Advanced programming constructs in Roblox Studio allow developers to create more complex and sophisticated gameplay mechanics, interactions, and systems within their games. These constructs leverage the Lua scripting language and provide a powerful set of tools and techniques. Here’s an introduction to some of the advanced programming constructs in Roblox Studio:
- Functions and Modules: Functions are reusable blocks of code that perform specific tasks. They allow you to encapsulate logic and execute it multiple times throughout your scripts. Modules are files that contain functions, variables, and other Lua code, which can be loaded and used in different scripts. Functions and modules help organize code, promote code reusability, and improve overall script maintainability.
- Object-Oriented Programming (OOP): Roblox Studio supports object-oriented programming principles, allowing developers to create custom classes and objects. By utilizing OOP concepts such as inheritance, encapsulation, and polymorphism, developers can create more structured and modular code. This approach is especially useful for creating complex systems, managing game entities, and organizing code into logical components.
- Event-driven Programming: Event-driven programming is a paradigm where code execution is driven by events or triggers. In Roblox Studio, events can be used to respond to user input, changes in game state, or interactions with objects. By subscribing to and handling events, developers can create interactive and responsive gameplay experiences. Common event-driven constructs include event listeners, event firing, and event-based callbacks.
- Coroutines: Coroutines are a powerful feature in Lua that allow for cooperative multitasking. They enable the execution of multiple tasks concurrently, without blocking the main thread or freezing the game. Coroutines are useful for managing time-based processes, complex animations, and asynchronous operations. By leveraging coroutines, developers can create smoother, more responsive gameplay experiences.
- Data Structures and Algorithms: Advanced programming in Roblox Studio involves working with data structures and algorithms to efficiently manage and manipulate game data. This includes concepts such as arrays, lists, dictionaries, stacks, queues, and sorting/searching algorithms. By employing appropriate data structures and algorithms, developers can optimize game performance, handle large data sets, and implement complex gameplay mechanics.
- Error Handling and Debugging: Roblox Studio provides tools and techniques for error handling and debugging. Proper error handling ensures that your scripts gracefully handle unexpected situations and provide meaningful error messages. Debugging tools, such as the output window, breakpoints, and variable inspection, help identify and fix issues in your code, improving script functionality and stability.
By familiarizing yourself with these advanced programming constructs in Roblox Studio, you can unlock the full potential of Lua scripting and create more intricate, interactive, and optimized games. These constructs enable you to implement complex gameplay mechanics, manage game data effectively, and create dynamic and engaging experiences for your players.
Discussion of different types of data structures
In Roblox Studio, different types of data structures, such as tables, arrays, and dictionaries, play significant roles in organizing and manipulating game data. Here’s a discussion of these data structures and their applications in game development:
- Tables: Tables in Lua are versatile data structures that can store multiple values of different types. They can be used to represent arrays, dictionaries, or a combination of both. Tables in Roblox Studio are commonly employed for various purposes, including:
- Storing Player Data: Tables can be used to store and manage player-specific information, such as player scores, inventory items, or game progress.
- Managing Game Levels: Tables can represent level layouts, with each entry storing information about the environment, objects, or obstacles present in a particular level.
- Creating Data Structures: Tables can be used to create more complex data structures, such as linked lists, stacks, or queues, by organizing data in a specific manner.
- Arrays: Arrays are a specific type of table in which values are stored in sequential, numerical indices. They are useful for storing and accessing a collection of similar data elements. In Roblox Studio, arrays find applications in:
- Storing Game Objects: Arrays can be used to store references to game objects like NPCs, enemies, or collectible items, allowing for efficient access and manipulation of these objects.
- Animation Sequences: Arrays can hold a series of animation frames or sequences, enabling smooth transitions between different animation states.
- Managing Game Grids or Maps: Arrays can represent grids or maps, allowing developers to access and update specific locations or tiles efficiently.
- Dictionaries: Dictionaries, also known as associative arrays or key-value pairs, store values with unique keys for efficient retrieval. In Roblox Studio, dictionaries are commonly used for:
- Storing Configuration Data: Dictionaries can hold configuration settings, such as game options or customizable player settings, using specific keys to access and modify the values.
- Language Localization: Dictionaries can store translations for different languages, with keys representing the phrases or words and values containing the translated versions.
- Managing Game Data: Dictionaries are useful for organizing game data, such as item attributes, enemy attributes, or level-specific information, allowing quick access to the relevant data using specific keys.
By leveraging these data structures in Roblox Studio, developers can efficiently manage and manipulate game data, improve performance, and implement complex gameplay mechanics. It’s important to choose the appropriate data structure based on the specific requirements of your game to ensure efficient storage, retrieval, and manipulation of data.
Exploring advanced scripting techniques, such as coroutines and state machines, to manage complex game logic
Exploring advanced scripting techniques, such as coroutines and state machines, can greatly assist in managing complex game logic in Roblox Studio. These techniques provide efficient ways to handle asynchronous operations, control game flow, and create dynamic gameplay experiences. Let’s delve into the details of coroutines and state machines:
- Coroutines: Coroutines are a powerful feature in Lua that allow for cooperative multitasking. They enable the execution of multiple tasks concurrently without blocking the main thread or freezing the game. Coroutines are useful for managing time-based processes, complex animations, and asynchronous operations. Some applications of coroutines in Roblox Studio include:
- Smooth Animations: Coroutines can be used to create complex animations that involve sequential or simultaneous movements, easing transitions between different animation states.
- Timed Actions: Coroutines can handle time-based actions, such as delayed effects, countdowns, or timed events, allowing for more precise control over gameplay elements.
- Asynchronous Operations: Coroutines can manage time-consuming tasks that don’t need to be executed all at once, such as loading large game assets or performing server requests.
By utilizing coroutines effectively, developers can achieve smoother gameplay, more responsive interactions, and efficient management of time-based processes in their games.
- State Machines: State machines are programming constructs that represent different states and transitions between them. They are particularly useful for managing complex game logic, character behaviors, and game system interactions. In Roblox Studio, state machines provide a structured approach to handle game states and transitions. Some applications of state machines in Roblox Studio include:
- Character AI and Behaviors: State machines can control character behaviors, allowing for different states such as idle, walking, running, attacking, or interacting with objects. Transitions between states occur based on specific conditions or triggers.
- Game System Management: State machines can manage game systems, such as menus, quests, or game modes. They help control the flow and transitions between different states, ensuring smooth and intuitive user experiences.
- Interactive Objects: State machines can be used to define the behavior of interactive objects, such as doors, switches, or puzzles. Different states represent the various interactions or states of the object, while transitions determine how the object responds to user input or triggers.
Implementing state machines in Roblox Studio helps maintain a structured and modular approach to game logic, making it easier to manage complex interactions and behaviors.
By utilizing coroutines and state machines in Roblox Studio, developers gain powerful tools for managing complex game logic. These advanced scripting techniques enable the creation of dynamic and engaging gameplay experiences, with smoother animations, responsive interactions, and efficient handling of asynchronous operations. Leveraging these techniques can lead to more polished and immersive games in Roblox Studio.
Introduction to classes and object-oriented programming (OOP) concepts
Classes and object-oriented programming (OOP) are fundamental concepts in software development, including game development in Roblox Studio. They provide a structured and modular approach to organizing code and modeling real-world entities within a game. Here’s an introduction to classes and OOP concepts in Roblox Studio:
- Classes: In Roblox Studio, a class is a blueprint or template for creating objects. It defines the properties (attributes) and behaviors (methods) that objects of that class will possess. A class serves as a blueprint from which you can create multiple instances, each with its own unique data and functionality. Classes in Roblox Studio allow for code reusability, encapsulation, and modularity.
- Objects: Objects are instances created from a class. They represent specific entities or elements in the game world. Objects have their own set of properties and behaviors, which are defined by the class they belong to. In Roblox Studio, objects can be game characters, items, NPCs, or any other interactive or non-interactive elements in the game.
- Encapsulation: Encapsulation is the concept of bundling data and related behaviors within an object, hiding the internal implementation details from external entities. In Roblox Studio, encapsulation helps in organizing code and creating objects that have well-defined interfaces for accessing and modifying their data. It promotes code modularity and enhances code readability and maintainability.
- Inheritance: Inheritance is a principle that allows classes to inherit properties and behaviors from other classes. It facilitates code reuse and promotes a hierarchical structure among classes. In Roblox Studio, inheritance is used to create specialized classes (derived classes) that inherit attributes and methods from a base class. This enables the sharing of common functionality while allowing customization and specialization in derived classes.
- Polymorphism: Polymorphism is the ability of objects to take on different forms or exhibit different behaviors based on the context. In Roblox Studio, polymorphism allows objects of different classes to respond differently to the same method call. This flexibility enables the creation of dynamic and adaptable game systems, such as character interactions, event handling, or object behaviors.
By utilizing classes and object-oriented programming concepts in Roblox Studio, developers can create more organized, modular, and scalable code. It allows for better code reuse, promotes efficient game development practices, and enhances the overall structure and maintainability of the project. OOP concepts provide a powerful paradigm for modeling game entities and interactions, facilitating the creation of complex and interactive game experiences.
How to use classes and object-oriented programming?
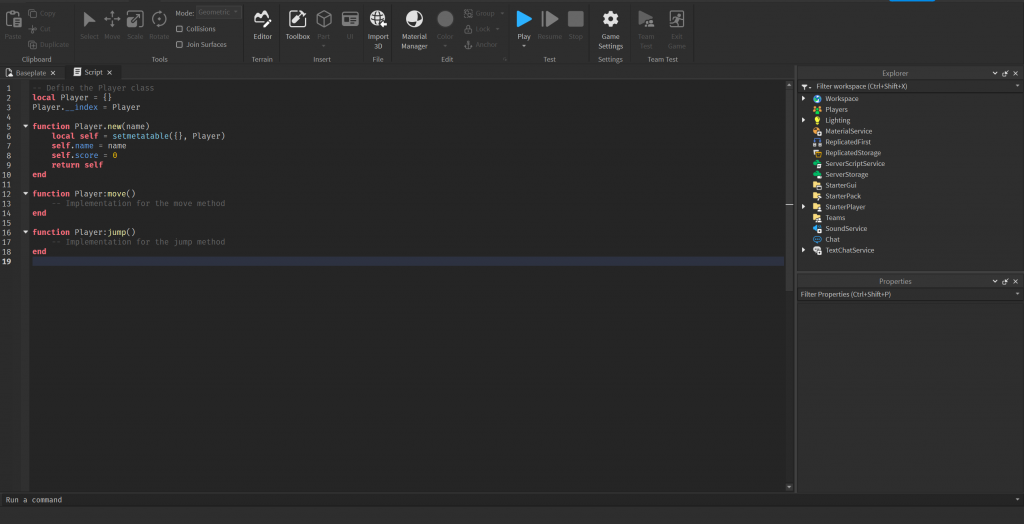
To use classes and object-oriented programming (OOP) in Roblox Studio, follow these steps:
- Define a Class: Start by defining a class that represents the blueprint for creating objects. A class includes attributes (properties) and behaviors (methods) that define the characteristics and functionality of the objects. For example, you might create a class called “Player” that has properties like “name” and “score,” as well as methods like “move” and “jump.”
-- Define the Player class
local Player = {}
Player.__index = Player
function Player.new(name)
local self = setmetatable({}, Player)
self.name = name
self.score = 0
return self
end
function Player:move()
-- Implementation for the move method
end
function Player:jump()
-- Implementation for the jump method
end
- Create Objects from the Class: Instantiate objects from the defined class using the
new
method. Each object will have its own set of properties and can perform actions using the defined methods. For example:
-- Create two Player objects
local player1 = Player.new("John")
local player2 = Player.new("Jane")
- Access Properties and Call Methods: Access the properties and call methods of the created objects using the dot notation. For example:
-- Access and modify properties
player1.score = 10
print(player2.name) -- Output: "Jane"
-- Call methods
player1:move()
player2:jump()
- Inheritance: To implement inheritance, create a base class and derived classes that inherit from the base class. The derived classes can have additional properties and behaviors while inheriting the ones defined in the base class. For example:
-- Define a base class
local Animal = {}
Animal.__index = Animal
function Animal.new()
local self = setmetatable({}, Animal)
return self
end
function Animal:sound()
-- Implementation for the sound method
end
-- Define a derived class
local Dog = setmetatable({}, { __index = Animal })
function Dog.new()
local self = setmetatable({}, Dog)
return self
end
function Dog:fetch()
-- Implementation for the fetch method
end
Now, you can create objects from the derived class and access both the methods defined in the base class and the derived class:
-- Create a Dog object
local dog = Dog.new()
-- Call methods from the base class
dog:sound()
-- Call methods from the derived class
dog:fetch()
By utilizing classes and object-oriented programming concepts in Roblox Studio, you can create structured and modular code, improve code reuse, and create more dynamic and interactive game experiences.
Discussing the implementation of classes to create objects with properties and methods for encapsulation and code reusability
Implementing classes in Roblox Studio allows for the creation of objects with properties and methods, promoting encapsulation and code reusability. Here’s a discussion of how classes can be implemented to achieve these goals:
- Encapsulation: Encapsulation refers to bundling data and related behaviors within an object, hiding the internal implementation details from external entities. In Roblox Studio, classes enable encapsulation by defining properties and methods that are accessible only within the class or through designated interfaces.
- Properties: Properties represent the data or state of an object. They can be defined within the class and accessed or modified using getter and setter methods, ensuring controlled access to the data. Encapsulating properties helps maintain data integrity and prevents direct manipulation from outside the class.
- Methods: Methods represent the behaviors or actions that an object can perform. They are defined within the class and can access and modify the object’s properties. Encapsulating methods allows for better organization and encapsulation of functionality, keeping related behaviors within the class and promoting modular code design.
By encapsulating properties and methods within a class, you establish a clear boundary for the object’s data and behavior, allowing for better control and abstraction of game elements.
- Code Reusability: One of the advantages of using classes in Roblox Studio is the ability to achieve code reusability. With classes, you can define a blueprint or template for creating multiple objects with similar properties and behaviors. By creating multiple instances of a class, each object retains its own data while sharing the same functionality.
- Object Creation: Objects are instances created from a class, and each object has its own set of properties and can invoke the methods defined in the class. By creating objects from a class, you can reuse the defined properties and methods for multiple game entities or elements.
- Inheritance: Inheritance is another powerful feature of classes that facilitates code reuse. It allows you to create specialized classes (derived classes) that inherit properties and methods from a base class. This mechanism promotes code reuse by sharing common functionality defined in the base class and allowing customization and specialization in derived classes.
By designing classes with encapsulated properties and methods, you create reusable components that can be instantiated and customized for different game entities, leading to more efficient development and maintenance of the game.
In conclusion, implementing classes in Roblox Studio provides a structured approach to create objects with encapsulated properties and methods, promoting code reusability and modularity. Encapsulation ensures controlled access to object data, while code reusability allows for the efficient reuse of code across multiple game elements. By leveraging classes effectively, developers can create organized, maintainable, and scalable game projects in Roblox Studio.
Discussing techniques for inheritance, polymorphism, and encapsulation to leverage the benefits of object-oriented programming (OOP) in game development
- Inheritance: Inheritance is a powerful concept in OOP that allows you to create specialized classes (derived classes) based on an existing class (base class). The derived class inherits properties and methods from the base class, enabling code reuse and promoting a hierarchical structure among classes.
In game development, inheritance can be used to model relationships between game entities. For example, you could have a base class called “Character” that defines common properties and behaviors shared by all characters in the game. You can then create derived classes like “PlayerCharacter” and “EnemyCharacter,” which inherit from the base class and add additional properties and behaviors specific to players and enemies, respectively. This approach helps to reuse code and maintain a consistent structure across different types of characters.
- Polymorphism: Polymorphism is the ability of objects to take on different forms or exhibit different behaviors based on their context. In OOP, polymorphism allows objects of different classes to be treated as objects of a common base class, enabling flexibility and dynamic behavior.
In game development, polymorphism can be useful for handling interactions and behaviors. For example, you could have a base class called “Interactable” that defines a method called “Interact.” Derived classes like “Button” and “NPC” can implement their own versions of the “Interact” method. When interacting with game objects, you can treat them uniformly as instances of the “Interactable” class and invoke the “Interact” method, which will exhibit different behaviors based on the specific class of the object. This approach enables flexible interactions and supports extensibility in the game.
- Encapsulation: Encapsulation involves bundling data and related behaviors within an object, hiding the internal implementation details from external entities. It promotes modularity, code organization, and data integrity.
In game development, encapsulation plays a crucial role in managing game entities and their interactions. By encapsulating properties and methods within classes, you establish clear boundaries and prevent direct manipulation of data from outside the class. This helps to maintain data integrity, prevent unwanted modifications, and provide controlled access to the object’s functionality. Encapsulation also allows for easier code maintenance and refactoring since changes can be localized to the class where the data and behavior are encapsulated.
By leveraging techniques such as inheritance, polymorphism, and encapsulation in game development, you can create modular, extensible, and maintainable code. Inheritance enables code reuse and promotes a hierarchical structure among classes, polymorphism allows for flexible interactions and dynamic behavior, and encapsulation ensures data integrity and controlled access to object functionality. These techniques collectively enhance the power and flexibility of OOP in developing complex and interactive games.
Conclusion
Advanced programming constructs in Roblox Studio offer powerful tools and techniques to enhance game development. By leveraging concepts such as data structures, classes, object-oriented programming (OOP), and advanced scripting techniques, developers can create more sophisticated and immersive experiences.
Data structures like tables, arrays, and dictionaries enable efficient storage and manipulation of data, allowing for organized and optimized game logic. They provide flexibility in handling complex data relationships, managing collections of objects, and implementing algorithms.
Classes and OOP concepts bring modularity, encapsulation, and code reusability to Roblox Studio. They allow for the creation of objects with properties and methods, promoting structured and organized code. Inheritance and polymorphism enable the modeling of relationships between classes, facilitating code reuse and dynamic behaviors.
Advanced scripting techniques like coroutines and state machines help manage complex game logic and asynchronous tasks. Coroutines allow for concurrent execution and efficient resource utilization, while state machines offer a structured approach to manage different states and behaviors in the game.
Additionally, integrating animations and sounds using Roblox Studio’s animation editor and sound uploading capabilities enriches the immersive experience of games. Animations bring characters and objects to life, while sounds add realism and interactivity to the game world.
By understanding and applying these advanced programming constructs, developers can create robust, scalable, and engaging games in Roblox Studio. These constructs provide the tools to manage complexity, organize code, enhance interactivity, and deliver unique experiences to players. As game development continues to evolve, mastering these advanced techniques becomes increasingly valuable for creating high-quality games in Roblox Studio.