Introduction
In this lesson, we will explore a simple Roblox Studio script that allows a player to move a part to the position of their mouse click. This script utilizes basic concepts such as player input, part manipulation, and loops. By the end of this lesson, you’ll understand how to create interactive and responsive components in your Roblox game.
Prerequisites
Before starting this lesson, make sure you have the following:
- Basic understanding of Lua programming language.
- Roblox Studio installed and a basic knowledge of its interface.
Explanation
- Player and Mouse Setup:
local player = game.Players.LocalPlayer
local mouse = player:GetMouse()
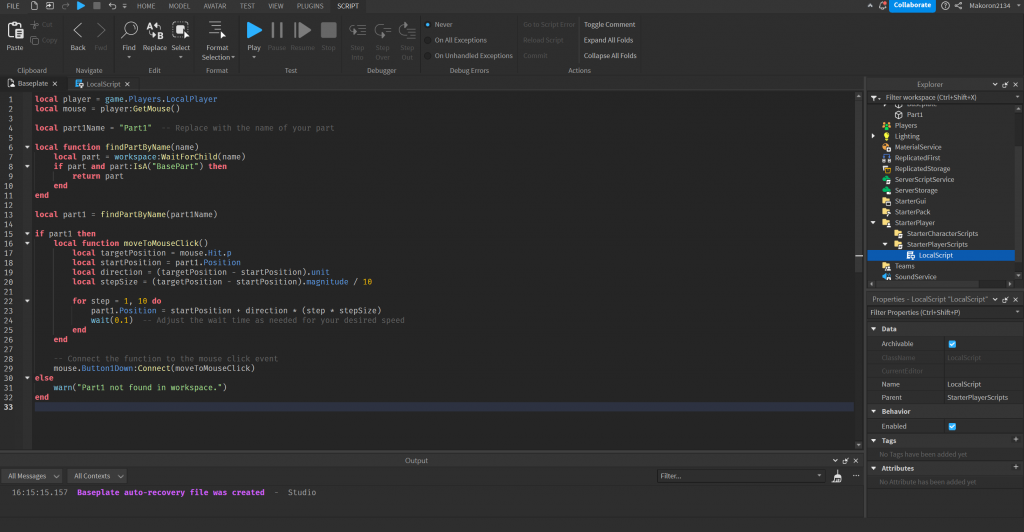
These lines retrieve the local player and their mouse, which are essential for capturing player input.
Part Identification:
local part1Name = "Part1" local function findPartByName(name) local part = workspace:WaitForChild(name) if part and part:IsA("BasePart") then return part end end local part1 = findPartByName(part1Name)
These lines define a function findPartByName
to search for a part by name within the workspace. The result is stored in the part1
variable.
Move to Mouse Click:
local function moveToMouseClick() local targetPosition = mouse.Hit.p local startPosition = part1.Position local direction = (targetPosition - startPosition).unit local stepSize = (targetPosition - startPosition).magnitude / 10 for step = 1, 10 do part1.Position = startPosition + direction * (step * stepSize) wait(0.1) end end
The moveToMouseClick
function calculates the direction and distance between the current part position and the mouse click position. It then moves the part in increments over 10 steps to reach the target position.
Connect Function to Mouse Click Event:
mouse.Button1Down:Connect(moveToMouseClick)
This line connects the moveToMouseClick
function to the Button1Down
event of the mouse, triggering the movement when the left mouse button is clicked.

Check if Part Exists:
if part1 then -- Code to move the part and connect events else warn("Part1 not found in workspace.") end
It checks if the part was successfully found. If it exists, the script proceeds to set up the movement; otherwise, a warning is issued.
Script Overview
Let’s break down the provided Lua code:
local player = game.Players.LocalPlayer
local mouse = player:GetMouse()
local part1Name = "Part1"
local function findPartByName(name)
local part = workspace:WaitForChild(name)
if part and part:IsA("BasePart") then
return part
end
end
local part1 = findPartByName(part1Name)
if part1 then
local function moveToMouseClick()
local targetPosition = mouse.Hit.p
local startPosition = part1.Position
local direction = (targetPosition - startPosition).unit
local stepSize = (targetPosition - startPosition).magnitude / 10
for step = 1, 10 do
part1.Position = startPosition + direction * (step * stepSize)
wait(0.1)
end
end
mouse.Button1Down:Connect(moveToMouseClick)
else
warn("Part1 not found in workspace.")
end
Conclusion
By understanding and modifying this script, you can create interactive elements in your Roblox game that respond to player input. Experiment with different parts, speeds, and events to enhance the user experience in your game.