Roblox Studio provides a powerful environment for game developers to bring their creations to life. One exciting aspect of game development is incorporating interactive elements that engage players and enhance the overall gaming experience. In this lesson, we’ll explore how to create a simple interactive sound effect using a Click Detector and a Lua script.
Lesson Overview:
Objective: Enable a sound effect to play when a player clicks on a specific part in the game.
Prerequisites:
- Basic understanding of Roblox Studio.
- Familiarity with the Lua programming language.
Step 1: Setting Up the Part
- Open Roblox Studio and create a new or open an existing game.
- Insert a part into the workspace where you want the interactive sound effect to occur.
- Inside this part, insert a Click Detector. Right-click on the part, select “Insert Object,” and then choose “ClickDetector.”
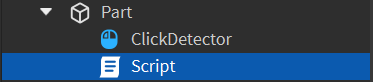
Step 2: Writing the Lua Script
Now, let’s create a Lua script that will be responsible for playing the sound when the part is clicked.
-- Variables
local part = script.Parent -- Reference to the part
local clickDetector = part:WaitForChild("ClickDetector") -- Wait for Click Detector to be present
local musicId = "rbxassetid://123456789" -- Replace with your desired Music ID
-- Function to play sound
local function playSound()
local sound = Instance.new("Sound")
sound.SoundId = musicId
sound.Parent = part
sound:Play()
wait(sound.TimeLength) -- Wait for the sound to finish playing
sound:Destroy() -- Clean up the sound object
end
-- Connect the function to the Click Detector's MouseClick event
clickDetector.MouseClick:Connect(playSound)
Replace "rbxassetid://123456789"
with the actual Music ID of the sound you want to play. This script creates a function (playSound
) that, when called, instantiates a sound object, assigns the specified Music ID, plays the sound, waits for it to finish, and then destroys the sound object.
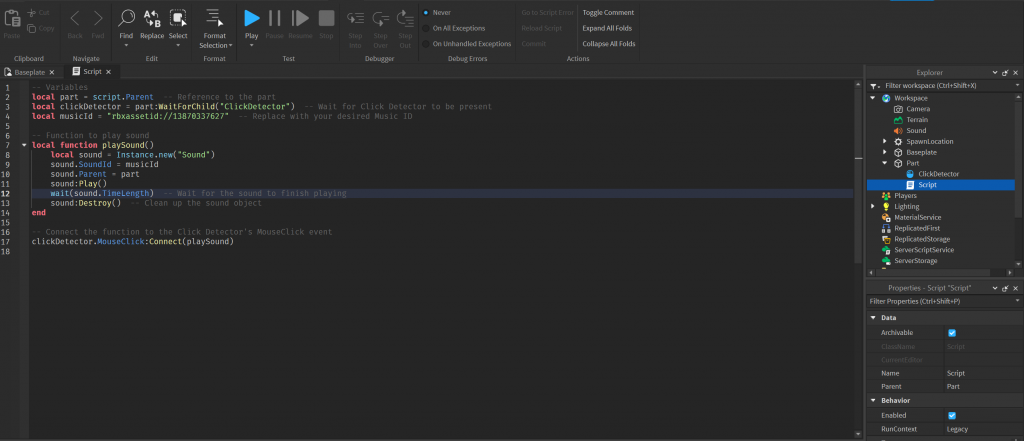
Step 3: Testing the Interactive Sound
- Click on the “Play” button in Roblox Studio to test your game.
- Navigate to the part with the Click Detector.
- Click on the part and observe the sound effect.
Conclusion:
Congratulations! You’ve successfully implemented an interactive sound effect in your Roblox game. This lesson covers the basics of using Click Detectors and Lua scripting to create engaging and interactive elements. Feel free to experiment with different sounds and customize the script to suit your game’s unique atmosphere. Incorporating interactive elements enhances player engagement and contributes to a more immersive gaming experience.