Obtaining an API Key from Alpha Vantage for Stock Price Data
Alpha Vantage is a financial data provider that offers APIs for accessing various financial data, including real-time and historical stock prices. To use their services, you need to obtain an API key. Here’s a step-by-step guide on how to get an API key from Alpha Vantage:
Step 1: Visit Alpha Vantage Website
Go to the Alpha Vantage official website: https://www.alphavantage.co/
Step 2: Sign Up for an Account
- If you already have an account, log in. If not, click on the “Sign Up” button to create a new account.
- Fill in the required information to register for an account.
Step 3: Log In
Once you’ve signed up or logged in, you’ll be directed to the Alpha Vantage dashboard.
Step 4: Generate API Key
- On the Alpha Vantage dashboard, locate the “Get your free API key” section.
- Click on the “Get your free API key” link.
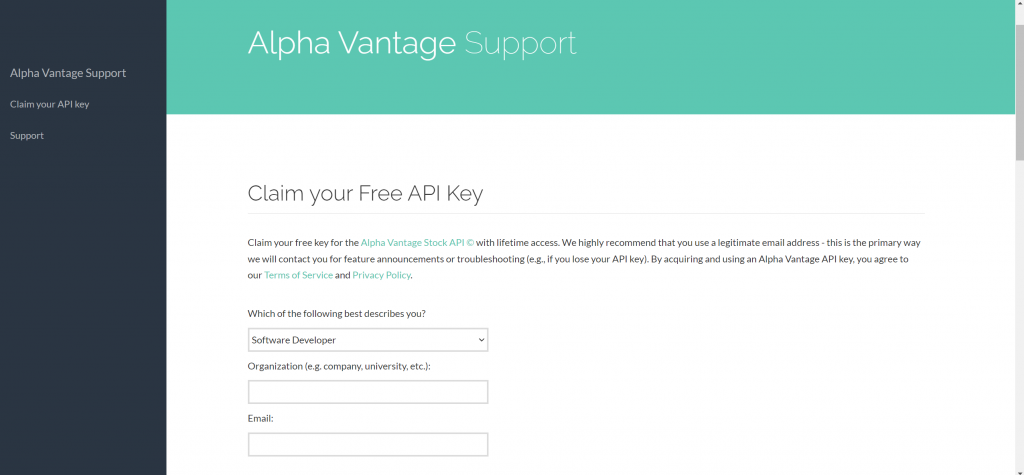
Server Script
The provided script is a basic example of using Roblox Studio to fetch real-time stock prices for a given symbol (in this case, “RBLX”) using the Alpha Vantage API. The fetched data is then sent to all clients through a RemoteEvent named “Getprice” stored in ReplicatedStorage. Here’s a step-by-step overview:
Import Necessary Services:
- Import the
HttpService
module to make HTTP requests.
local HttpService = game:GetService("HttpService")
Set Up API Key and Symbol:
- Replace
"your_api_code"
with your actual Alpha Vantage API key. - Set the
symbol
variable to the desired stock symbol (e.g., “RBLX”).
local apiKey = "your_api_code"
local symbol = "RBLX" -- Symbol for Roblox company
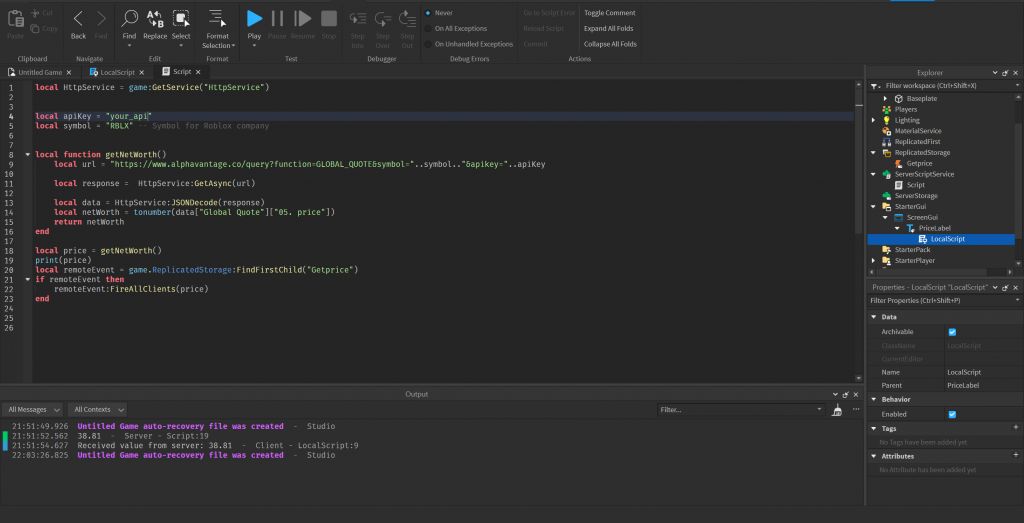
Define Function to Get Net Worth:
- Create a function named
getNetWorth()
that constructs the API URL, makes a request, and parses the JSON response to get the net worth.
local function getNetWorth()
local url = "https://www.alphavantage.co/query?function=GLOBAL_QUOTE&symbol="..symbol.."&apikey="..apiKey
local response = HttpService:GetAsync(url)
local data = HttpService:JSONDecode(response)
local netWorth = tonumber(data["Global Quote"]["05. price"])
return netWorth
end
Get Net Worth and Fire RemoteEvent:
- Call the
getNetWorth()
function to obtain the stock price. - Find the RemoteEvent named “Getprice” in
ReplicatedStorage
. - If found, fire the RemoteEvent to all clients with the fetched price.
local price = getNetWorth()
local remoteEvent = game.ReplicatedStorage:FindFirstChild("Getprice")
if remoteEvent then
remoteEvent:FireAllClients(price)
end
Full Server Script
local HttpService = game:GetService("HttpService")
local apiKey = "your_api_key"
local symbol = "RBLX" -- Symbol for Roblox company
local function getNetWorth()
local url = "https://www.alphavantage.co/query?function=GLOBAL_QUOTE&symbol="..symbol.."&apikey="..apiKey
local response = HttpService:GetAsync(url)
local data = HttpService:JSONDecode(response)
local netWorth = tonumber(data["Global Quote"]["05. price"])
return netWorth
end
local price = getNetWorth()
print(price)
local remoteEvent = game.ReplicatedStorage:FindFirstChild("Getprice")
if remoteEvent then
remoteEvent:FireAllClients(price)
end
Remember to ensure that the RemoteEvent is properly set up on the client side to receive and handle the price data. Additionally, consider implementing error handling for the HTTP requests and data parsing to enhance the script’s robustness.
How to Add Remote Event?
- Create a RemoteEvent:
- In the “Explorer” window, locate “ReplicatedStorage.”
- Right-click on “ReplicatedStorage” and choose “Insert Object.”
- Choose “RemoteEvent” from the list.
- Rename the RemoteEvent:
- Right-click on the newly created RemoteEvent in the “Explorer.”
- Choose “Rename” and give it a name “Getprice”
- Accessing the RemoteEvent:
- To access the RemoteEvent in a script, you’ll typically use
game.ReplicatedStorage
to get to ReplicatedStorage and then access your RemoteEvent.
- To access the RemoteEvent in a script, you’ll typically use

How To Enable HTTP Requests and Access to API Services?
To enable HTTP requests in Roblox Studio, you can follow these steps:
- Open the game you wish to enable HTTP requests for.
- Click on the
Home
tab in the top menu. - Click on
Game Settings
. - In the window that opens, click on the
Security
tab. - Under the
Security
tab, find theEnable HTTP Requests
option and check the box next to it. - Click
Save
.
After enabling HTTP requests, you can use the HttpService
in your scripts. Here’s a basic example of how to get HttpService
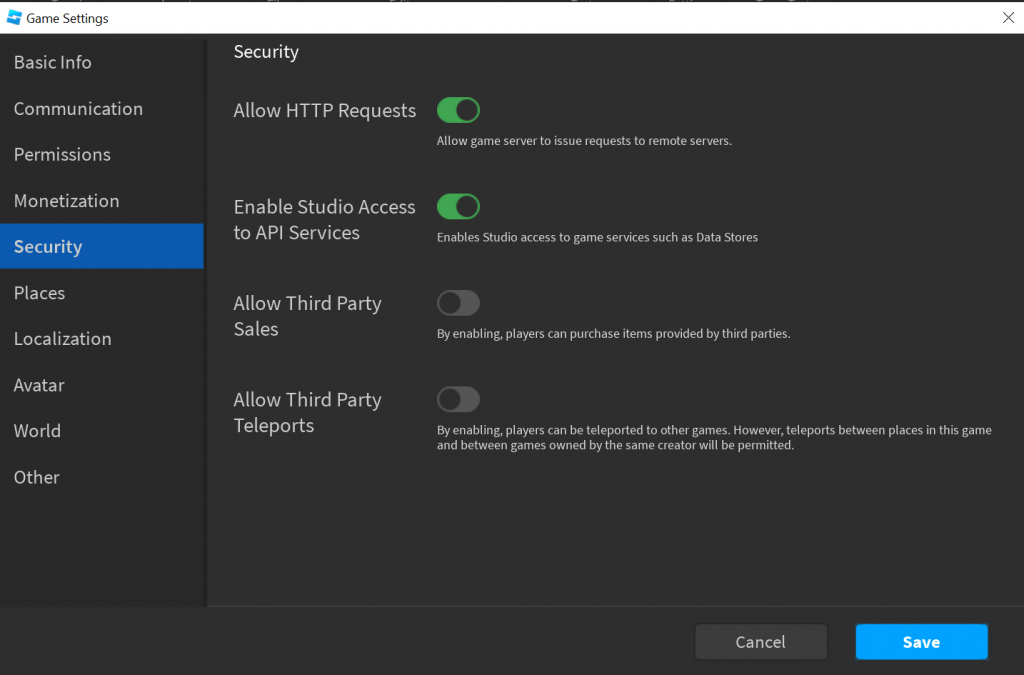
Local Script
First of all , add Screen GUI and text tabel to Starter GUI. Rename Text label to “PriceLabel” and add local script to it
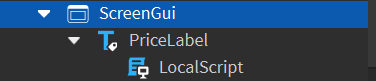
The provided script is a client-side script that listens for events sent from the server via a RemoteEvent named “Getprice”. Here’s a step-by-step overview:
Find RemoteEvent:
- Find the RemoteEvent named “Getprice” in
ReplicatedStorage
.
local remoteEvent = game.ReplicatedStorage:FindFirstChild("Getprice")
Check if RemoteEvent Exists:
- Verify if the RemoteEvent was found before proceeding.
if remoteEvent then
Connect to Client Event:
- Connect to the
OnClientEvent
event of the RemoteEvent to handle incoming data from the server.
remoteEvent.OnClientEvent:Connect(function(price)
Print Received Value:
- Print the received value (price) to the output console for debugging purposes.
print("Received value from server:", price)
Access ScreenGui and TextLabel:
- Assuming you have a
ScreenGui
with aTextLabel
named ‘PriceLabel’ in the player’s GUI hierarchy, access these elements.
local ScreenGui = game.Players.LocalPlayer:WaitForChild("PlayerGui"):WaitForChild("ScreenGui")
local PriceLabel = ScreenGui:WaitForChild("PriceLabel")
Update TextLabel with Price:
- Update the
TextLabel
with the received price information.
PriceLabel.Text = "Net Worth: " .. tostring(price)
End of If Statement:
- Close the
if
statement.
end)
The script essentially listens for price data from the server, prints it to the output for debugging, and updates a TextLabel
in the player’s GUI with the received net worth information. Make sure that the GUI elements (ScreenGui
and PriceLabel
) are correctly set up on the client side to avoid errors.
Full Local Script:
local remoteEvent = game.ReplicatedStorage:FindFirstChild("Getprice")
if remoteEvent then
remoteEvent.OnClientEvent:Connect(function(price)
-- 'value' contains the data sent from the server (e.g., Price)
print("Received value from server:", price)
-- Assuming you have a TextLabel named 'PriceLabel' in a ScreenGui
local ScreenGui = game.Players.LocalPlayer:WaitForChild("PlayerGui"):WaitForChild("ScreenGui")
local PriceLabel = ScreenGui:WaitForChild("PriceLabel")
-- Update the TextLabel with the price
PriceLabel.Text = "Net Worth: " .. tostring(price)
end)
end
Task Conclusion
In conclusion, the provided script demonstrates an interaction between a Roblox game and an external API using the HTTP service and JSON encoding/decoding. The script fetches real-time stock data for a specified symbol (“RBLX”) from the Alpha Vantage API. The obtained net worth is then sent to all clients through a RemoteEvent named “Getprice.”

The script is divided into two parts:
- Server-Side Script (Fetching and Broadcasting):
- Utilizes the
HttpService
to make an asynchronous HTTP request to the Alpha Vantage API, fetching global quote data for the specified stock symbol. - Parses the JSON response to extract the net worth value.
- Fires a RemoteEvent named “Getprice” to all clients, sending the net worth data.
- Utilizes the
- Client-Side Script (Receiving and Displaying):
- Finds the “Getprice” RemoteEvent in
ReplicatedStorage
. - Connects a function to the
OnClientEvent
event of the RemoteEvent, which triggers when the server fires the event. - Prints the received value to the output console for debugging.
- Retrieves a
ScreenGui
and aTextLabel
named ‘PriceLabel’ from the player’s GUI hierarchy. - Updates the
TextLabel
with the received net worth, displaying it as “Net Worth: [value].”
- Finds the “Getprice” RemoteEvent in
This script allows developers to integrate real-time financial data into their Roblox games, providing a dynamic and interactive element based on external API information. Developers can customize the GUI elements and further enhance the script by incorporating error handling and additional features based on the received data.
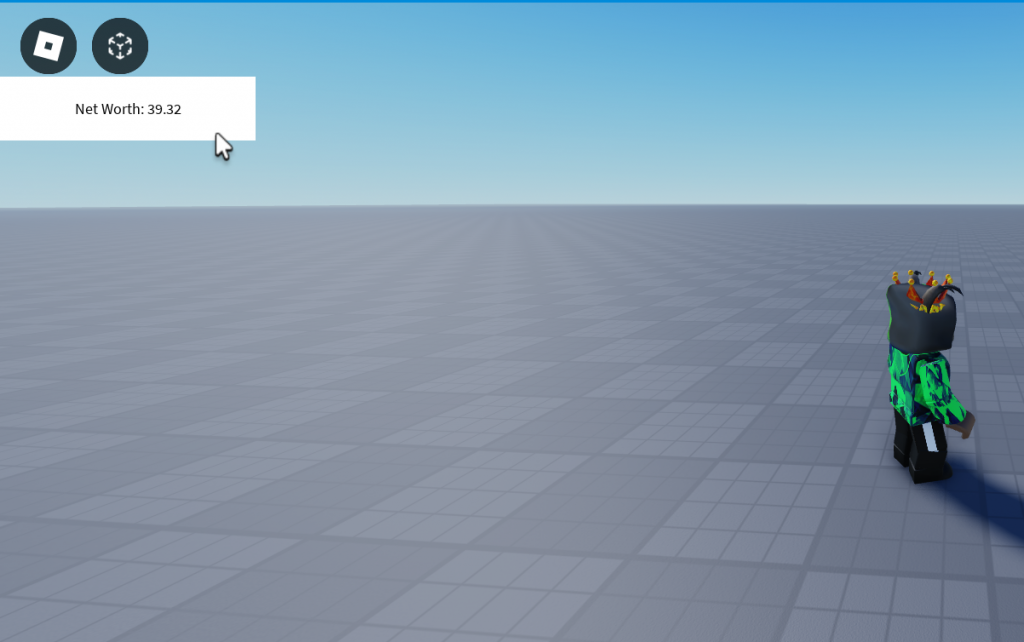