The purpose of the script is to create a “leaderstats” folder for each player and a corresponding “Money” IntValue inside that folder, initializing the money value to 0. Here’s a step-by-step explanation of the script:
Inside the Explorer window, locate “ServerScriptService” and add a new script.
Function Definition:
local function onPlayerAdded(player)
This line declares a local function named onPlayerAdded
that takes a player
parameter. This function will be executed whenever a new player joins the game.
Creating Leaderstats Folder:
local leaderstats = Instance.new("Folder")
leaderstats.Name = "leaderstats"
Here, a new Folder
instance is created, named “leaderstats”. This folder will be used to store various statistics or values for the player.
Creating Money IntValue:
local money = Instance.new("IntValue")
money.Name = "Money"
money.Value = 0
Another instance is created, this time an IntValue
named “Money”. This value will represent the amount of money a player has, and it is initialized to 0.
Parenting:
leaderstats.Parent = player
money.Parent = leaderstats
The leaderstats
folder is set as a child of the player
instance, effectively attaching it to the player in the game. Similarly, the money
IntValue is made a child of the leaderstats
folder.
Connection to PlayerAdded Event:
game.Players.PlayerAdded:Connect(onPlayerAdded)
This line connects the onPlayerAdded
function to the PlayerAdded
event of the Players
service in the game
object. This ensures that the onPlayerAdded
function will be called whenever a new player joins the game.
In summary, this script creates a structure for storing player statistics by creating a “leaderstats” folder for each player and initializing a “Money” value inside it. The onPlayerAdded
function is connected to the event that triggers whenever a player joins the game, ensuring that this structure is set up for every new player.
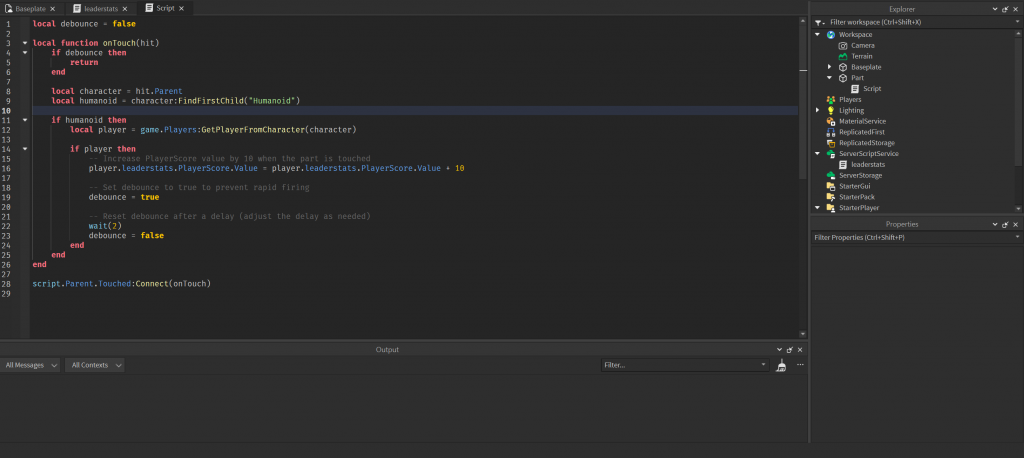
Full Server Script (ServerScriptService)
local function onPlayerAdded(player)
-- Create leaderstats for the player
local leaderstats = Instance.new("Folder")
leaderstats.Name = "leaderstats"
local money = Instance.new("IntValue")
money.Name = "Money"
money.Value = 0
leaderstats.Parent = player
money.Parent = leaderstats
end
game.Players.PlayerAdded:Connect(onPlayerAdded)
Part Script (Attach to any part in the game)

This script appears to be an enhanced version of the previous script with the addition of a debounce
mechanism to prevent rapid firing. Here’s a breakdown of the script:
This is a script with the addition of a debounce
mechanism to prevent rapid firing. Here’s a breakdown of the script:
Debounce Initialization:
local debounce = false
This line initializes a local variable debounce
and sets it to false. This variable will be used to control whether the script should respond to additional touch events or not.
Function Definition:
local function onTouch(hit)
This line declares a local function named onTouch
that takes a parameter hit
. The hit
parameter represents the object that was touched.
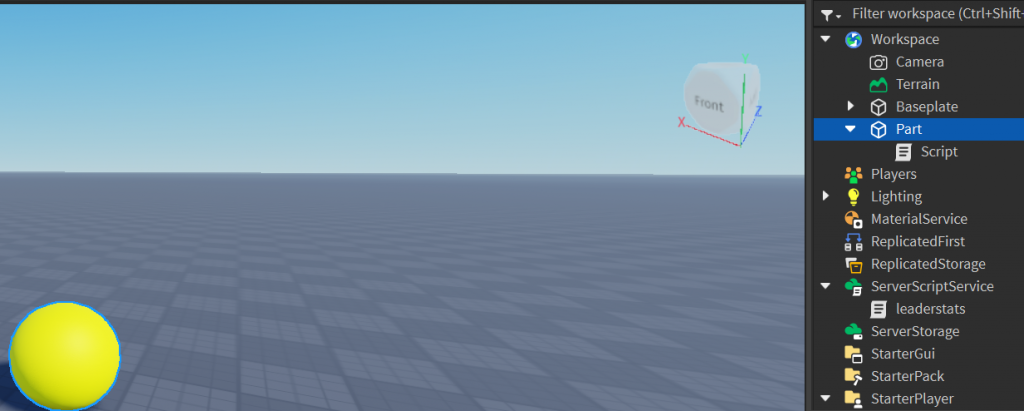
Debounce Check:
if debounce then return end
This conditional statement checks if debounce
is true. If it is, the function immediately returns without further execution. This prevents the script from responding to touch events if the debounce
flag is already set, effectively creating a cooldown period.
Identifying the Character and Humanoid:
local character = hit.Parent local humanoid = character:FindFirstChild("Humanoid")
These lines get the parent of the touched object (character
) and attempt to find a child named “Humanoid” within that character. This is a common structure in Roblox games where the player’s character is represented by a model that contains a humanoid part.
Checking for Humanoid Existence:
if humanoid then
This conditional statement checks if a humanoid part was found within the character. If a humanoid is present, it means that the touched object is part of a player’s character.
Getting Player from Character:
local player = game.Players:GetPlayerFromCharacter(character)
This line attempts to get the player associated with the character. It uses the GetPlayerFromCharacter
method provided by the Players
service in the game
object.
Checking Player Existence:
if player then
This conditional statement checks if a player is associated with the character. If a player is found, it means that the touched object is part of a player’s character, and the player is in the game.
Increasing PlayerScore Value and Debouncing:
player.leaderstats.PlayerScore.Value = player.leaderstats.PlayerScore.Value + 10 debounce = true wait(2) debounce = false
If a player is found, this block of code increases the “PlayerScore” value in the player’s leaderstats
folder by 10, sets the debounce
flag to true to prevent rapid firing, waits for 2 seconds using the wait(2)
function, and then resets the debounce
flag to false. This entire sequence ensures that there is a cooldown period between consecutive touch events.
In summary, this script enhances the previous script by adding a debounce mechanism, preventing the script from responding to touch events too rapidly. The debounce
flag is used to control this behavior, creating a brief cooldown period after each touch event.
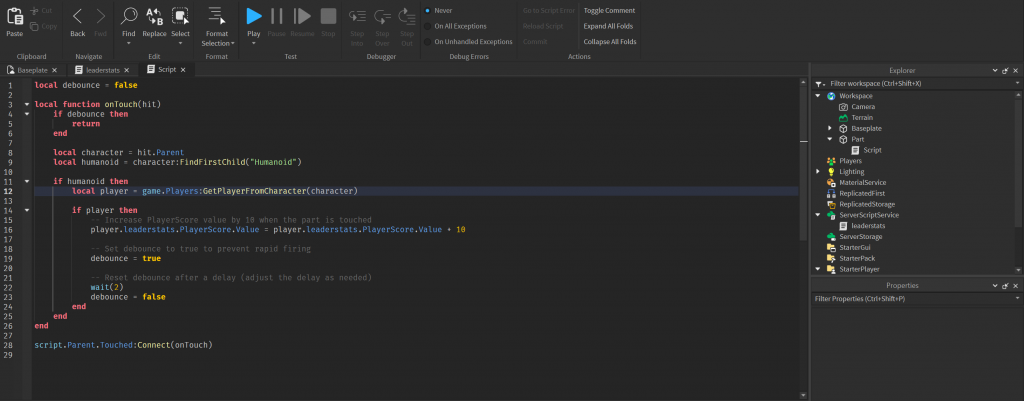
Full Part Script:
local debounce = false
local function onTouch(hit)
if debounce then
return
end
local character = hit.Parent
local humanoid = character:FindFirstChild("Humanoid")
if humanoid then
local player = game.Players:GetPlayerFromCharacter(character)
if player then
-- Increase PlayerScore value by 10 when the part is touched
player.leaderstats.PlayerScore.Value = player.leaderstats.PlayerScore.Value + 10
-- Set debounce to true to prevent rapid firing
debounce = true
-- Reset debounce after a delay (adjust the delay as needed)
wait(2)
debounce = false
end
end
end
script.Parent.Touched:Connect(onTouch)
Conclusion
provided scripts showcase a system in Roblox Studio where a player’s “PlayerScore” value is increased when a specific part is touched. The scripts implement key features such as the creation of a “leaderstats” folder for each player and the utilization of a debounce mechanism to prevent rapid firing or continuous execution of the script.
The first script establishes the foundation by creating the necessary player statistics structure upon joining the game. It ensures that each player has a “PlayerScore” value initialized to 0 within their “leaderstats” folder.
The second script, an extension of the first, introduces a touch event mechanism. When a player’s character touches a specific part, the script increments the player’s “PlayerScore” value by 10. The addition of a debounce mechanism prevents immediate and continuous execution, introducing a brief cooldown period between touch events.
Together, these scripts provide a basis for developing interactive game mechanics in Roblox Studio, allowing developers to track and modify player statistics based on in-game interactions. The debounce mechanism enhances the user experience by preventing unintended or rapid triggering of events, contributing to a more controlled and enjoyable gameplay experience.