Implementing gameplay mechanics in 3D games in Unity involves a combination of designing and programming various systems and interactions that define how the game behaves and how players can interact with it. These mechanics are crucial in creating engaging and enjoyable gameplay experiences. In this overview, we’ll explore the key aspects of implementing gameplay mechanics in Unity.
- Designing Gameplay Mechanics: The first step in implementing gameplay mechanics is to design them. This involves conceptualizing and planning the various actions, behaviors, and systems that players will encounter in the game. This may include movement controls, combat mechanics, puzzles, AI behavior, and more. It’s important to have a clear understanding of the desired player experience and iterate on the design until it feels fun and balanced.
- Game Objects and Components: In Unity, gameplay mechanics are typically implemented using game objects and components. Game objects are the basic building blocks of a scene and can represent characters, items, obstacles, or any other interactive element. Components are attached to game objects and define their behavior. For example, a character controller component may handle player movement, while a weapon component may govern the behavior of a weapon.
- Physics and Collisions: Realistic and responsive physics are often an important part of 3D gameplay mechanics. Unity provides a built-in physics engine that allows you to simulate the laws of physics in your game. You can use rigidbody components to apply forces, gravity, and collisions to game objects. This enables actions such as jumping, falling, object interactions, and environmental interactions.
- Scripting and Programming: To implement gameplay mechanics in Unity, you’ll need to write scripts and code. Unity supports multiple programming languages, including C# and UnityScript. You can create scripts that control the behavior of game objects, handle input from the player, manage game state, and more. By scripting, you can define how mechanics like character movement, enemy AI, and interactive objects function in the game.
- Animation and Visual Feedback: Gameplay mechanics are not just about functionality; they also involve providing visual and audio feedback to the player. Unity offers powerful animation tools that allow you to create animations for characters, objects, and environmental elements. By animating the movements and interactions, you can enhance the visual appeal and make the gameplay mechanics feel more immersive and responsive.
- Iteration and Playtesting: Implementing gameplay mechanics is an iterative process. It’s essential to playtest and iterate on the mechanics to ensure they are enjoyable, balanced, and free from bugs or issues. Regular playtesting allows you to gather feedback and make necessary adjustments to improve the gameplay experience. Unity provides tools for quick iteration, allowing you to make changes, test them, and refine the mechanics efficiently.
- Optimizing Performance: As your game grows in complexity, it’s crucial to optimize performance to ensure smooth gameplay. This involves techniques such as optimizing physics calculations, implementing efficient AI algorithms, and reducing unnecessary computations. Unity provides profiling tools that help you identify performance bottlenecks and optimize your gameplay mechanics to deliver a smooth and responsive experience.
In summary, implementing gameplay mechanics in 3D games in Unity involves a combination of designing, scripting, and programming interactive systems, along with providing visual and audio feedback. With Unity’s powerful tools and features, developers can create immersive and engaging gameplay experiences that captivate players and bring their game worlds to life.
Implementing Basic 3D Character Movement
Implementing basic 3D character movement in Unity is a fundamental aspect of creating a playable character that can navigate and explore the game world. This involves setting up input controls, handling player movement, and applying physics for realistic interactions. In this overview, we’ll discuss the key steps to implement basic 3D character movement in Unity.
- Character Controller: Unity provides a built-in component called Character Controller, which is ideal for implementing basic character movement. The Character Controller allows you to handle character physics and collisions without requiring a full rigidbody component. To begin, create a new GameObject and attach the Character Controller component to it.
- Input Handling: To control the character’s movement, you need to handle player input. Unity provides input handling through the Input class, which allows you to detect keyboard, mouse, or controller inputs. In your script, you can use methods like Input.GetAxis to retrieve the input values for horizontal and vertical movement.
- Translate the Character: Once you have the input values, you can translate the character based on them. In your script, use the transform.Translate method to move the character in the desired direction. Multiply the input values with the character’s speed to control the movement speed. You can also use Time.deltaTime to ensure smooth movement across different frame rates.
- Gravity and Jumping: To create realistic movement, you’ll want to apply gravity to the character and implement jumping mechanics. Use the Character Controller’s Move method to handle gravity and falling. By applying a downward force over time, you can simulate the effect of gravity. Additionally, you can detect a jump input and add an upward force to the character to simulate jumping.
- Collision Detection: Collision detection is crucial to prevent the character from passing through walls or objects. The Character Controller component handles simple collision detection automatically. However, you may need to set up additional colliders on the environment or other objects to ensure proper interaction. You can use Unity’s built-in colliders (BoxCollider, SphereCollider, etc.) or create custom colliders as needed.
- Camera Movement: In a 3D game, the character’s movement is often accompanied by camera movement to provide a dynamic perspective. You can attach the camera to a separate GameObject and use scripting to align it with the character’s position and rotation. By adjusting the camera’s position and rotation based on the character’s movement, you can create a smooth and responsive camera movement.
- Testing and Refinement: It’s essential to playtest and iterate on the character movement to ensure it feels responsive and natural. Test the movement in different environments and situations to identify any issues or areas for improvement. Adjust the movement speed, acceleration, and other parameters as necessary to achieve the desired gameplay feel.
Remember, the implementation of character movement can vary depending on the specific requirements of your game. For more advanced movement mechanics, such as sprinting, crouching, or climbing, you may need to extend the basic implementation or consider additional components or scripts.
By following these steps and iterating on your implementation, you can create a solid foundation for character movement in your 3D Unity game. Building upon this foundation, you can expand and refine your character’s movement mechanics to suit the unique gameplay experience you aim to deliver.
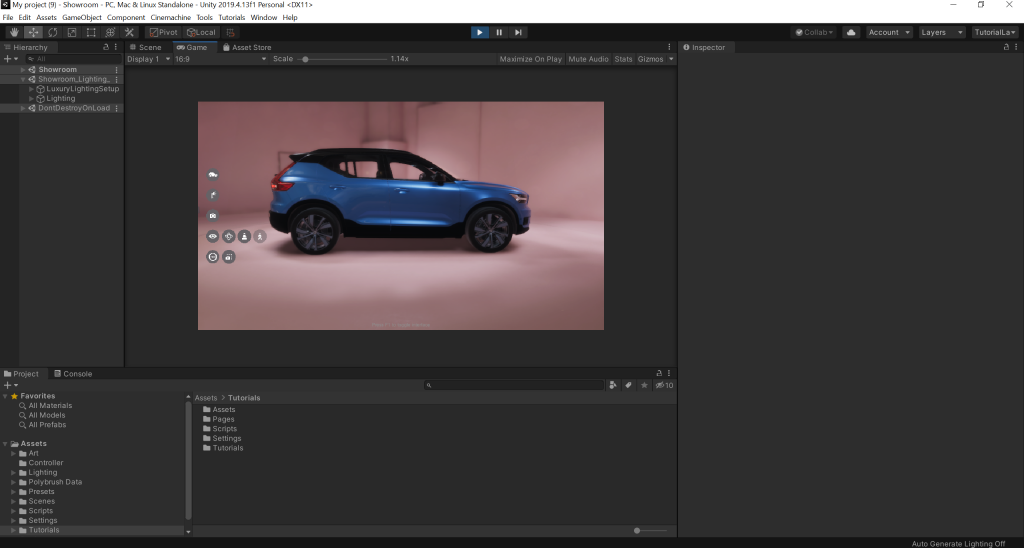
Creating and configuring colliders for game objects
Creating and configuring colliders for 3D game objects in Unity is crucial for enabling collision detection and interaction between objects in your game world. Colliders define the shape and size of an object’s physical presence, allowing you to detect when objects come into contact and respond accordingly. In this overview, we’ll discuss the steps to create and configure colliders in Unity.
- Types of Colliders: Unity provides several types of colliders, each suited for different shapes and purposes. The commonly used colliders include:
- BoxCollider: Represents a box-shaped collider.
- SphereCollider: Represents a sphere-shaped collider.
- CapsuleCollider: Represents a capsule-shaped collider.
- MeshCollider: Uses a custom mesh shape for collision detection.
- CharacterController: Specifically designed for character movement and physics interaction.
- Adding a Collider to an Object: To add a collider to a game object, select the object in the Unity Editor’s Hierarchy or Scene view. Then, in the Inspector window, click the “Add Component” button and search for the desired collider type. Selecting the collider component will attach it to the game object.
- Adjusting Collider Properties: Once the collider is added, you can configure its properties in the Inspector window. The available properties depend on the collider type but often include parameters such as size, radius, height, and center. Adjust these properties to match the object’s shape and size accurately.
- Collider Size and Position: The size and position of the collider determine the area that is considered for collision detection. For example, a BoxCollider’s size defines the dimensions of the box-shaped collider. Ensure that the collider encompasses the visible part of the object and accurately represents its physical presence.
- Complex Colliders with MeshCollider: In some cases, you may need to create colliders for objects with more complex shapes, such as detailed 3D models. Unity’s MeshCollider allows you to use a custom mesh shape for collision detection. To do this, assign the appropriate mesh to the MeshCollider component. Ensure that the mesh’s geometry matches the object’s shape for precise collision detection.
- Compound Colliders: Unity also supports compound colliders, which are colliders composed of multiple primitive shapes. By combining colliders, you can create more complex collision areas. For example, you can attach multiple BoxColliders or SphereColliders to a single game object, each representing a different part of the object’s collision shape.
- Trigger Colliders: In addition to standard colliders, Unity provides trigger colliders. Trigger colliders are not affected by physics simulation but instead invoke events when other objects enter or exit their area. This is useful for implementing interactions such as triggering events, detecting pickups, or activating specific game mechanics.
- Collision Detection and Response: To handle collision detection and response, you can use Unity’s physics system, which utilizes colliders and rigidbody components. By attaching rigidbody components to objects, you enable physics interactions, including forces, gravity, and bouncing. You can then use events and scripts to handle collisions and respond accordingly, such as applying damage, playing sound effects, or triggering specific behaviors.
- Testing and Iteration: After setting up colliders, it’s important to playtest and iterate to ensure accurate collision detection and desired interactions. Test different scenarios, object sizes, and collision angles to ensure that the colliders are functioning as intended. Make adjustments to the collider properties as needed to achieve the desired results.
By following these steps, you can create and configure colliders for 3D game objects in Unity. Colliders enable realistic collision detection, physics interactions, and gameplay mechanics within your game world. By refining and fine-tuning your colliders, you can create immersive and engaging experiences for your players.
Implementing physics-based interactions
Implementing physics-based interactions in Unity allows you to create dynamic and realistic gameplay experiences. Whether it’s applying forces to objects, detecting triggers, or simulating physical interactions, Unity provides a robust physics engine that can handle these interactions. In this overview, we’ll explore the steps to implement physics-based interactions in Unity.
- Rigidbodies and Colliders: To enable physics interactions, you need to work with two key components in Unity: Rigidbodies and Colliders. Rigidbodies simulate the physics behavior of an object, such as gravity, forces, and collisions. Colliders define the shape and size of an object’s physical presence for collision detection. To start, attach a Rigidbody component to the game object that requires physics interactions.
- Applying Forces: Applying forces to objects allows you to create movement or reactions based on physics. You can use the Rigidbody’s AddForce method to apply forces in different directions. For example, you can apply a force when an object is hit by a projectile, pushed by the player, or affected by an explosion. Experiment with different force values and directions to achieve the desired behavior.
- Detecting Collisions and Triggers: Unity provides collision detection functionality through events and methods. By attaching Colliders to objects, you can detect when collisions occur. There are two ways to handle collisions: OnCollisionEnter/Stay/Exit events and OnCollisionEnter/Stay/Exit methods. These events and methods allow you to trigger specific actions when collisions happen, such as playing a sound effect, spawning particles, or applying damage.
- Trigger Colliders and OnTrigger Events: In addition to standard colliders, you can use trigger colliders to detect when objects enter or exit a specific area without physically interacting with them. Trigger colliders are useful for implementing interactions like pickups, area-based effects, or triggers for events. By marking a collider as a trigger and attaching a script with OnTriggerEnter/Stay/Exit events, you can respond to objects entering or leaving the trigger area.
- Interacting with Constraints and Joints: Unity provides additional components like Hinge Joint, Fixed Joint, and Spring Joint that allow you to simulate more complex physics-based interactions. Joints and constraints enable you to create systems such as swinging doors, rope-like objects, or articulated mechanisms. These components provide control over constraints, limits, and behaviors of connected objects.
- Physics Materials: Physics materials allow you to control friction and bounce properties of colliders. By adjusting the friction, bounciness, and other parameters of a physics material, you can fine-tune the behavior of objects when they collide or slide against surfaces. This can significantly impact the realism and feel of your physics-based interactions.
- Iteration and Playtesting: Implementing physics-based interactions requires iteration and playtesting to ensure desired behavior and optimal performance. Experiment with different forces, collision settings, and physics material properties. Test your interactions in various scenarios and environments to identify and address any issues or unexpected behavior. Use the Unity Profiler to optimize performance if needed.
Remember, implementing physics-based interactions in Unity requires careful balancing and tuning to achieve the desired gameplay experience. It’s essential to find the right combination of forces, collisions, and physics settings to create engaging and realistic interactions within your game.
By following these steps and experimenting with Unity’s physics engine, you can implement physics-based interactions that bring your game world to life, providing immersive and dynamic experiences for your players.
Creating UI elements
Creating UI elements in Unity 3D allows you to design and display interactive user interfaces for your game. UI elements provide important information, feedback, and controls for players, enhancing their overall experience. In this overview, we’ll discuss the steps to create UI elements in Unity 3D.
- Canvas: Start by creating a Canvas, which serves as a container for all UI elements. The Canvas acts as a 2D plane within the 3D game world and provides a rendering surface for UI elements. In the Unity Editor, go to GameObject -> UI -> Canvas to create a new Canvas.
- UI Elements: Unity provides various UI elements that you can use to create your interface. Some commonly used UI elements include:
- Text: Used to display text-based information to the player.
- Image: Used to display static or dynamic images or icons.
- Button: Allows player interaction through clicking or tapping.
- Slider: Enables players to adjust values within a specified range.
- Toggle: Represents a binary on/off state controlled by the player.
- Dropdown: Displays a list of options for the player to choose from.
- Anchoring and Positioning: After adding a UI element, position it within the Canvas. Unity provides anchoring and positioning options to control how UI elements behave when the screen size changes. You can anchor UI elements to specific corners, edges, or the center of the Canvas. Adjust the position, size, and anchor settings of each UI element to achieve the desired layout.
- Styling and Appearance: Customize the appearance of UI elements to match the visual style of your game. Unity allows you to modify various properties, such as colors, fonts, sizes, and textures. Use the Inspector window to access and modify these properties. You can also use image assets and sprites to apply visual elements to UI elements.
- Interactivity and Event Handling: UI elements can be made interactive by attaching scripts and event handlers. For example, you can associate a function with a button’s onClick event to trigger a specific action when the button is clicked. Use Unity’s Event System and Event Trigger components to handle user input and create dynamic interactions.
- UI Layouts: Unity provides layout systems to help organize and arrange UI elements. You can use layouts like Horizontal Layout Group, Vertical Layout Group, or Grid Layout Group to automatically position and resize UI elements based on specific rules. These layouts simplify the process of creating responsive and dynamic UIs.
- Testing and Iteration: Test your UI elements in different resolutions, aspect ratios, and devices to ensure they adapt and scale correctly. Playtest your game to evaluate the usability, readability, and functionality of the UI elements. Make adjustments to the layout, positioning, and appearance based on feedback and usability considerations.
- Animation and Transitions: To add visual effects or create transitions between different UI states, you can utilize Unity’s animation system. You can animate UI elements to fade in or out, slide in or out, or scale in size, providing a more polished and engaging user experience.
By following these steps, you can create UI elements in Unity 3D to enhance your game’s user interface. Carefully design and iterate on your UI elements to ensure they provide clear communication, intuitive interaction, and an aesthetically pleasing visual presentation.
Designing levels and environments for gameplay mechanics
Designing levels and environments for gameplay mechanics in Unity is a crucial aspect of creating engaging and immersive games. Well-designed levels can enhance player experience, challenge their skills, and showcase your gameplay mechanics. In this overview, we’ll discuss the steps to design levels and environments in Unity.
- Define Gameplay Mechanics: Before designing levels, it’s important to have a clear understanding of your game’s core gameplay mechanics. Identify the unique mechanics and interactions that players will engage with. This includes movement abilities, combat mechanics, puzzles, platforming elements, or any other gameplay systems that define your game.
- Establish Design Goals: Define the goals and objectives of your level design. Determine the desired player experience, whether it’s exploration, challenge, narrative progression, or a combination of elements. Consider the pacing, difficulty progression, and variety of challenges throughout the game.
- Plan the Level Layout: Start by sketching or creating a high-level overview of your level layout. Consider the flow and progression of the gameplay, including the starting point, key objectives, and potential branching paths. Identify areas for combat encounters, puzzles, platforming sections, or other interactions based on your gameplay mechanics.
- Iterate and Playtest: Level design is an iterative process. Create a rough prototype of your level and playtest it to gather feedback and evaluate its gameplay flow. Make necessary adjustments to improve pacing, challenge, and player experience. Repeat this process multiple times until you achieve the desired gameplay balance and quality.
- Create the Environment: Use Unity’s Scene view and assets to build the visual environment of your level. Place objects, props, terrain, and other assets to create a cohesive and immersive world. Consider the theme, art style, and narrative of your game when designing the environment. Experiment with lighting, materials, and particle effects to enhance the atmosphere.
- Use Level Design Techniques: Utilize level design techniques to guide players, create interest, and communicate information. These techniques include:
- Landmark Placement: Use prominent and visually distinct elements as landmarks to help players orient themselves within the level.
- Signposting: Use visual cues, lighting, or UI elements to draw attention to important areas or objects.
- Clear Pathways: Ensure players can navigate through the level intuitively by providing clear pathways and reducing unnecessary obstructions.
- Visual Variations: Add visual variety and diversity to the level to maintain player interest and avoid monotony.
- Reward Placement: Strategically place rewards, collectibles, or power-ups to incentivize exploration and reward player progress.
- Balancing and Difficulty: Balance the difficulty of your level by gradually introducing and scaling challenges based on the player’s skill progression. Provide a mix of easy, medium, and hard sections to keep players engaged. Playtest the level to ensure it offers appropriate difficulty, avoiding frustrating or overly simplistic experiences.
- Audio and Music: Enhance the level’s atmosphere and immersion by incorporating audio and music. Use ambient sounds, environmental effects, and music tracks to create an audio landscape that complements the level’s theme and gameplay moments.
- Accessibility Considerations: Keep accessibility in mind when designing levels. Ensure that the level is playable and enjoyable for a wide range of players, including those with different abilities or preferences. Provide options for difficulty settings, adjustable controls, and clear visual and audio cues.
- Documentation and Communication: Document your level design decisions, guidelines, and iteration processes to facilitate collaboration with your team members. Use visual aids such as sketches, diagrams, or concept art to effectively communicate your design intentions.
By following these steps and principles, you can create well-designed levels and environments that effectively showcase and complement your gameplay mechanics in Unity. Iteration, playtesting, and player feedback are essential to refine and optimize your level designs, leading to a more enjoyable and immersive player experience.
Conclusion
Implementing gameplay mechanics in 3D games using Unity opens up a world of possibilities for creating immersive and engaging experiences for players. Throughout this overview, we explored the process of implementing various gameplay mechanics, including character movement, physics-based interactions, health and damage systems, and UI elements.
By leveraging Unity’s powerful tools and features, developers can bring their game mechanics to life. Creating 3D character movement involves utilizing Unity’s input system, animations, and physics components to provide fluid and responsive controls. Implementing physics-based interactions allows for realistic and dynamic gameplay experiences, from applying forces to objects to detecting triggers and collisions.
Handling health and damage systems for players and enemies adds depth and consequences to gameplay interactions. By managing health components, calculating damage, and incorporating death or defeat handling, players can experience challenging and rewarding encounters. Furthermore, creating UI elements in Unity provides essential information, feedback, and interactivity to players, enhancing their immersion and understanding of the game.
Designing levels and environments in Unity involves carefully planning gameplay flow, balancing challenges, and creating visually captivating worlds. Through iteration, playtesting, and utilizing level design techniques, developers can create levels that guide players, provide variety, and maintain a balanced difficulty curve.
Ultimately, the process of implementing gameplay mechanics in 3D games using Unity requires a combination of technical proficiency, creativity, and player-centric design. It is an iterative process that involves constant refinement and playtesting to ensure the mechanics align with the desired player experience.
With Unity’s versatile toolset and a solid understanding of game design principles, developers can create captivating gameplay mechanics that captivate players and bring their virtual worlds to life. The possibilities are endless, and it is up to developers to unleash their creativity and design gameplay mechanics that leave a lasting impression on players.