Roblox Studio allows developers to create interactive and engaging experiences by incorporating scripts that respond to various events. One powerful feature is the ClickDetector, which enables you to trigger actions when a player clicks on a specific part in the game world. In this article, we’ll explore a simple teleportation script that utilizes a ClickDetector to move a part to a predefined destination.
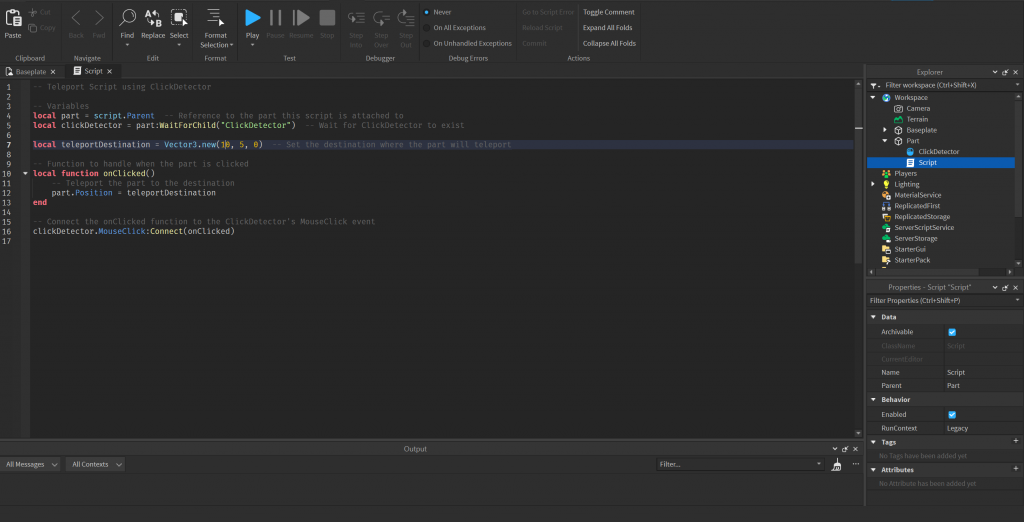
Understanding the Script
Let’s break down the provided script to understand its components and functionality:
-- Teleport Script using ClickDetector
-- Variables
local part = script.Parent -- Reference to the part this script is attached to
local clickDetector = part:WaitForChild("ClickDetector") -- Wait for ClickDetector to exist
local teleportDestination = Vector3.new(10, 5, 0) -- Set the destination where the part will teleport
The script begins by declaring variables. part
is a reference to the part the script is attached to, providing context for the teleportation. clickDetector
is assigned the ClickDetector associated with the part, which is crucial for detecting player interactions. The script also sets the teleportDestination
variable to a specific Vector3 position, indicating where the part will be teleported when clicked.
-- Function to handle when the part is clicked
local function onClicked()
-- Teleport the part to the destination
part.Position = teleportDestination
end
The core functionality is encapsulated within the onClicked
function. When the player clicks on the part, this function is invoked. Inside the function, the script updates the Position
property of the part, effectively teleporting it to the specified destination.
-- Connect the onClicked function to the ClickDetector's MouseClick event
clickDetector.MouseClick:Connect(onClicked)
The final section of the script establishes a connection between the onClicked
function and the MouseClick
event of the ClickDetector. This ensures that when a player clicks on the associated part, the teleportation function is triggered.
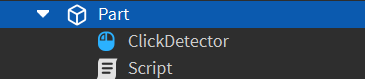
Implementing the Script
To use this teleportation script in your Roblox game, follow these steps:
- Attach the script to the part you want to be clickable.
- Make sure the part has a ClickDetector as a child. You can add one manually in the Roblox Studio editor.
- Adjust the
teleportDestination
variable to set the desired teleportation destination.
This script provides a foundation for creating interactive elements in your game, allowing players to teleport to different locations with a simple click. As you continue developing your game in Roblox Studio, you can build upon this script to create more complex and immersive interactions.