C# (pronounced “C sharp”) plays a crucial role as the primary scripting language in Unity, one of the most popular game development engines in the industry. Unity provides a robust and flexible environment for creating games and interactive experiences across various platforms, including desktop, mobile, and virtual reality. C# serves as the scripting language of choice in Unity due to its power, versatility, and seamless integration with the engine.
- Performance: C# is a statically typed language that compiles to efficient machine code, resulting in high-performance applications. Unity leverages the Mono runtime, an open-source implementation of the .NET framework, to execute C# scripts. This combination ensures that games developed in Unity with C# achieve optimal performance, making it suitable for both small indie projects and large-scale productions.
- Ease of Use: C# is known for its readability and user-friendly syntax, making it relatively easy for developers to learn and work with. Unity’s scripting API provides extensive documentation and a rich set of features that allow developers to interact with the game engine seamlessly. Additionally, Unity’s visual editor integrates well with C#, enabling developers to design and modify game logic through an intuitive interface.
- Powerful Features: C# offers a wide range of features and libraries that empower game developers to implement complex functionality with ease. From basic control flow statements to advanced object-oriented programming techniques, C# provides a robust foundation for building games. Unity extends this power with its own API, allowing developers to access and manipulate various aspects of the game engine, such as physics, rendering, audio, and user input.
- Cross-platform Development: Unity enables developers to target multiple platforms with a single codebase, thanks to its multi-platform support. C# plays a significant role in this aspect by providing a consistent scripting language across different platforms. Developers can write platform-agnostic code in C#, and Unity takes care of adapting it to the target platform during the build process. This greatly simplifies the development workflow and allows developers to reach a broader audience with their games.
- Vast Community and Ecosystem: C# has a large and active community of developers, which is further amplified by Unity’s popularity. The Unity Asset Store offers a vast collection of pre-built assets, plugins, and tools, many of which are written in C#. This ecosystem provides developers with an extensive range of resources and solutions to enhance their games, improve productivity, and accelerate development cycles.
- Extensibility: C# in Unity is not limited to just game logic scripting. Developers can extend the Unity editor itself by writing custom editor scripts using C#. This allows for the creation of custom tools, workflows, and UI enhancements tailored to specific project requirements. The extensibility of C# in Unity empowers developers to streamline their development process and create more efficient workflows.
Overall, C# serves as the primary scripting language in Unity due to its performance, ease of use, powerful features, cross-platform capabilities, thriving community, and extensibility. The combination of Unity’s game engine and C#’s flexibility provides developers with a powerful toolset to bring their creative visions to life and deliver engaging and immersive experiences to players.
Setting up Unity for scripting
- Setting Up the Scripting Environment: Unity uses Microsoft’s Visual Studio as the default integrated development environment (IDE) for scripting in C#. You can install Visual Studio by selecting “Download Visual Studio” from the Unity Editor’s “Preferences” or “External Tools” settings. Alternatively, you can use other compatible IDEs like Visual Studio Code or JetBrains Rider, which require additional setup steps.
- Creating Scripts: To create a new script, right-click in the Unity Project panel, select “Create,” and choose “C# Script.” Give your script a name and double-click it to open it in your selected IDE. The script will open with a template that includes a starting point for writing your code.
- Scripting in C#: Begin writing your C# code within the script file. Unity’s scripting API provides a wide range of classes and functions that allow you to interact with the game engine. You can access Unity’s features, such as physics, rendering, input handling, and asset management, through the API. Refer to Unity’s scripting documentation (https://docs.unity3d.com/ScriptReference) for detailed information about available classes, functions, and their usage.
- Attaching Scripts to Game Objects: To use a script in your scene, you need to attach it to a game object. Select a game object in the Scene View or Hierarchy, and in the Inspector panel, click “Add Component” and search for the name of your script. Click on the script to attach it to the selected game object. You can then access and control the behavior of that object using the script’s code.
- Running and Testing: Once you’ve written your script, save it, and switch back to the Unity Editor. Unity automatically detects changes in your script files and recompiles them. To test your script, click the “Play” button in the Unity Editor to enter Play Mode and see how your scripts interact with the game objects and the environment. The Console panel will display any runtime errors or log messages generated by your scripts.
Remember to save your work regularly and utilize version control systems like Git to track changes and collaborate with others effectively.
By following these steps, you can set up Unity for scripting and begin developing interactive behaviors, game mechanics, and custom functionality using C# within the Unity game development environment.
Syntax and structure of C# scripts
C# scripts in Unity follow the syntax and structure of the C# programming language, with some additional Unity-specific elements. Here’s an overview of the syntax and structure of C# scripts in Unity:
- Script Naming and Extension: Unity scripts are typically saved with a .cs extension. It’s common practice to name scripts with descriptive names that reflect their purpose or functionality.
- Using Directives: C# scripts in Unity begin with using directives, which provide access to namespaces containing the classes and functions you want to use. For example, to use Unity’s built-in classes, you would typically include the following using directive at the top of your script:
using UnityEngine;
This allows you to access Unity’s classes, such as MonoBehaviour, GameObject, Transform, etc., without fully qualifying their names.
- Class Declaration: C# scripts in Unity are defined within a class. The class typically derives from MonoBehaviour, which is a base class provided by Unity for script components. Here’s an example of a basic script structure:
using UnityEngine;
public class MyScript : MonoBehaviour
{
// Script code goes here
}
In the example above, “MyScript” is the name of the script class. The “public” keyword allows the class to be accessed from other scripts and components.
- Methods: Methods are functions defined within a class and contain the script’s logic. Unity scripts commonly use the following methods:
- Start(): This method is called once, at the beginning, when the script component is enabled or when the scene starts.
- Update(): This method is called every frame and is where you place code for continuous actions or checks.
- FixedUpdate(): This method is called at a fixed interval, mainly used for physics-related calculations.
Here’s an example of a script with Start() and Update() methods:
using UnityEngine;
public class MyScript : MonoBehaviour
{
private void Start()
{
// Initialization code
}
private void Update()
{
// Update code
}
}
You can add additional methods to handle specific behaviors or events based on your requirements.
- Variables and Data Types: C# supports various data types, such as integers, floats, booleans, strings, and more. Variables in C# scripts need to be declared with a specific type before use. Here’s an example of variable declarations:
int score = 0;
float speed = 5.0f;
bool isActive = true;
string playerName = "John";
Unity-specific data types are also available for representing game objects, components, transforms, and more.
- Unity-Specific Functions: Unity provides numerous built-in functions that you can override or use within your scripts to interact with the game engine. These functions are called by the engine at specific times or events. For example:
- Awake(): Called when the script component is initialized.
- OnCollisionEnter(): Called when the game object collides with another collider.
- OnTriggerEnter(): Called when the game object enters a trigger collider.
- Unity API: The Unity API (Application Programming Interface) provides a vast set of classes and functions to interact with the Unity engine and its features, such as rendering, physics, input handling, and more. You can access and utilize the Unity API within your script to manipulate game objects, apply physics forces, play sounds, load scenes, and much more.For example, you can access the transform component of a game object and modify its position like this:
transform.position = new Vector3(0, 0, 0);
This code sets the position of the game object to the world coordinates (0, 0, 0).
C# scripts in Unity combine the power and flexibility of the C# language with Unity’s game engine capabilities. By following the syntax and structure outlined above, you can create dynamic and interactive behaviors for your games and applications within the Unity environment.
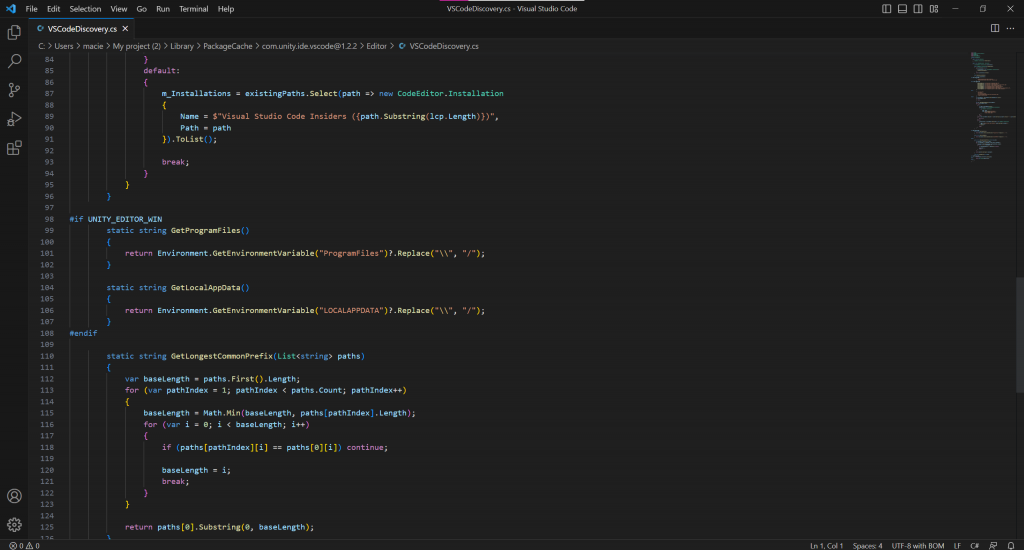
Data types, variables, and constants in C#
In C#, data types, variables, and constants are fundamental elements used to store and manipulate data within a program. Here’s an overview of data types, variables, and constants in C#:
Data Types:
C# supports various data types, each designed to hold a specific kind of data. The common data types in C# include:
- Numeric Types:
- Integral Types: byte, sbyte, short, ushort, int, uint, long, ulong
- Floating-Point Types: float, double
- Decimal Type: decimal
- Boolean Type: bool, which represents a true or false value.
- Character Type: char, used to store a single Unicode character.
- String Type: string, used to store a sequence of characters.
- Enumeration Type: enum, which defines a set of named constants.
- Structures: struct, a user-defined composite data type.
- Classes: class, a reference type that serves as a blueprint for creating objects.
- Arrays: Used to store a collection of elements of the same type.
- Nullable Types: Nullable<T>, which allows value types to have a null value.
Variables:
Variables are used to store and manipulate data within a program. In C#, variables must be declared with a specific data type before they can be used. Here’s the syntax for declaring variables:
dataType variableName;
For example, to declare an integer variable named “score,” you would write:
int score;
Variables can also be initialized at the time of declaration:
int score = 0;
Variables can be assigned new values later in the program:
score = 100;
Constants:
Constants are similar to variables, but their values cannot be changed once they are assigned. They are useful for storing values that remain constant throughout the program’s execution. In C#, constants are declared using the const
keyword:
const dataType constantName = value;
For example, to declare a constant named “Gravity” with a value of 9.8, you would write:
const float Gravity = 9.8f;
Constants must be assigned a value at the time of declaration and cannot be modified later.
Naming Conventions:
In C#, variables and constants follow certain naming conventions for readability and maintainability. Here are some common conventions:
- Variable and constant names should be meaningful and descriptive.
- Use camelCase for variable names (e.g., myVariable).
- Use PascalCase for constant and type names (e.g., MaxValue, MyClass).
- Avoid using reserved keywords as variable or constant names.
- Use plural names for collections or arrays (e.g., scores[], playersList).
- Use uppercase for constant names (e.g., PI, MAX_VALUE).
Understanding data types, variables, and constants in C# is essential for effectively working with and manipulating data within a program. By choosing appropriate data types, declaring variables, and using constants, you can store and manipulate data to achieve the desired functionality in your C# programs.
Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a programming paradigm widely used in Unity game development. OOP focuses on organizing code into reusable objects, which encapsulate data and behavior. Unity itself is built on the principles of OOP, and understanding OOP concepts is crucial for effective Unity development. Here’s an overview of how OOP is applied in Unity:
1. Classes and Objects:
In Unity, classes are the building blocks of objects. A class is a blueprint that defines the properties and behavior of an object. For example, a “Player” class may have properties like name, health, and score, as well as methods like Move() and Attack().
Objects, also called instances, are created from classes and represent individual entities within the game. For instance, you can create multiple instances of the Player class to represent different players in the game.
2. Inheritance:
Inheritance is a key concept in OOP that allows classes to inherit properties and methods from a parent class. In Unity, the MonoBehaviour class serves as the base class for scripts that are attached to game objects. By deriving from MonoBehaviour, you inherit built-in Unity functionality, such as the Update() method for frame-by-frame updates.
public class Player : MonoBehaviour
{
// Player-specific properties and methods
}
3. Encapsulation:
Encapsulation refers to the bundling of data and methods within a class to control access and ensure data integrity. In Unity, encapsulation is commonly used to hide implementation details and expose only necessary functionality. By defining properties and methods as public, private, or protected, you control how they are accessed by other classes.
public class Player : MonoBehaviour
{
private string playerName; // private property
public void SetName(string name) // public method
{
playerName = name;
}
}
4. Polymorphism:
Polymorphism allows objects of different classes to be treated as objects of a common base class. This enables flexibility and extensibility in Unity. For example, you can have multiple enemy types derived from a common Enemy base class, and treat them uniformly when writing code that handles enemy behavior.
public class Enemy : MonoBehaviour
{
public virtual void Attack()
{
// Common attack behavior for all enemies
}
}
public class BossEnemy : Enemy
{
public override void Attack()
{
// Special attack behavior for boss enemy
}
}
5. Composition:
Composition involves creating complex objects by combining simpler objects. In Unity, this is often achieved through the use of components. Game objects can be composed of various components that define their functionality, such as rendering, physics, audio, and more. Components can be added, removed, or modified to build complex behaviors and interactions.
6. Event-Driven Programming:
Unity heavily relies on event-driven programming, where actions are triggered by events. Events can be related to user input, collisions, time-based updates, and more. Unity provides event hooks, such as OnCollisionEnter(), Update(), and OnTriggerEnter(), which can be overridden in scripts to respond to specific events.
public class Player : MonoBehaviour
{
private void OnTriggerEnter(Collider other)
{
// Code to handle collision with other objects
}
}
Understanding and applying OOP concepts in Unity allows for modular, maintainable, and scalable code. It promotes code reusability, organization, and extensibility, making it easier to develop and maintain complex game projects. By leveraging OOP principles, you can design and implement flexible and interactive gameplay systems within the Unity game development environment.
Capturing input from keyboard, mouse, and touch
Capturing input from keyboard, mouse, and touch is essential for creating interactive experiences in Unity. Unity provides a convenient input system that allows you to easily handle input events from various sources. Here’s an overview of capturing input from keyboard, mouse, and touch in Unity:
1. Keyboard Input:
To capture keyboard input in Unity, you can use the Input class. The Input class provides static methods to detect key presses, releases, and continuous input. Here’s an example of capturing keyboard input:
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
// Space key was pressed
}
if (Input.GetKey(KeyCode.W))
{
// W key is being held down
}
if (Input.GetKeyUp(KeyCode.Escape))
{
// Escape key was released
}
}
In the example above, the Update() method is called every frame, allowing you to check for keyboard input continuously. You can use KeyCode enumeration values to specify the desired key to detect.
2. Mouse Input:
Unity provides methods to capture mouse input, such as button clicks, position, and movement. The Input class also handles touch input on devices that support touch. Here’s an example of capturing mouse input:
void Update()
{
if (Input.GetMouseButtonDown(0))
{
// Left mouse button was clicked
}
if (Input.GetMouseButton(1))
{
// Right mouse button is being held down
}
if (Input.GetMouseButtonUp(2))
{
// Middle mouse button was released
}
float mouseX = Input.GetAxis("Mouse X");
float mouseY = Input.GetAxis("Mouse Y");
// Use mouseX and mouseY to handle mouse movement
}
In the example above, the GetMouseButtonDown(), GetMouseButton(), and GetMouseButtonUp() methods are used to detect mouse button events. You can also use GetAxis() to capture mouse movement along the x and y axes.
3. Touch Input:
For touch devices like smartphones and tablets, Unity provides touch input handling. The Input class allows you to detect touch events and access touch properties, such as position and phase. Here’s an example of capturing touch input:
void Update()
{
if (Input.touchCount > 0)
{
Touch touch = Input.GetTouch(0);
if (touch.phase == TouchPhase.Began)
{
// Touch began
}
if (touch.phase == TouchPhase.Moved)
{
// Touch moved
}
if (touch.phase == TouchPhase.Ended)
{
// Touch ended
}
}
}
In the example above, Input.touchCount is used to check if any touches are detected. If at least one touch is detected, the GetTouch() method is used to access touch information. The TouchPhase enum is used to check the phase of the touch event, such as TouchPhase.Began, TouchPhase.Moved, and TouchPhase.Ended.
4. Input Axes and Custom Input:
Unity’s input system also supports input axes, which allow you to define and map custom input controls. Input axes provide a flexible way to handle input from various sources, such as gamepads and joysticks. You can configure input axes in Unity’s Input Manager and access them through the Input class.
void Update()
{
float horizontal = Input.GetAxis("Horizontal");
float vertical = Input.GetAxis("Vertical");
// Use horizontal and vertical for custom input handling
}
In the example above, Input.GetAxis() is used to retrieve the values of custom input axes defined in the Input Manager. The values can be used for custom input handling, such as character movement or camera control.
Capturing input from keyboard, mouse, and touch allows you to create interactive and responsive gameplay in Unity. By utilizing the Input class and its related methods, you can detect and handle input events from various input sources, enhancing the user experience of your Unity games and applications.
Working with Unity Components and Systems
In Unity, components and systems are key elements that enable the creation of complex behaviors and interactions within a game or application. Components are attached to game objects to define their functionality, while systems provide a framework for managing and updating those components. Let’s explore working with Unity components and systems:
1. Components:
Components are the building blocks of game objects in Unity. They define the behavior and properties of the objects they are attached to. Unity provides a wide range of built-in components for common functionalities like rendering, physics, audio, input, and more. Additionally, you can create custom components to extend the functionality of your game objects.
To attach a component to a game object, you can either add it through the Unity editor or attach it dynamically through scripting. In the Unity editor, you can select a game object, go to the Inspector panel, and click on the “Add Component” button to choose from the available components.
Components can be accessed and manipulated through scripts attached to the same game object or through other scripts using the GetComponent<T>() method.
// Accessing a component attached to the same game object
public class MyScript : MonoBehaviour
{
private Rigidbody rb;
private void Start()
{
rb = GetComponent<Rigidbody>();
}
private void Update()
{
// Use rb for manipulating the Rigidbody component
}
}
2. Systems:
Systems in Unity are responsible for managing and updating components. They define the rules and behaviors that govern how components interact with each other and the game environment. Unity uses various systems, such as the Transform system, Physics system, Input system, and more, to handle different aspects of the game.
Systems are automatically executed during different phases of the game loop. For example, the Update() method is called once per frame, making it suitable for handling continuous updates, while the FixedUpdate() method is used for physics-related calculations, as it is called at a fixed time step.
public class MyScript : MonoBehaviour
{
private void Update()
{
// Executed once per frame
}
private void FixedUpdate()
{
// Executed at a fixed time step
}
}
In addition to Unity’s built-in systems, you can create custom systems using Unity’s ECS (Entity Component System) architecture or leverage third-party systems and frameworks.
3. ScriptableObjects:
ScriptableObjects are a special type of asset in Unity that allow you to create reusable data containers. They are not attached to game objects like components but can be used to store and manipulate data that can be shared across multiple instances or scripts.
ScriptableObjects can be used to define custom data structures, configurations, or game settings. They can also be used as an event system, allowing components to communicate with each other without direct references.
[CreateAssetMenu(fileName = "NewConfig", menuName = "Game Configuration")]
public class GameConfig : ScriptableObject
{
public int playerHealth;
public float enemySpeed;
}
ScriptableObjects can be created through the Unity editor and referenced from other scripts. They provide a flexible and efficient way to manage and share data in Unity projects.
Working with Unity components and systems allows you to create dynamic and interactive behaviors within your games and applications. By attaching components to game objects, utilizing built-in systems, and creating custom systems and ScriptableObjects, you can build complex and engaging experiences in Unity.
Debugging and Optimization
Debugging and optimization are crucial aspects of Unity scripting to ensure the smooth and efficient performance of your game or application. Debugging helps identify and fix errors, while optimization focuses on improving performance by reducing resource usage. Let’s delve into debugging and optimization techniques in Unity scripting:
1. Debugging:
Debugging is the process of identifying and resolving errors or issues in your code. Unity provides several tools and techniques to assist with debugging:
- Logging and Console: Use the
Debug.Log()
method to print messages to the Unity console. This allows you to inspect variable values, track the flow of execution, and identify issues. - Breakpoints: Place breakpoints in your code to pause execution at specific points. This allows you to examine variable states, step through code line by line, and identify the source of errors. Breakpoints can be set within the Unity editor or using integrated development environments (IDEs) like Visual Studio.
- Exception Handling: Implement exception handling using try-catch blocks to catch and handle runtime errors. This helps prevent your game or application from crashing and provides better error handling.
- Inspector Debugging: Utilize the Inspector window in Unity to inspect and modify the values of variables attached to game objects in real-time. This is particularly useful for tweaking values and testing behavior.
- Debugging Tools: Unity provides additional debugging tools, such as the Profiler, which helps analyze performance bottlenecks and resource usage, and the Unity Remote app, which allows you to test your game on mobile devices while viewing the console output on your computer.
2. Optimization:
Optimization involves improving the performance of your game or application to ensure smooth gameplay and efficient resource usage. Here are some optimization techniques to consider:
- Avoiding Expensive Operations: Identify and minimize computationally expensive operations, such as complex calculations or repetitive tasks. Optimize algorithms and data structures to reduce processing time.
- Object Pooling: Implement object pooling to reuse game objects instead of instantiating and destroying them frequently. This reduces the overhead of memory allocation and garbage collection.
- Level of Detail (LOD): Utilize LOD systems to decrease the level of detail in distant or less important objects. This reduces rendering workload and improves performance.
- Batching: Use Unity’s batching techniques, such as static and dynamic batching, to combine multiple objects into a single draw call. This reduces CPU overhead and improves rendering performance.
- Culling: Employ frustum culling and occlusion culling techniques to prevent rendering objects that are not within the camera’s view or occluded by other objects. This reduces unnecessary rendering computations.
- Asset Optimization: Optimize assets, such as textures, models, and audio files, by reducing their size and optimizing their formats without sacrificing quality. This reduces loading times and memory usage.
- Profiling: Use Unity’s Profiler to identify performance bottlenecks, such as excessive CPU usage, high memory consumption, or frequent garbage collection. Analyze the profiler data to pinpoint areas that require optimization.
- Mobile Optimization: Pay attention to performance considerations specific to mobile platforms, such as reducing draw calls, optimizing shaders, minimizing texture size, and using efficient lighting techniques.
- Testing and Iteration: Regularly test your game or application on different devices and platforms to ensure optimal performance. Gather feedback from users and iterate on optimization techniques based on their experiences.
By effectively debugging and optimizing your Unity scripts, you can enhance the performance and user experience of your game or application. Identifying and fixing errors, reducing resource usage, and improving performance ultimately contribute to a smoother and more enjoyable gameplay experience for your players.
Conclusion
Scripting in Unity using the C# programming language is a powerful and essential aspect of game development. With C# as the primary scripting language in Unity, developers have a robust and flexible toolset to bring their creative ideas to life.
Through scripting, developers can define the behavior and functionality of game objects, create interactive gameplay systems, handle input from various sources, and implement complex game logic. C# provides a wide range of features and concepts, including data types, variables, constants, control structures, functions, classes, inheritance, encapsulation, polymorphism, and more. These features enable developers to write modular, maintainable, and extensible code.
Unity’s component-based architecture allows developers to attach scripts as components to game objects, defining their behavior and interactions. By leveraging Unity’s built-in components and systems, as well as creating custom components, developers can build complex and interactive game worlds.
Debugging and optimization are vital aspects of Unity scripting. Debugging tools and techniques help identify and resolve errors, ensuring the stability and correctness of the code. Optimization techniques focus on improving performance by minimizing resource usage, reducing bottlenecks, and enhancing the overall efficiency of the game or application.
With the combination of C# scripting, Unity’s powerful editor, and the extensive library of assets and resources, developers can create immersive and engaging experiences for various platforms, including desktop, mobile, consoles, and virtual reality.
Scripting in Unity with C# empowers developers to turn their creative visions into reality, providing the tools and capabilities necessary to build innovative and captivating games and applications. Whether you are a beginner or an experienced developer, learning and mastering Unity scripting opens up a world of possibilities in game development.