Tables in Roblox Studio
Tables in Roblox Studio serve as versatile data structures used to store and organize related data in key-value pairs. They are essential for managing complex data structures, such as player data, game settings, and level information, within Roblox games and experiences. Tables offer flexibility in storing various data types and can be nested to create hierarchical structures. Understanding tables is vital for implementing robust game mechanics, user interfaces, and data management systems in Roblox Studio.
Full lesson about tables in Roblox Studio:
Script Overview
In this script, we’ll create a basic inventory system in Roblox Studio using Lua scripting language. Let’s go through each part of the script and understand its functionality.
-- Create the inventory table
local Inventory = {}
Explanation:
- We start by creating an empty table named
Inventory
. This table will store the items in our inventory.
-- Function to add an item to the inventory
function Inventory:addItem(itemID)
if self[itemID] then
self[itemID] = self[itemID] + 1
else
self[itemID] = 1
end
end
Explanation:
- This function
addItem
is used to add an item to the inventory. - If the item already exists in the inventory (
self[itemID]
is not nil), then we increment its count by 1. - If the item doesn’t exist in the inventory, we add it with a count of 1.
-- Function to remove an item from the inventory
function Inventory:removeItem(itemID)
if self[itemID] then
self[itemID] = self[itemID] - 1
if self[itemID] <= 0 then
self[itemID] = nil
end
else
print("Item not found in inventory")
end
end
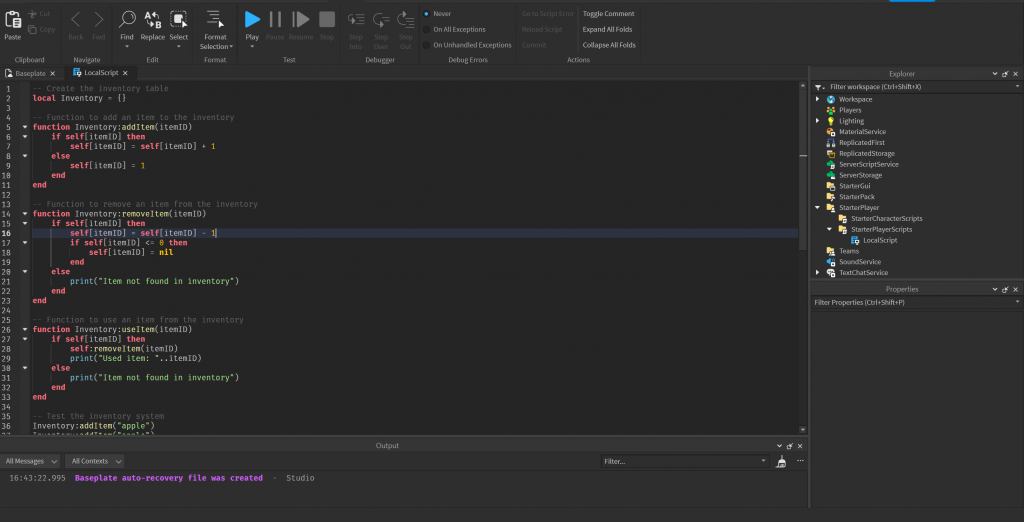
Explanation:
- This function
removeItem
is used to remove an item from the inventory. - If the item exists in the inventory, we decrement its count by 1.
- If the count becomes less than or equal to 0, we remove the item from the inventory by setting it to nil.
- If the item doesn’t exist in the inventory, it prints a message indicating that the item was not found.
-- Function to use an item from the inventory
function Inventory:useItem(itemID)
if self[itemID] then
self:removeItem(itemID)
print("Used item: "..itemID)
else
print("Item not found in inventory")
end
end
Explanation:
- This function
useItem
is used to simulate using an item from the inventory. - If the item exists in the inventory, we call the
removeItem
function to remove it and print a message indicating that the item was used. - If the item doesn’t exist in the inventory, it prints a message indicating that the item was not found.
-- Test the inventory system
Inventory:addItem("apple")
Inventory:addItem("apple")
Inventory:useItem("apple")
Inventory:removeItem("apple")
Explanation:
- Finally, we test the inventory system by adding two “apple” items, using one of them, and then removing one “apple” from the inventory.

This script provides a basic framework for managing an inventory system in Roblox Studio, allowing you to add, remove, and use items efficiently. You can expand upon this script by adding more functionality as needed for your game or application.
Full script:
-- Create the inventory table
local Inventory = {}
-- Function to add an item to the inventory
function Inventory:addItem(itemID)
if self[itemID] then
self[itemID] = self[itemID] + 1
else
self[itemID] = 1
end
end
-- Function to remove an item from the inventory
function Inventory:removeItem(itemID)
if self[itemID] then
self[itemID] = self[itemID] - 1
if self[itemID] <= 0 then
self[itemID] = nil
end
else
print("Item not found in inventory")
end
end
-- Function to use an item from the inventory
function Inventory:useItem(itemID)
if self[itemID] then
self:removeItem(itemID)
print("Used item: "..itemID)
else
print("Item not found in inventory")
end
end
-- Test the inventory system
Inventory:addItem("apple")
Inventory:addItem("apple")
Inventory:useItem("apple")
Inventory:removeItem("apple")
Conclusion
Provided script in Roblox Studio demonstrates the utilization of Lua tables to create an inventory system. While Lua does not have a built-in array data type, tables serve as a versatile substitute for arrays, allowing for the storage and manipulation of ordered collections of elements.
The script defines functions for adding, removing, and using items within the inventory. These functions leverage the flexibility of Lua tables to manage item counts efficiently. Additionally, the script includes test cases to validate the functionality of the inventory system.
By utilizing Lua tables effectively, developers can implement various data structures, including arrays, lists, and dictionaries, within Roblox Studio. This script serves as a foundational example for developing inventory systems and showcases the power and flexibility of Lua scripting in Roblox game development. Developers can extend this script to create more complex inventory systems or integrate it into their games to enhance gameplay experiences.