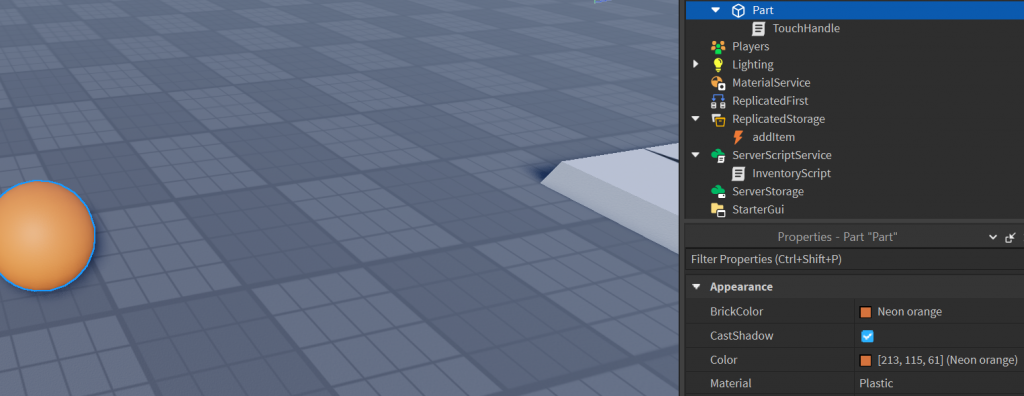
Conclusion
The provided script exemplifies fundamental concepts of scripting in Roblox, showcasing event handling, variable usage, and communication between client and server. Here’s a summary of the key takeaways from analyzing this script:
- Event Handling: The script utilizes the
Touched
event of a part to trigger its functionality when another object makes contact with it. This event-driven approach allows for interactive gameplay elements within a Roblox game. - Variable Usage: The
debounce
variable serves as a mechanism to prevent rapid execution of the script. By togglingdebounce
betweentrue
andfalse
, the script controls the frequency at which it can be triggered, thus avoiding unintended behaviors or exploits. - Communication with Server: Through the use of a
BindableEvent
, the script communicates with the server to trigger an action—adding an item to a player’s inventory. This demonstrates the importance of server-client communication for implementing game mechanics that require server-side validation or data manipulation. - Cooldown Mechanism: The script implements a simple cooldown mechanism using the
wait
function. After firing the event to add an item, the script waits for a specified duration before allowing itself to be triggered again. This prevents spamming of the action, ensuring fair gameplay and server performance.
In summary, this script serves as a foundational example of how scripting is employed in Roblox to create interactive and dynamic experiences. By understanding and mastering these scripting concepts, developers can craft engaging gameplay mechanics and features for their Roblox games.