This Lua script is designed to manage a simple game scenario where the player has health and a score. The script defines initial values for player health and score, then continuously updates these values in a loop, simulating actions like taking damage and earning points.
Let’s break down the script:
Variable Definitions:
local playerHealth = 100 -- Initial health value local playerScore = 0 -- Initial score value
playerHealth
is initialized with a value of 100, representing the player’s initial health.playerScore
is initialized with a value of 0, representing the player’s initial score.
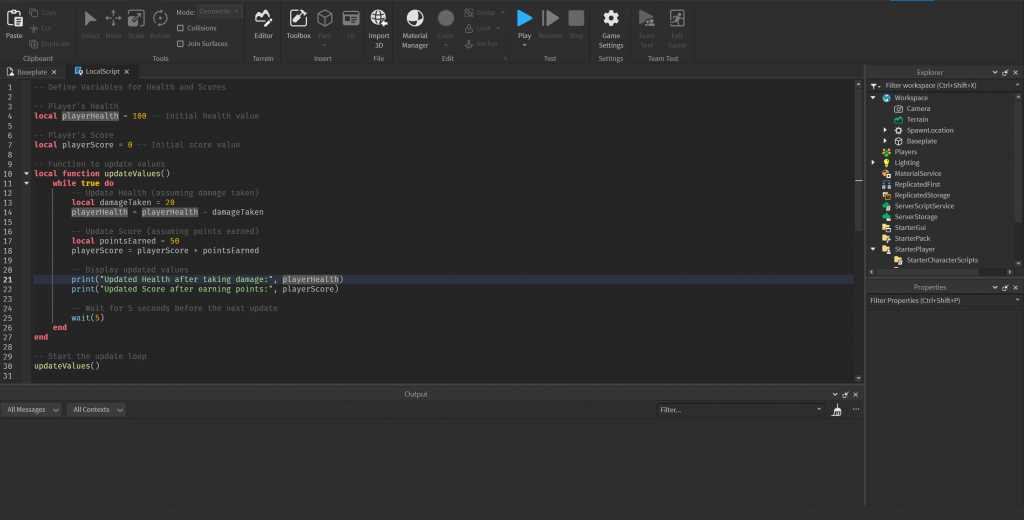
Update Function:
local function updateValues() while true do -- Update Health (assuming damage taken) local damageTaken = 20 playerHealth = playerHealth - damageTaken -- Update Score (assuming points earned) local pointsEarned = 50 playerScore = playerScore + pointsEarned -- Display updated values print("Updated Health after taking damage:", playerHealth) print("Updated Score after earning points:", playerScore) -- Wait for 5 seconds before the next update wait(5) end end
updateValues
is a function that continuously runs in an infinite loop (while true
).- It simulates the player taking damage by subtracting 20 from
playerHealth
. - It simulates the player earning points by adding 50 to
playerScore
. - It prints the updated health and score values to the console.
- It then waits for 5 seconds before the next iteration.
Start the Loop:
updateValues()
- This line initiates the update loop by calling the
updateValues
function.
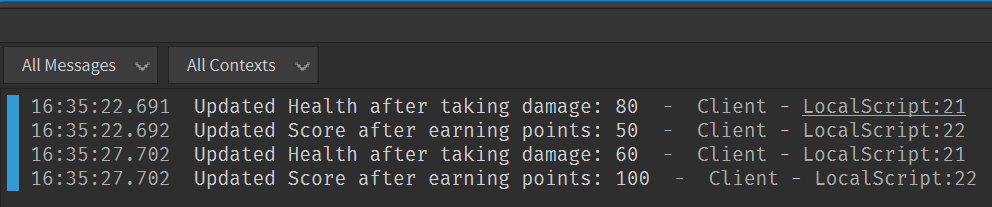
Keep in mind that this script is a simplified example and does not interact with a game environment. It’s more of a demonstration of a basic structure for managing player health and score in a game loop. In a real game, you would likely have more sophisticated mechanisms for handling player actions, events, and interactions with the game world.
Introduction to Leaderstats
In the dynamic world of Roblox game development, effective management and tracking of player data are crucial for creating engaging and immersive experiences. One essential feature that facilitates this is the implementation of Leaderstats. Leaderstats is a mechanism within Roblox games that enables developers to organize and manage player-specific data efficiently.
Leaderstats essentially function as a set of properties associated with each player, allowing developers to keep track of various in-game metrics such as scores, currency, and other custom statistics. This information is often displayed in a leaderboard, providing players with a sense of progression and comparison within the game.
Why leaderstats are better than using local variables?
Using Leaderstats in Roblox offers several advantages over relying solely on local variables. Here are some key reasons why the Leaderstats approach is often considered better:
- Persistence:
- Leaderstats data is stored on the server, providing persistence across player sessions. This ensures that player progress, scores, and other important data are retained even if players leave the game and return later. Local variables, being client-side, do not offer this level of persistence.
- Security:
- Leaderstats data is less susceptible to exploitation or cheating by players. Since the information is stored on the server, it is more challenging for players to manipulate or modify their own data to gain an unfair advantage. Local variables, being accessible on the client side, are more vulnerable to exploitation.
- Global Accessibility:
- Leaderstats data is easily accessible across different scripts and game components. This global accessibility simplifies the implementation of features such as leaderboards, achievements, and quests. Local variables are limited to the scope of the script or function where they are defined, making them less suitable for widespread data management.
- Data Synchronization:
- Leaderstats automatically handles the synchronization of data between the server and clients. This ensures that all players receive consistent and up-to-date information about their own progress and the progress of others. Local variables do not offer this built-in synchronization, requiring developers to implement their own syncing mechanisms.
- Scalability:
- Leaderstats provide a scalable solution for managing player data in more complex games. As the game grows in complexity and features, the centralized nature of Leaderstats makes it easier to manage and scale the storage and retrieval of player-specific information. Local variables may become unwieldy as the game expands, leading to potential issues with organization and scalability.
- Game Integrity:
- Leaderstats contribute to maintaining the integrity of the game by preventing players from easily manipulating their own data. This ensures a fair and consistent experience for all players, contributing to a more enjoyable and competitive gaming environment. Local variables lack the server-side control necessary for maintaining this level of integrity.
Leaderstats approach in Roblox is preferable to using local variables due to its advantages in terms of persistence, security, global accessibility, data synchronization, scalability, and game integrity. These factors collectively contribute to a more robust and reliable system for managing player-specific data in the dynamic world of Roblox game development.
In Roblox, saving player data into Leaderstats involves using a player’s data store. Here’s an example script that demonstrates how to save playerHealth
and playerScore
as local variables into the player’s Leaderstats:
-- Function to save player data to Leaderstats
local function saveDataToLeaderstats(player)
-- Check if the player has a leaderstats folder, create one if not
local leaderstatsFolder = player:FindFirstChild("leaderstats")
if not leaderstatsFolder then
leaderstatsFolder = Instance.new("Folder")
leaderstatsFolder.Name = "leaderstats"
leaderstatsFolder.Parent = player
end
-- Create or get the playerHealth and playerScore variables
local playerHealth = player:FindFirstChild("playerHealth") or Instance.new("IntValue")
playerHealth.Name = "playerHealth"
playerHealth.Parent = leaderstatsFolder
local playerScore = player:FindFirstChild("playerScore") or Instance.new("IntValue")
playerScore.Name = "playerScore"
playerScore.Parent = leaderstatsFolder
-- Save the local variables into Leaderstats
playerHealth.Value = 100 -- Set playerHealth to an initial value
playerScore.Value = 0 -- Set playerScore to an initial value
-- Connect a function to save the variables when they change
playerHealth.Changed:Connect(function()
player:SetAttribute("PlayerHealth", playerHealth.Value)
end)
playerScore.Changed:Connect(function()
player:SetAttribute("PlayerScore", playerScore.Value)
end)
end
-- Function to load player data from Leaderstats
local function loadDataFromLeaderstats(player)
local leaderstatsFolder = player:FindFirstChild("leaderstats")
if leaderstatsFolder then
local playerHealth = leaderstatsFolder:FindFirstChild("playerHealth")
local playerScore = leaderstatsFolder:FindFirstChild("playerScore")
if playerHealth and playerScore then
-- Load the saved values into local variables
playerHealth.Value = player:GetAttribute("PlayerHealth") or 100
playerScore.Value = player:GetAttribute("PlayerScore") or 0
end
end
end
-- Connect the functions to player events
game.Players.PlayerAdded:Connect(function(player)
saveDataToLeaderstats(player)
loadDataFromLeaderstats(player)
end)
-- Optional: Save data when player leaves the game
game.Players.PlayerRemoving:Connect(function(player)
player:SetAttribute("PlayerHealth", nil)
player:SetAttribute("PlayerScore", nil)
end)
This script creates and updates two local variables (playerHealth
and playerScore
) within a player’s Leaderstats. The data is stored and retrieved using the player’s attributes. Remember to modify this script according to your game’s specific needs and integrate it into your existing game structure.
Conclusion
Provided Lua script demonstrates a basic framework for managing a player’s health and score in a simulated game environment. The script employs a continuous update loop, executed by the updateValues
function, which simulates the player taking damage and earning points. The script showcases the use of variables (playerHealth
and playerScore
) to keep track of the player’s status in the game.
However, it’s important to note that this script serves as a simplified example and lacks interaction with an actual game environment. In a real gaming scenario, additional complexities and interactions would be necessary, such as user input handling, game events, and more sophisticated mechanisms for updating health and score.
Additionally, the wait(5)
function is used to introduce a delay of 5 seconds between each iteration of the loop, providing a sense of time progression within the game. This delay is crucial for controlling the frequency of updates and ensuring a reasonable pace of events in the game.
In a practical application, this script would be part of a larger codebase, integrated with game mechanics and possibly linked to user inputs, events, or other game systems. The simplicity of this script makes it a foundation for more advanced game development where health and score management are critical components.