This lesson will guide you through creating a simple Roblox script that activates a particle emitter when a player’s character touches a specific part. The script is written in Lua, the scripting language used in Roblox Studio.
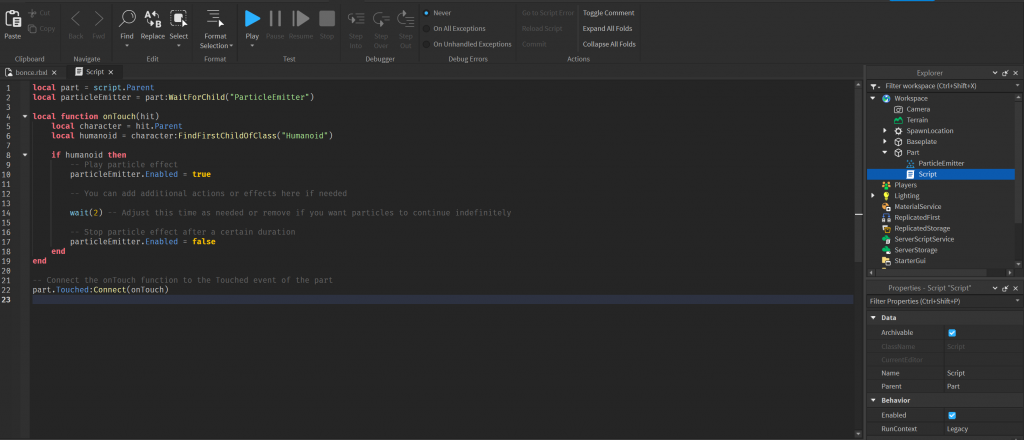
Script Explanation: Let’s break down the provided script step by step:
local part = script.Parent
: This line creates a local variable namedpart
and assigns it the value of the script’s parent. In Roblox, scripts are often placed inside objects (such as parts), andscript.Parent
refers to the object that contains the script.local particleEmitter = part:WaitForChild("ParticleEmitter")
: This line creates another local variable,particleEmitter
, and assigns it the value of the child object named “ParticleEmitter” within the part.WaitForChild
is used to wait for the specified child to exist before proceeding.local function onTouch(hit) ... end
: This declares a local function namedonTouch
that takes a parameterhit
. This function will be executed when the part is touched by any object.local character = hit.Parent
: This line retrieves the parent of the object that touched the part. In most cases, this would be the character model of a player.local humanoid = character:FindFirstChildOfClass("Humanoid")
: This line looks for the first child of the character object that is an instance of the “Humanoid” class. This is done to ensure that the object touching the part is a player character.if humanoid then ... end
: This conditional statement checks if a humanoid was found. If true, it means a player touched the part.particleEmitter.Enabled = true
: This line sets theEnabled
property of the ParticleEmitter to true, activating the emission of particles.wait(2)
: The script pauses execution for 2 seconds using thewait
function. Adjust this value based on the desired duration of particle emission.particleEmitter.Enabled = false
: After the wait, this line disables the particle emitter, stopping the emission of particles.part.Touched:Connect(onTouch)
: This line connects theonTouch
function to theTouched
event of the part. This ensures that theonTouch
function is called whenever the part is touched.
Analysis:
- The script utilizes the
Touched
event, which is triggered when an object is touched by another object in the game. - It checks if the touching object is a player character by looking for a “Humanoid” class within the object’s children.
- Particle emission is controlled by setting the
Enabled
property of the ParticleEmitter. - The script includes a brief delay using
wait
before disabling the particle emission, allowing for a controlled duration of the particle effect.
Customization: Feel free to customize the script based on your needs. You can adjust particle properties, add more effects, or modify the duration of particle emission by changing the wait
duration.

Script Overview
local part = script.Parent
local particleEmitter = part:WaitForChild("ParticleEmitter")
local function onTouch(hit)
local character = hit.Parent
local humanoid = character:FindFirstChildOfClass("Humanoid")
if humanoid then
-- Play particle effect
particleEmitter.Enabled = true
-- You can add additional actions or effects here if needed
wait(2) -- Adjust this time as needed or remove if you want particles to continue indefinitely
-- Stop particle effect after a certain duration
particleEmitter.Enabled = false
end
end
-- Connect the onTouch function to the Touched event of the part
part.Touched:Connect(onTouch)
Conclusion
provided script offers a simple yet effective solution for creating touch-activated particle emission in Roblox Studio. The script utilizes fundamental concepts such as events, conditional statements, and delays to achieve its functionality.