In Roblox Studio, strings and tables are fundamental data types used for storing and manipulating data within scripts. Let’s take a closer look at each of them:
A string is a sequence of characters enclosed within quotation marks (either single or double). It can contain letters, numbers, symbols, and whitespace. Strings are commonly used to store and manipulate text-based data such as names, messages, and descriptions.
Here’s an example of declaring a string variable in Lua, the scripting language used in Roblox:
local myString = "Hello, world!"
In this case, myString
is a variable that holds the value “Hello, world!”.
Strings can be concatenated (combined) using the concatenation operator (..
):
local greeting = "Hello" local name = "
Joseph" local message = greeting .. ", " .. name .. "!" print(message) --> "Hello, Joseph!"
Defining strings using double quotes or single quotes.
In Roblox Studio, you have the flexibility to define strings using either double quotes ("
) or single quotes ('
). Both methods are valid and will create a string with the enclosed characters. Let’s explore how to define strings using both double quotes and single quotes in Lua scripting within Roblox Studio:
- Double Quotes:
local myString = "Hello, world!"
In this example, the string “Hello, world!” is enclosed within double quotes, and the variable myString
is assigned that value. Double quotes are commonly used to define strings in Lua and are the preferred convention in Roblox Studio.
- Single Quotes:
local myString = 'Hello, world!'
Here, the string “Hello, world!” is enclosed within single quotes. This method is also acceptable in Lua and Roblox Studio. However, it is recommended to use double quotes for consistency and readability, as it is the convention widely followed in Lua scripting.
It’s important to note that if your string contains a quote character within it, you should use the opposite type of quote to enclose the string. For example:
local myString = "He said, 'Hello!'"
In this case, the string contains single quotes within it, so we use double quotes to enclose the entire string.
Similarly:
local myString = 'She said, "Hi!"'
In this case, the string contains double quotes within it, so we use single quotes to enclose the entire string.
Regardless of whether you choose to use double quotes or single quotes, the important thing is to be consistent within your codebase to maintain readability and avoid confusion.
Concatenating strings using the concatenation operator
In Roblox Studio, you can concatenate strings by using the concatenation operator (..
). The concatenation operator allows you to combine multiple strings into a single string. Here’s how you can concatenate strings in Lua scripting within Roblox Studio:
-- Concatenating strings
local greeting = "Hello"
local name = "John"
local message = greeting .. ", " .. name .. "!"
print(message) -- Output: Hello, John!
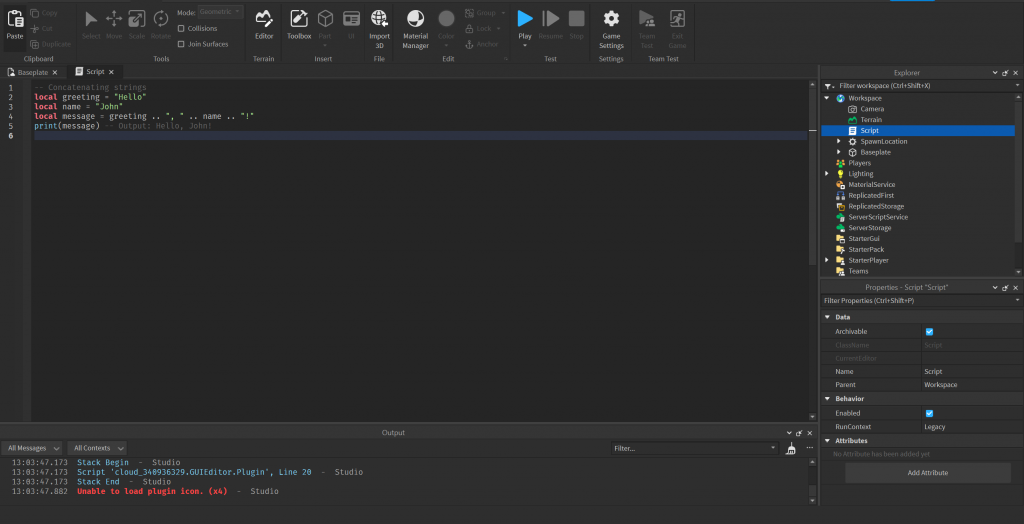
In this example, three strings are concatenated together to form the message
string. The concatenation operator (..
) joins the strings on either side of it.
Let’s break down the code:
- The variable
greeting
holds the string “Hello”. - The variable
name
holds the string “John”. - The
message
variable is created by concatenating the strings using the concatenation operator (..
). The resulting string is “Hello, John!”. - Finally, the
message
is printed to the console usingprint()
.
You can concatenate any number of strings together using the concatenation operator. Here’s an example with more strings:
local part1 = "Roblox"
local part2 = " Studio"
local part3 = " is awesome!"
local combined = part1 .. part2 .. part3
print(combined) -- Output: Roblox Studio is awesome!
In this case, the variables part1
, part2
, and part3
hold different parts of the final string. The concatenation operator (..
) is used multiple times to concatenate all the parts together, resulting in the string “Roblox Studio is awesome!”.
Concatenating strings is useful in various scenarios, such as constructing dynamic messages, building file paths, generating chat messages, or forming complex sentences. By combining different strings, you can create more versatile and personalized text output in your Roblox games and experiences.
Using string methods to transform strings (e.g., upper, lower, sub).
In Roblox Studio, you can use various string methods to transform strings and manipulate their contents. These methods are built-in Lua functions that allow you to perform operations such as converting strings to uppercase or lowercase, extracting substrings, and more. Here are a few commonly used string methods in Roblox Studio:
string.upper()
andstring.lower()
:string.upper()
converts a string to uppercase.string.lower()
converts a string to lowercase.
Example usage:
local myString = "Hello, World!"
print(string.upper(myString)) -- Output: HELLO, WORLD!
print(string.lower(myString)) -- Output: hello, world!
string.sub()
:string.sub(str, start [, end])
extracts a substring from the given stringstr
.- The
start
andend
parameters indicate the range of characters to extract. - If
end
is omitted, the substring extends to the end of the string.
Example usage:
local myString = "Hello, World!"
print(string.sub(myString, 1, 5)) -- Output: Hello
print(string.sub(myString, 8)) -- Output: World!
string.len()
:string.len(str)
returns the length of the given stringstr
.
Example usage:
local myString = "Hello, World!"
print(string.len(myString)) -- Output: 13
string.find()
:string.find(str, pattern [, init [, plain]])
searches for the first occurrence ofpattern
in the given stringstr
.- The optional
init
parameter specifies the starting index of the search. - The optional
plain
parameter, if set totrue
, treats the pattern as a plain string rather than a pattern.
Example usage:
local myString = "Hello, World!"
local startIndex, endIndex = string.find(myString, "World")
print(startIndex, endIndex) -- Output: 8 12
These are just a few examples of the string methods available in Lua. There are many more functions and methods you can explore and utilize to manipulate and transform strings in Roblox Studio.
Using string methods allows you to perform tasks like changing case, extracting specific portions, searching for patterns, and more. These operations are valuable for handling user input, manipulating text-based data, and implementing various game mechanics within your Roblox games and experiences.
Replacing characters or patterns within a string using gsub
In Roblox Studio, you can replace characters or patterns within a string using the string.gsub()
function. The gsub
function allows you to globally substitute occurrences of a specific character or pattern in a string with another character or pattern. Here’s how you can use gsub
in Lua scripting within Roblox Studio:
-- Replacing characters or patterns within a string
local myString = "Hello, World!"
local newString = string.gsub(myString, "o", "e")
print(newString) -- Output: Helle, Werld!
In this example, the gsub
function is used to replace all occurrences of the character “o” in the myString
variable with the character “e”. The resulting string is stored in the newString
variable and printed to the console.
Here’s a breakdown of the code:
- The
myString
variable holds the string “Hello, World!”. - The
string.gsub()
function takes three parameters:- The first parameter is the string you want to modify (
myString
). - The second parameter is the character or pattern you want to replace (
"o"
). - The third parameter is the replacement character or pattern (
"e"
).
- The first parameter is the string you want to modify (
- The
gsub
function scans themyString
and replaces all occurrences of “o” with “e”. - The modified string is stored in the
newString
variable. - Finally, the
newString
is printed to the console.
The gsub
function can also work with patterns using Lua’s pattern matching syntax. For example:
local myString = "The quick brown fox jumps over the lazy dog."
local newString = string.gsub(myString, "%a+", "word")
print(newString)
In this case, the %a+
pattern matches one or more alphabetical characters. The gsub
function replaces all occurrences of such patterns with the word “word”.
Using gsub
, you can perform powerful replacements within strings, whether it’s replacing single characters, specific patterns, or even utilizing regular expressions. This functionality is handy for tasks such as text manipulation, filtering, and formatting within your Roblox games and experiences.
Using string indexing to access individual characters
In Roblox Studio, you can use string indexing to access individual characters within a string. String indexing allows you to retrieve or modify specific characters based on their position within the string. Here’s how you can use string indexing in Lua scripting within Roblox Studio:
-- Accessing individual characters in a string
local myString = "Hello, World!"
-- Accessing a specific character
local firstCharacter = myString:sub(1, 1) -- Using the sub method
local secondCharacter = myString:sub(3, 3)
local thirdCharacter = myString:sub(-1, -1)
print(firstCharacter) -- Output: H
print(secondCharacter) -- Output: l
print(thirdCharacter) -- Output: !
In this example, we use the sub
method to access individual characters in the myString
variable. The sub
method takes two parameters: the starting index and the ending index of the desired substring. In this case, we specify the same index for both parameters to retrieve a single character.
It’s important to note that string indexing in Lua starts from index 1 for the first character, unlike some other programming languages that start from index 0.
Alternatively, you can use square brackets ([]
) for string indexing:
local myString = "Hello, World!"
-- Accessing a specific character using square brackets
local firstCharacter = myString[1]
local secondCharacter = myString[3]
local thirdCharacter = myString[#myString]
print(firstCharacter) -- Output: H
print(secondCharacter) -- Output: l
print(thirdCharacter) -- Output: !
In this case, we use square brackets to directly access characters at specific indices within the string.
String indexing allows you to access and manipulate individual characters within a string, which can be useful for various tasks, such as parsing input, checking conditions, or modifying specific characters in text-based data within your Roblox games and experiences.
Using string methods to extract substrings (e.g., find, match)
In Roblox Studio, you can use string methods to extract substrings from a larger string based on specific patterns or conditions. These string methods allow you to locate and retrieve substrings that match a certain pattern, find the position of a substring within a string, and more. Here are a couple of commonly used string methods for extracting substrings in Lua scripting within Roblox Studio:
string.find()
:string.find(str, pattern [, init [, plain]])
searches for the first occurrence ofpattern
in the given stringstr
.- The
init
parameter specifies the starting index for the search (optional). - The
plain
parameter, if set totrue
, treats the pattern as a plain string rather than a pattern (optional).
Example usage:
local myString = "Hello, World!"
local startIndex, endIndex = string.find(myString, "World")
print(startIndex, endIndex) -- Output: 8 12
In this example, string.find()
is used to locate the first occurrence of the pattern “World” in the myString
variable. It returns the starting index and the ending index of the found substring. In this case, the starting index is 8 and the ending index is 12.
string.match()
:string.match(str, pattern)
searches for the first occurrence ofpattern
in the given stringstr
.- It returns the matching substring if found or
nil
otherwise.
Example usage:
local myString = "The quick brown fox jumps over the lazy dog."
local match = string.match(myString, "brown %a+")
print(match) -- Output: brown fox
In this example, string.match()
is used to find the first occurrence of the pattern “brown” followed by one or more alphabetical characters in the myString
variable. It returns the matching substring “brown fox”.
These are just a few examples of the string methods available in Lua for extracting substrings. You can utilize more advanced patterns and techniques to match and retrieve specific substrings based on your requirements.
By using string methods like string.find()
and string.match()
, you can effectively extract relevant information, parse data, and perform operations on substrings within larger strings in your Roblox games and experiences.
Using string format to insert values into formatted strings
In Roblox Studio, you can use the string.format()
function to insert values into formatted strings. This function allows you to construct dynamic strings by incorporating placeholders that are replaced with actual values. Here’s how you can use string.format()
in Lua scripting within Roblox Studio:
-- Using string.format() to insert values into formatted strings
local name = "John"
local age = 25
local message = string.format("Hello, my name is %s and I am %d years old.", name, age)
print(message) -- Output: Hello, my name is John and I am 25 years old.
In this example, the string.format()
function is used to create the message
string. The function takes a format string as the first argument, which contains placeholders marked with %
followed by a format specifier (s
for strings, d
for integers). The subsequent arguments provide the actual values to be inserted into the placeholders.
Let’s break down the code:
- The
name
variable holds the string “John”. - The
age
variable holds the integer value 25. - The
string.format()
function constructs themessage
string:- The format string is
"Hello, my name is %s and I am %d years old."
. - The
%s
placeholder is replaced with the value ofname
(“John”). - The
%d
placeholder is replaced with the value ofage
(25).
- The format string is
- The resulting formatted string is stored in the
message
variable. - Finally, the
message
is printed to the console.
You can use various format specifiers with string.format()
to handle different data types and formatting requirements. Some commonly used format specifiers include %s
for strings, %d
for integers, %f
for floating-point numbers, and %02d
for zero-padded integers.
Here’s an example using multiple format specifiers:
local score = 95.75
local grade = "A"
local result = string.format("Your score is %.2f and your grade is %s.", score, grade)
print(result) -- Output: Your score is 95.75 and your grade is A.
In this case, the %f
format specifier is used to insert the score
value with two decimal places, and %s
is used to insert the grade
value.
Using string.format()
, you can create dynamic strings that incorporate variable values, making it useful for generating messages, displaying scores, formatting numerical data, and more within your Roblox games and experiences.
Creating tables
Tables in Lua are versatile data structures that can store multiple values of different types, including other tables. They are used to organize and represent complex data. Tables are similar to arrays or dictionaries in other programming languages.
Here’s an example of declaring and accessing values in a table:
local player = { name = "
Joseph", age = 25, score = 100 } print(player.name) --> "
Joseph" print(player.age) --> 25 print(player.score) --> 100
In this case, player
is a table with three key-value pairs. The keys (name
, age
, score
) are used to access the corresponding values.
Tables can also have arrays-like behavior, where values are stored in numeric indices starting from 1:
local fruits = {"apple", "banana", "orange"}
print(fruits[2]) --> "banana"
In this example, "banana"
is accessed using the index 2
in the fruits
table.
Tables provide flexibility for storing and organizing data in complex game systems, allowing you to create data structures such as inventories, leaderboards, and more.
Understanding strings and tables is essential for developing games in Roblox Studio, as they are used extensively in scripting to handle various aspects of gameplay, user interfaces, and data management.
Defining tables with curly braces ({}) or the table constructor
In Roblox Studio, you can define tables using either curly braces ({}
) or the table constructor. Tables are a fundamental data structure in Lua and allow you to store and organize data in key-value pairs or as arrays. Here’s how you can define tables using both methods:
- Curly Braces ({}):
-- Defining a table using curly braces
local myTable = { key1 = value1, key2 = value2, key3 = value3 }
In this method, you use curly braces to enclose the key-value pairs of the table. Each key-value pair consists of a key, followed by an equals sign (=
), and then the corresponding value. Multiple key-value pairs are separated by commas.
Here’s an example:
local player = {
name = "John",
score = 100,
level = 5
}
In this case, the player
table is defined with three key-value pairs: name
with the value “John”, score
with the value 100, and level
with the value 5.
- Table Constructor:
-- Defining a table using the table constructor
local myTable = table[key1] = value1, key2 = value2, key3 = value3
In this method, you use the table
keyword followed by square brackets to define the table. Inside the square brackets, you define the key-value pairs separated by commas.
Here’s an example:
local player = {
["name"] = "John",
["score"] = 100,
["level"] = 5
}
In this case, the player
table is defined using the table constructor with three key-value pairs.
Both methods achieve the same result: defining a table with key-value pairs. The curly brace method is more commonly used and generally considered more readable. However, the table constructor method allows for more flexibility in key naming, such as using keys with spaces or special characters.
You can access and manipulate table values using the keys. For example:
print(player.name) -- Output: John
print(player.score) -- Output: 100
print(player.level) -- Output: 5
player.score = 150
print(player.score) -- Output: 150
player["level"] = 6
print(player.level) -- Output: 6
In this case, we access and modify values in the player
table using both dot notation (player.name
, player.score
) and square bracket notation (player["level"]
).
Tables are versatile data structures in Lua and are extensively used in Roblox Studio for storing and organizing game data, configuring objects, managing inventories, implementing data structures like arrays or dictionaries, and much more.
Iterating over table elements using loops (e.g., for each, ipairs).
In Roblox Studio, you can iterate over table elements using loops to access and process each element within the table. Lua provides two commonly used loop constructs for iterating over tables: the for each
loop and the ipairs
loop. Here’s how you can use these loops to iterate over table elements:
for each
Loop:
-- Iterating over table elements using the for each loop
local myTable = {10, 20, 30, 40, 50}
for index, value in pairs(myTable) do
print(index, value)
end
In this example, the for each
loop iterates over the elements of the myTable
table. The pairs()
function is used to retrieve the key-value pairs of the table. The loop assigns each key to the index
variable and each value to the value
variable. Inside the loop, you can perform operations on each element or access their values.
The output of the above code would be:
1 10
2 20
3 30
4 40
5 50
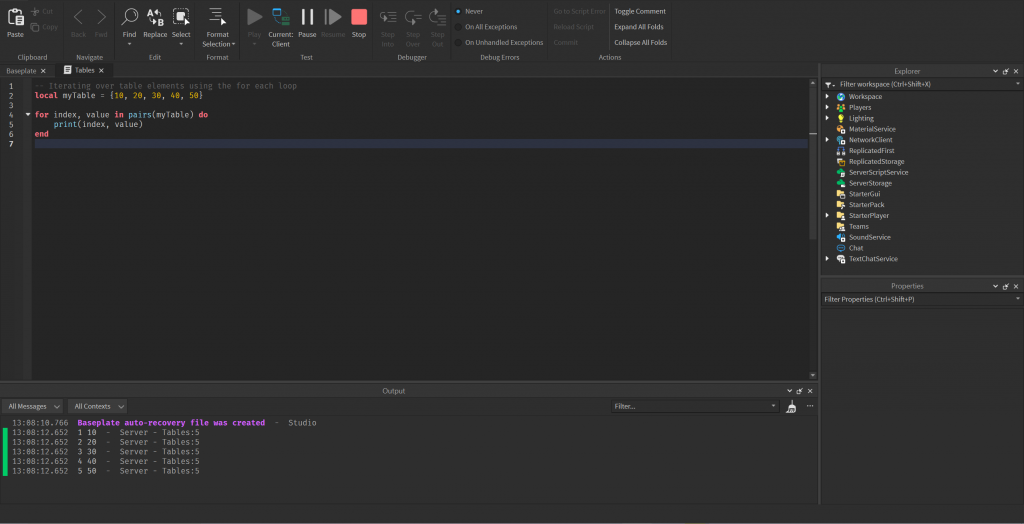
The pairs()
function iterates over the elements in an arbitrary order, as tables in Lua do not have an inherent order.
ipairs
Loop:
-- Iterating over table elements using the ipairs loop
local myTable = {10, 20, 30, 40, 50}
for index, value in ipairs(myTable) do
print(index, value)
end
In this example, the ipairs()
function is used to iterate over the elements of the myTable
table. The ipairs
function iterates over elements in numerical order based on their indices. The loop assigns each index to the index
variable and each corresponding value to the value
variable.
The output of the above code would be:
1 10
2 20
3 30
4 40
5 50
The ipairs()
function is particularly useful when iterating over tables with sequential numeric indices, such as arrays.
By using these loop constructs, you can easily iterate over table elements in Roblox Studio, perform operations on each element, access their values, and use them to drive game logic, update object properties, or manipulate data within your Roblox games and experiences.
Adding or removing elements using table.insert and table.remove
In Roblox Studio, you can add or remove elements from a table using the table.insert()
and table.remove()
functions. These functions allow you to manipulate the contents of a table by adding new elements at a specific position or removing elements from a table. Here’s how you can use these functions:
table.insert()
:
-- Adding elements to a table using table.insert()
local myTable = {"apple", "banana", "orange"}
table.insert(myTable, "grape") -- Add "grape" at the end of the table
table.insert(myTable, 2, "pear") -- Add "pear" at index 2
-- Print the updated table
for index, value in ipairs(myTable) do
print(index, value)
end
In this example, table.insert()
is used to add elements to the myTable
table. The function takes two arguments: the table you want to modify and the value you want to insert. Optionally, you can provide a second argument that specifies the index at which the value should be inserted. If no index is specified, the value is added at the end of the table.
The output of the above code would be:
1 apple
2 pear
3 banana
4 orange
5 grape
table.remove()
:
-- Removing elements from a table using table.remove()
local myTable = {"apple", "pear", "banana", "orange", "grape"}
table.remove(myTable) -- Remove the last element
table.remove(myTable, 2) -- Remove the element at index 2
-- Print the updated table
for index, value in ipairs(myTable) do
print(index, value)
end
In this example, table.remove()
is used to remove elements from the myTable
table. The function takes one or two arguments: the table from which you want to remove elements and the index of the element you want to remove. If no index is specified, the last element is removed.
The output of the above code would be:
1 apple
2 banana
3 orange
By using table.insert()
and table.remove()
, you can dynamically modify the contents of a table in Roblox Studio. This can be useful for managing lists, inventories, player data, and other dynamic collections of data within your Roblox games and experiences.
Table manipulation
In Roblox Studio, table manipulation refers to various operations performed on tables to modify their contents, restructure their data, or extract specific information. Lua provides several built-in functions and techniques for table manipulation. Here are some commonly used techniques for manipulating tables in Roblox Studio:
- Accessing and Modifying Table Elements:
- You can access and modify individual elements of a table using their indices or keys. For example:
local myTable = {10, 20, 30}
print(myTable[2]) -- Output: 20
myTable[3] = 40
print(myTable[3]) -- Output: 40
- Adding and Removing Elements:
- You can add new elements to a table using the
table.insert()
function, as discussed in the previous response. - To remove elements from a table, you can use the
table.remove()
function, also explained in the previous response.
- You can add new elements to a table using the
- Sorting Tables:
- Lua provides the
table.sort()
function to sort the elements of a table. It allows you to sort tables in ascending or descending order based on certain criteria.
- Lua provides the
local myTable = {3, 1, 4, 2}
table.sort(myTable) -- Sort in ascending order
for index, value in ipairs(myTable) do
print(index, value)
end
- The output would be:
1 1
2 2
3 3
4 4
- Merging Tables:
- You can merge two or more tables together using the
table.concat()
function. It concatenates the elements of multiple tables into a single table.
- You can merge two or more tables together using the
local table1 = {1, 2, 3}
local table2 = {4, 5, 6}
local mergedTable = table.concat(table1, table2)
for index, value in ipairs(mergedTable) do
print(index, value)
end
- The output would be:
1 1
2 2
3 3
4 4
5 5
6 6
- Iterating over Tables:
- As discussed in a previous response, you can use loop constructs like the
for each
loop (pairs()
) or theipairs()
loop to iterate over the elements of a table and perform operations on them.
- As discussed in a previous response, you can use loop constructs like the
local myTable = {10, 20, 30}
for index, value in ipairs(myTable) do
print(index, value)
end
- The output would be:
1 10
2 20
3 30
These are just a few examples of table manipulation techniques in Roblox Studio. Lua provides various other functions and methods for working with tables, such as copying tables, merging tables, filtering elements, and more. By effectively manipulating tables, you can organize and transform data within your Roblox games and experiences.
Storing strings in tables
In Roblox Studio, you can store strings in tables by assigning string values to specific indices or keys within the table. Tables are versatile data structures that can hold various types of data, including strings. Here’s how you can store strings in tables:
- Index-based Approach:
-- Storing strings in a table using index-based approach
local myTable = {}
myTable[1] = "Hello"
myTable[2] = "World"
-- Accessing the stored strings
print(myTable[1]) -- Output: Hello
print(myTable[2]) -- Output: World
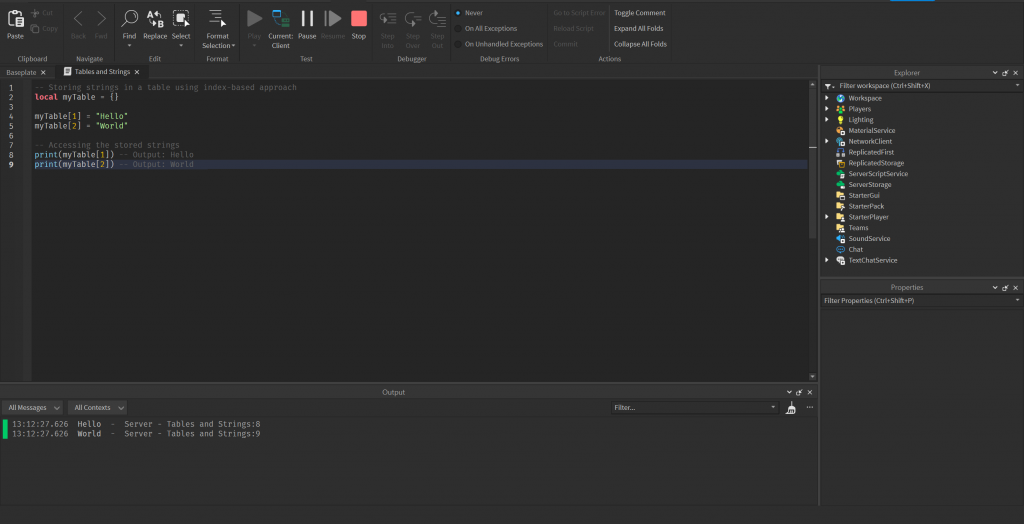
In this example, an empty table myTable
is created. Strings are then assigned to specific indices within the table using square bracket notation ([]
). The index 1
holds the string “Hello”, and the index 2
holds the string “World”. You can access the stored strings by referencing the corresponding indices.
- Key-based Approach:
-- Storing strings in a table using key-based approach
local myTable = {}
myTable["greeting"] = "Hello"
myTable["name"] = "John"
-- Accessing the stored strings
print(myTable["greeting"]) -- Output: Hello
print(myTable["name"]) -- Output: John
In this example, an empty table myTable
is created. Strings are assigned to specific keys within the table using square bracket notation. The key "greeting"
holds the string “Hello”, and the key "name"
holds the string “John”. You can access the stored strings by referencing the corresponding keys.
- Mixed Approach:
-- Storing strings in a table using a mix of index-based and key-based approach
local myTable = {}
myTable[1] = "Hello"
myTable["name"] = "John"
-- Accessing the stored strings
print(myTable[1]) -- Output: Hello
print(myTable["name"]) -- Output: John
In this example, both index-based and key-based approaches are used to store strings in the table. The index 1
holds the string “Hello” using the index-based approach, and the key "name"
holds the string “John” using the key-based approach. You can access the stored strings using the corresponding indices or keys.
Storing strings in tables allows you to organize and manipulate text data within your Roblox games and experiences. You can use tables to store strings associated with specific entities, messages, translations, or any other text-based information required in your game logic.
Parsing strings into tables
In Roblox Studio, you can parse strings into tables by splitting the string into individual components and organizing them within a table structure. This process is known as string parsing. You can use various techniques and functions available in Lua to achieve this. Here’s an example of how you can parse strings into tables:
-- Parsing a comma-separated string into a table
local csvString = "apple,banana,orange"
local dataTable = {}
for word in csvString:gmatch("[^,]+") do
table.insert(dataTable, word)
end
-- Accessing the parsed table elements
for index, value in ipairs(dataTable) do
print(index, value)
end
In this example, a comma-separated string csvString
is provided, which contains the values “apple”, “banana”, and “orange”. The string is parsed and stored in a table called dataTable
. The gmatch()
function is used to iterate through the string and extract each component separated by commas. The pattern "[^,]+"
matches one or more characters that are not commas. The table.insert()
function is used to insert each component into the dataTable
table.
The output of the above code would be:
1 apple
2 banana
3 orange
By using string parsing techniques like this, you can convert strings with specific patterns or separators into organized table structures. This can be useful for handling data in different formats, such as CSV files, serialized data, or custom string representations, within your Roblox games and experiences.
Splitting strings into tables based on a delimiter
In Roblox Studio, you can split strings into tables based on a delimiter using the string.split()
function or by manually iterating over the string and extracting substrings. Here are two approaches you can use to split strings into tables based on a delimiter:
Using string.split()
:
-- Splitting a string into a table using a delimiter local myString = "apple,banana,orange" local delimiter = "," local dataTable = string.split(myString, delimiter) -- Accessing the split table elements for index, value in ipairs(dataTable) do print(index, value) end
In this example, the string.split()
function is used to split the string myString
based on the delimiter .,
. The function returns a table containing the split substrings. You can then access the elements of the table using a loop or perform operations on them as needed.
Manual Splitting:
-- Splitting a string into a table manually using a delimiter local myString = "apple,banana,orange" local delimiter = "," local dataTable = {} local startIndex = 1 local endIndex = string.find(myString, delimiter) while endIndex do local substring = string.sub(myString, startIndex, endIndex - 1) table.insert(dataTable, substring) startIndex = endIndex + 1 endIndex = string.find(myString, delimiter, startIndex) end -- Adding the remaining part of the string local substring = string.sub(myString, startIndex) table.insert(dataTable, substring) -- Accessing the split table elements for index, value in ipairs(dataTable) do print(index, value) end
In this approach, the string myString
is manually split using the delimiter ,
. The string.find()
function is used to locate the index of the delimiter within the string. The string is iterated, extracting substrings between delimiters and adding them to the dataTable
table using table.insert()
. Finally, the remaining part of the string is added to the table. The elements can be accessed and processed using a loop or other table manipulation operations.
Both approaches achieve the same result of splitting a string into a table based on a delimiter. The string.split()
function provides a more convenient and concise way to accomplish this task, while the manual splitting approach offers more flexibility for custom parsing scenarios.
By splitting strings into tables based on a delimiter, you can organize and process data in a structured format within your Roblox games and experiences. This can be useful for handling inputs, parsing data files, or performing text-based operations within your game logic.
Conclusion
Working with strings and tables in Roblox Studio provides powerful capabilities for handling and manipulating data within your games and experiences. Strings allow you to store and manipulate text-based information, while tables provide a versatile data structure to organize, access, and modify collections of data.
When working with strings, you can define them using single or double quotes and utilize various string methods to transform and manipulate them. These methods include changing the case (upper, lower), extracting substrings (sub), replacing characters or patterns (gsub), and formatting strings (string.format).
Tables, on the other hand, can be defined using curly braces ({}) or the table constructor. They allow you to store and organize data, including strings, using index-based or key-based approaches. You can iterate over table elements using loops like for each
(pairs) or ipairs
and perform operations on them.
In addition, you can modify tables by adding or removing elements using table.insert()
and table.remove()
. Sorting tables, merging them with table.concat()
, and manipulating their structure provide further flexibility.
String parsing allows you to convert strings into tables based on specific delimiters, enabling structured data processing. Splitting strings into tables can be achieved using functions like string.split()
or by manually extracting substrings based on delimiters.
By leveraging strings and tables effectively, you can manage and process a wide range of data in your Roblox games and experiences. Whether it’s storing player information, managing inventories, handling data files, or implementing complex algorithms, understanding and utilizing string and table manipulation techniques will enhance your ability to create dynamic and interactive gameplay.