Roblox Studio provides a versatile environment for game developers to create immersive experiences. One exciting aspect is the ability to incorporate dynamic interactions, such as explosions, into the game world. The provided script is a simple example of how you can implement an explosion when a player touches a specific part in your Roblox game.
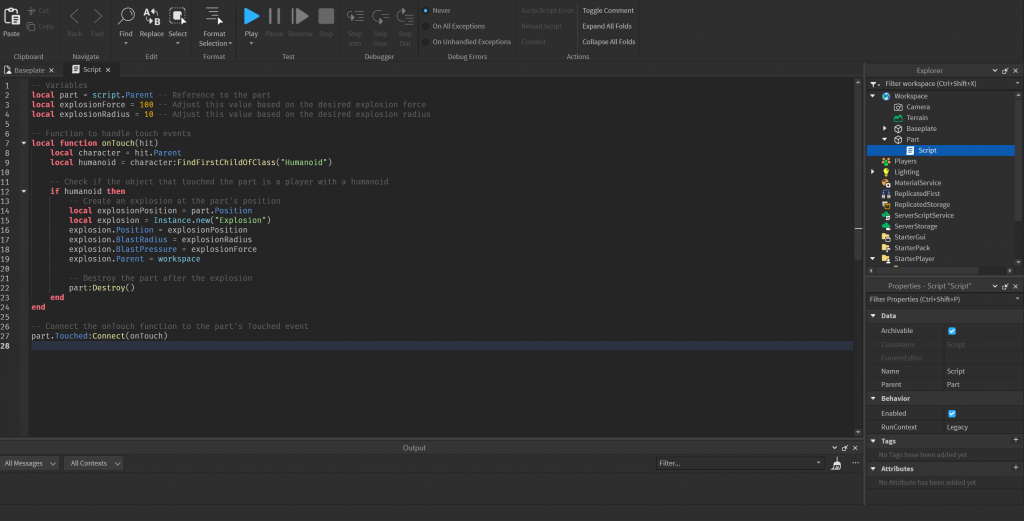
Let’s break down the script to understand its functionality:
-- Variables
local part = script.Parent -- Reference to the part
local explosionForce = 100 -- Adjust this value based on the desired explosion force
local explosionRadius = 10 -- Adjust this value based on the desired explosion radius
The script begins by declaring variables. part
is a reference to the part the script is attached to. explosionForce
and explosionRadius
are parameters that determine the characteristics of the explosion. You can tweak these values to achieve the desired impact in your game.
-- Function to handle touch events
local function onTouch(hit)
local character = hit.Parent
local humanoid = character:FindFirstChildOfClass("Humanoid")
-- Check if the object that touched the part is a player with a humanoid
if humanoid then
-- Create an explosion at the part's position
local explosionPosition = part.Position
local explosion = Instance.new("Explosion")
explosion.Position = explosionPosition
explosion.BlastRadius = explosionRadius
explosion.BlastPressure = explosionForce
explosion.Parent = workspace
-- Destroy the part after the explosion
part:Destroy()
end
end
The script defines a function called onTouch
, which is triggered when the Touched
event of the part is activated. The hit
parameter represents the object that touched the part.
Inside the function, it checks if the touching object has a humanoid (which is typical for players). If the condition is met, an explosion is created at the position of the part using the values set earlier. The explosion is then inserted into the game’s workspace.
After the explosion, the script destroys the part to simulate its destruction as a result of the explosion.
-- Connect the onTouch function to the part's Touched event
part.Touched:Connect(onTouch)
Finally, the script connects the onTouch
function to the Touched
event of the part. This ensures that the explosion logic is executed whenever the part is touched by an object with a humanoid.
To use this script, attach it to the part you want to be explosive in Roblox Studio. Adjust the explosionForce
and explosionRadius
variables to fine-tune the explosion’s impact. This script provides a foundation for creating explosive interactions in your Roblox game.