Introduction
Roblox Studio allows developers to create interactive and dynamic experiences within games. One exciting aspect is the ability to script parts to react to player interactions. In this tutorial, we’ll explore a simple yet effective script that makes a part bounce when touched in Roblox Studio.
Place this script inside a part that you want to bounce
local part = script.Parent -- Get the part this script is attached to
-- Function to handle collisions
local function onCollision(hit)
local humanoid = hit.Parent:FindFirstChildOfClass("Humanoid") -- Check if the object collided with is a player
if humanoid then
-- Handle player collision (you can add custom actions here)
print("Player touched the part!")
else
-- Handle non-player collision (bounce the object)
local otherPart = hit.Parent -- Get the part that collided with this part
local bounceForce = Vector3.new(0, 50, 0) -- Adjust the force as needed
-- Apply force to both parts to simulate bouncing
part.Velocity = bounceForce
otherPart.Velocity = -bounceForce
end
end
-- Connect the collision function to the Touched event of the part
part.Touched:Connect(onCollision)
Explanation
- Setting the Part: The script begins by assigning the variable
part
to the part the script is attached to usingscript.Parent
. - Collision Handling Function: The
onCollision
function is defined to handle collisions. It checks if the object that collided with the part is a player (Humanoid
class). If it is, you can customize actions for player interactions. In the provided example, a message is printed to the console. - Non-Player Collision Handling: If the collision involves a non-player object, a bouncing effect is triggered. The collided part’s velocity is altered to simulate a bounce. The
bounceForce
variable determines the force applied during the bounce and can be adjusted based on your desired effect. - Connecting the Function: The script connects the
onCollision
function to theTouched
event of the part. This ensures that the function is called whenever the part is touched.
Lesson: Understanding and Customizing the Script
Understanding the Code
- Variables: Understand the use of the
part
variable to reference the part the script is attached to. - Function: Analyze the
onCollision
function. Recognize how it checks for player collision and handles non-player collisions by applying forces. - Velocity: Notice how the script uses the
Velocity
property to create a bouncing effect. The force is applied to both the current part (part
) and the colliding part (otherPart
).
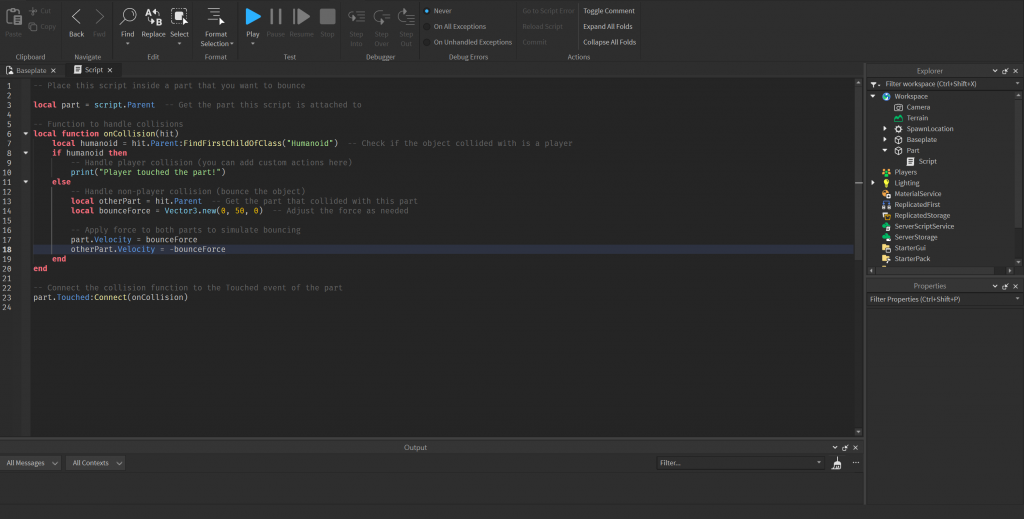
Customization
- Adjusting Bounce Force: Experiment with the
bounceForce
vector to change the intensity and direction of the bounce. - Adding Actions for Players: Enhance the script by adding custom actions when a player touches the part. You could teleport the player, play a sound, or trigger an animation.
- Modifying Player Detection: Modify the script to detect specific types of players or objects. You can customize the conditions for player interactions.
Conclusion
This script provides a foundation for creating interactive and dynamic elements in your Roblox games. Understanding and customizing scripts is crucial for game development, allowing you to tailor experiences to your creative vision. Experiment with the provided code and build upon it to create unique and engaging gameplay mechanics in Roblox Studio.