In this task, we’ll explore a script that generates terrain using Perlin noise. The script utilizes the Roblox Lua scripting language to create a voxel-based terrain with varying heights and colors. This is a basic introduction to procedural terrain generation using Perlin noise.
Step 1: Configuration
local gridSize = 50
local cellSize = 10
local maxHeight = 50
local frequency = 0.1
local seed = 42
This section defines the configuration parameters for the terrain generation. gridSize
determines the size of the grid, cellSize
specifies the size of each voxel, maxHeight
sets the maximum height of the terrain, frequency
controls the frequency of the Perlin noise, and seed
is used to initialize the random number generator for consistent results.
Step 2: Function to generate Perlin noise
local function generateNoise(x, y)
local p = math.noise(x * frequency, y * frequency, seed)
return p * maxHeight
end
This function uses Perlin noise to generate terrain heights based on input coordinates x
and y
. The math.noise
function generates a pseudo-random value based on the input coordinates and the specified seed. The result is then scaled by maxHeight
to ensure it falls within the desired height range.
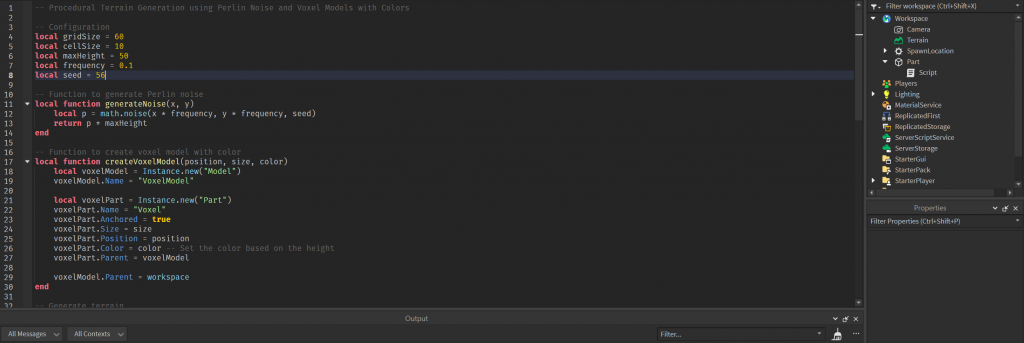
Step 3: Function to create voxel model with color
local function createVoxelModel(position, size, color)
local voxelModel = Instance.new("Model")
voxelModel.Name = "VoxelModel"
local voxelPart = Instance.new("Part")
voxelPart.Name = "Voxel"
voxelPart.Anchored = true
voxelPart.Size = size
voxelPart.Position = position
voxelPart.Color = color -- Set the color based on the height
voxelPart.Parent = voxelModel
voxelModel.Parent = workspace
end
This function creates a voxel model with a single part. The position
, size
, and color
parameters determine the position, size, and color of the voxel, respectively. The model is then parented to the workspace.
Step 4: Generate terrain
for x = 1, gridSize do
for y = 1, gridSize do
local height = generateNoise(x, y)
local position = Vector3.new(x * cellSize, height / 2, y * cellSize)
local size = Vector3.new(cellSize, height, cellSize)
-- Set color based on height
local color
if height > maxHeight * 0.75 then
color = Color3.new(0, 0, 1) -- Blue for higher terrain
else
color = Color3.new(0, 1, 0) -- Green for lower terrain
end
createVoxelModel(position, size, color)
end
end
This section generates the terrain by looping through each grid cell. It calculates the height using the generateNoise
function, determines the position and size of the voxel based on the grid cell and height, and sets the color based on the height. The createVoxelModel
function is then called to create and place the voxel in the workspace.
The color is determined by comparing the height to a threshold (maxHeight * 0.75
), and higher terrain is colored blue while lower terrain is colored green.
In summary, this script generates a terrain using Perlin noise and voxel models with varying heights and colors based on the generated terrain height. Higher terrain is displayed in blue, while lower terrain is displayed in green. The result is a procedurally generated landscape.

Lesson Steps
1. Understanding Perlin Noise
Explain the concept of Perlin noise, a gradient noise function used for procedural texture generation. Emphasize that it produces smooth, natural-looking patterns.
2. Configuring Terrain Generation
Discuss the significance of the configuration parameters:
gridSize
andcellSize
determine the size of the terrain grid and individual cells.maxHeight
controls the maximum height of the terrain.frequency
influences the smoothness of the terrain.seed
ensures reproducibility in terrain generation.
3. Perlin Noise Generation
Explain the generateNoise
function, emphasizing how it calculates Perlin noise using the specified frequency and seed.
4. Voxel Model Creation
Discuss the createVoxelModel
function, which creates a voxel model with a colored part. Highlight the importance of anchoring the part and setting its size, position, and color.
5. Terrain Generation Loop
Walk through the nested loops responsible for generating terrain. Explain how the script calculates the height for each cell, sets color based on height, and creates voxel models accordingly.
6. Color Representation
Explain the color choices based on height. Blue represents higher terrain, while green represents lower terrain. Discuss the flexibility of adjusting these color thresholds.
Conclusion
By the end of this lesson, students should understand the basics of procedural terrain generation using Perlin noise in Lua scripting for Roblox. They will have insights into configuring parameters, generating noise, creating voxel models, and representing terrain with varying colors.