Roblox Studio, a powerful game development platform, allows developers to create immersive experiences through Lua scripting. In this article, we’ll explore a Lua script designed to dynamically change the shape and size of a part within a Roblox game. The script utilizes key concepts such as CFrame, Vector3, functions, and loops to achieve its purpose.
Parent Assignment:
local part = script.Parent
The script starts by creating a local variable named part
and assigning it the value of script.Parent
. This assumes that the script is a child of the part you intend to modify, providing a reference to the specific part within the script.
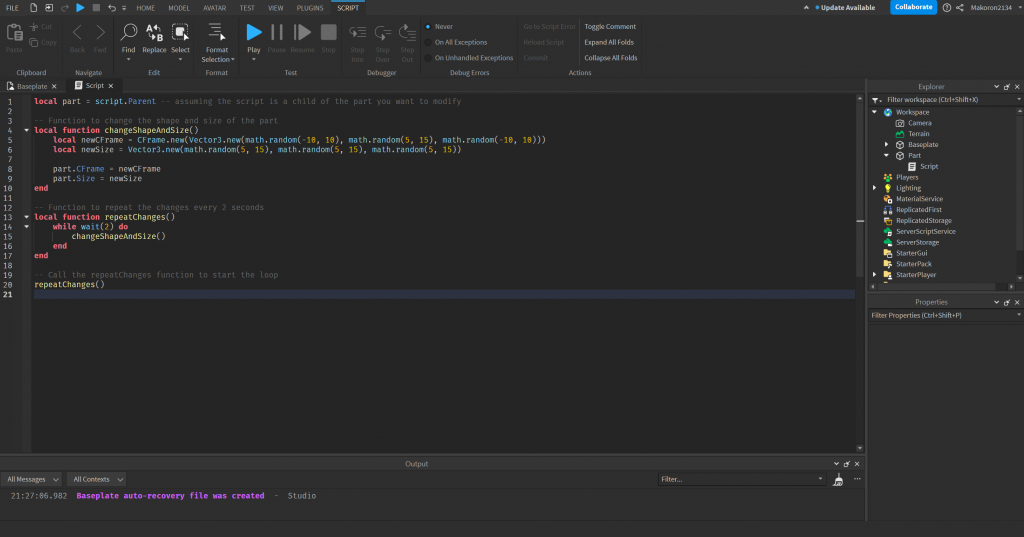
changeShapeAndSize Function:
local function changeShapeAndSize() local newCFrame = CFrame.new(Vector3.new(math.random(-10, 10), math.random(5, 15), math.random(-10, 10))) local newSize = Vector3.new(math.random(5, 15), math.random(5, 15), math.random(5, 15)) part.CFrame = newCFrame part.Size = newSize end
This function, changeShapeAndSize
, is responsible for altering the shape and size of the specified part. It generates random values for the new CFrame and Size using the math.random
function within a specific range. Finally, it assigns these values to the part’s CFrame
and Size
properties, effectively changing its appearance.
repeatChanges Function:
local function repeatChanges() while wait(2) do changeShapeAndSize() end end
The repeatChanges
function contains a loop that waits for 2 seconds (wait(2)
) and then calls the changeShapeAndSize
function. This loop continues indefinitely, creating a continuous cycle of shape and size changes every 2 seconds.
Executing the Loop:
repeatChanges()
Finally, the script calls the repeatChanges
function to initiate the loop. This ensures that the changeShapeAndSize
function is repeatedly executed at regular intervals, creating a dynamic and ever-changing appearance for the specified part.
Conclusion
This script exemplifies the dynamic nature of Lua scripting in Roblox Studio, showcasing how developers can use functions, loops, and mathematical operations to bring life to their in-game objects. By understanding the principles behind this script, developers can apply similar techniques to enhance the visual and interactive aspects of their Roblox games.